Building an Advice Generator Website
Introduction
Hello, fellow developers! Today, I am excited to share a fun and simple project that I recently worked on: an Advice Generator website. This project fetches random pieces of advice from an external API and displays them on a webpage. It's a great way to practice working with APIs and building interactive web applications.
Project Overview
The Advice Generator website is a straightforward application that allows users to get random advice at the click of a button. It uses the Advice Slip API to fetch advice and display it on the webpage.
Features
- Fetches Advice: Retrieves random advice from the Advice Slip API.
- Displays Advice: Shows the advice along with an advice number.
- Interactive Button: Users can fetch new advice by clicking a button.
Technologies Used
- HTML: For the structure of the webpage.
- CSS: For styling the webpage.
- JavaScript: For fetching data from the API and updating the DOM.
Project Structure
Here's a quick look at the project structure:
Advice-Generator/ ├── index.html ├── style.css └── script.js
Installation
To get started with the project, follow these steps:
-
Clone the repository:
git clone https://github.com/abhishekgurjar-in/Advice-Generator.git
Copy after login -
Open the project directory:
cd Advice-Generator
Copy after login -
Run the project:
- You can either run it on a local server or simply open the index.html file in a web browser.
Usage
- Open the website in a web browser.
- Click the "Get Advice" button to fetch a new piece of advice.
- Enjoy the wisdom!
Code Explanation
HTML
The HTML file contains the basic structure of the webpage, including a button to fetch advice and a section to display the advice.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Advice Generator</title> <link rel="stylesheet" href="style.css"> </head> <body> <div class="container"> <h1>Advice Generator</h1> <p id="advice">Click the button to get a piece of advice!</p> <button id="adviceBtn">Get Advice</button> </div> <script src="script.js"></script> </body> </html>
CSS
The CSS file styles the webpage to make it visually appealing.
body { font-family: Arial, sans-serif; display: flex; justify-content: center; align-items: center; height: 100vh; background-color: #f0f0f0; } .container { text-align: center; background: #fff; padding: 20px; border-radius: 10px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } button { padding: 10px 20px; font-size: 16px; cursor: pointer; background-color: #007BFF; color: #fff; border: none; border-radius: 5px; margin-top: 20px; } button:hover { background-color: #0056b3; }
JavaScript
The JavaScript file fetches advice from the API and updates the DOM.
document.getElementById('adviceBtn').addEventListener('click', fetchAdvice); function fetchAdvice() { fetch('https://api.adviceslip.com/advice') .then(response => response.json()) .then(data => { document.getElementById('advice').innerText = `Advice #${data.slip.id}: ${data.slip.advice}`; }) .catch(error => { console.error('Error fetching advice:', error); }); }
Live Demo
You can check out the live demo of the Advice Generator website here.
Conclusion
Building the Advice Generator website was a fun and educational experience. It helped me practice working with APIs and building interactive web applications. I hope you find this project as enjoyable and informative as I did. Feel free to clone the repository and play around with the code. Happy coding!
Credits
- This project uses the Advice Slip API.
Author
-
Abhishek Gurjar
- GitHub Profile
The above is the detailed content of Building an Advice Generator Website. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










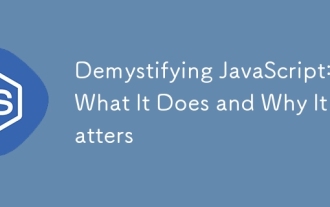
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
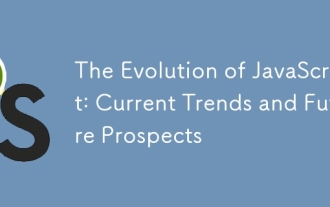
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
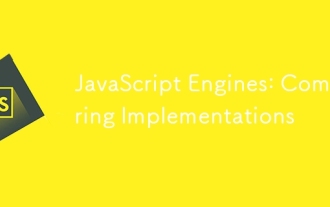
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
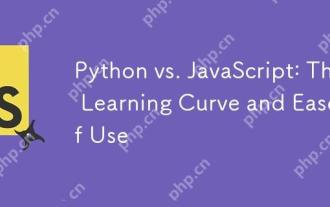
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
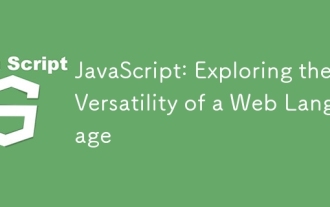
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
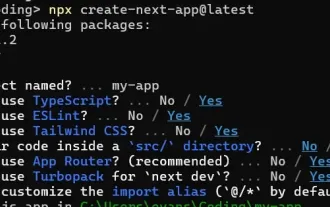
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
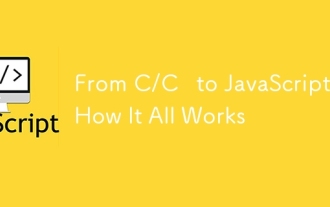
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
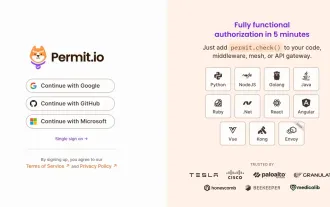
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
