JavaScript Cheat Sheets
Hey everyone! I've got some great news for all you beginners learning JavaScript! A quick reference guide for JavaScript is now available! You can easily check important concepts, functions, and methods right away. For more details about the guide, check it out here.
What's in the Quick Reference Guide
Console
// => Hello world! console.log("Hello world!"); // => Hello CheatSheets.zip console.warn("hello %s", "CheatSheets.zip"); // Output error message to standard error console.error(new Error("Oops!"));
Numbers
let amount = 6; let price = 4.99;
Variables
let x = null; let name = "Tammy"; const found = false; // => Tammy, false, null console.log(name, found, x); var a; console.log(a); // => undefined
Strings
let single = "Wheres my bandit hat?"; let double = "Wheres my bandit hat?"; // => 21 console.log(single.length);
Arithmetic Operators
5 + 5 = 10 // Addition 10 - 5 = 5 // Subtraction 5 * 10 = 50 // Multiplication 10 / 5 = 2 // Division 10 % 5 = 0 // Modulus
Comments
// This is a comment /* You need to change this setting before deployment. */
Assignment Operators
let number = 100; // Both statements add 10 number = number + 10; number += 10; console.log(number); // => 120
String Interpolation
let age = 7; // String concatenation "Tommy is " + age + " years old."; // String interpolation `Tommy is ${age} years old.`;
Conditional Statements
const isMailSent = true; if (isMailSent) { console.log("Mail sent to recipient"); }
Ternary Operator
var x = 1; // => true result = x == 1 ? true : false;
Logical Operators
true || false; // true 10 > 5 || 10 > 20; // true false || false; // false 10 > 100 || 10 > 20; // false
Comparison Operators
1 > 3; // false 3 > 1; // true 250 >= 250; // true 1 === 1; // true 1 === 2; // false 1 === "1"; // false
Arrays
const fruits = ["apple", "orange", "banana"]; // Different data types const data = [1, "chicken", false];
Objects
const apple = { color: "Green", price: { bulk: "$3/kg", smallQty: "$4/kg" }, }; console.log(apple.color); // => Green console.log(apple.price.bulk); // => $3/kg
Classes
class Dog { constructor(name) { this._name = name; } introduce() { console.log("This is " + this._name + " !"); } // Static method static bark() { console.log("Woof!"); } } const myDog = new Dog("Buster"); myDog.introduce(); // Calling static method Dog.bark();
Modules
// myMath.js // Default export export default function add(x, y) { return x + y; } // Named export export function subtract(x, y) { return x - y; } // Multiple exports function multiply(x, y) { return x * y; } function duplicate(x) { return x * 2; } export { multiply, duplicate };
Asynchronous Processing
function helloWorld() { return new Promise((resolve) => { setTimeout(() => { resolve("Hello World!"); }, 2000); }); } async function msg() { const msg = await helloWorld(); console.log("Message:", msg); } msg(); // Message: Hello World! <-- after 2 seconds
This quick reference guide covers a wide range of topics from the basics to more advanced JavaScript concepts. Be sure to download it here and make it a handy tool for your coding journey!
The above is the detailed content of JavaScript Cheat Sheets. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










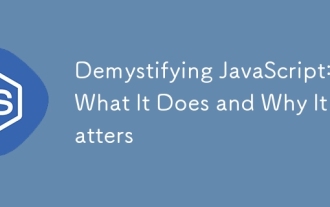
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
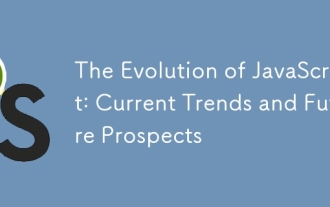
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
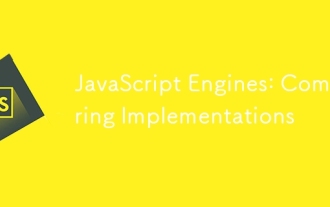
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
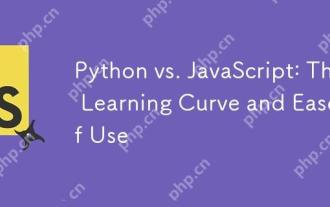
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
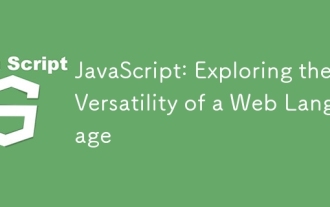
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
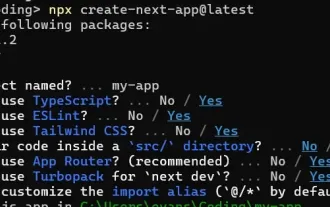
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
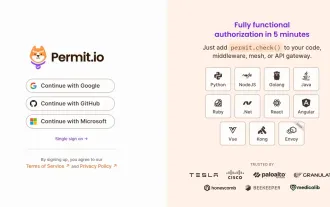
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
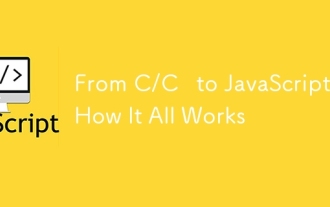
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
