Lambda functions in Python clearly explained!!
In this post we will explore Lambda functions in Python:
- What exactly is lambda functions?
- Why Do We Need Lambda Functions?
- When to Use Lambda Functions?
- Best Practices
- Examples
What exactly is lambda functions?
In Python, a lambda function is a small, anonymous function that can take any number of arguments, but can only have one expression. It's a shorthand way to create a function without declaring it with the def keyword.
Still confused?
Let's understand in laymen's terms
A lambda function is a small, shortcut way to create a simple function. Think of it like a recipe:
Normal Function (Recipe)
- Write down a list of steps (function name, ingredients, instructions)
- Follow the steps to make the dish (call the function)
Lambda Function (Quick Recipe)
- Write down just the essential steps (ingredients, instructions)
- Use it to make the dish quickly (call the lambda function)
In programming, a lambda function is a concise way to:
- Take some input (ingredients)
- Do a simple task (instructions)
- Return the result (dish)
It's like a quick, disposable recipe that you can use once or multiple times, without having to write down the full recipe book!
Syntax of Lambda Function
Where arguments is a comma-separated list of variables that will be passed to the function, and expression is the code that will be executed when the function is called.
Let's create a lambda function that takes one argument, x, and returns its square:
In this example, x is the argument, and x ** 2 is the expression that will be executed when the function is called. We can call this function like this:
print(square(5)) # Output: 25
Example: Lambda Function with Multiple Arguments
Let's create a lambda function that takes two arguments, x and y, and returns their sum:
In this example, x and y are the arguments, and x + y is the expression that will be executed when the function is called. We can call this function like this:
print(add(3, 4)) # Output: 7
Lambda functions are often used with the map(), filter(), and reduce() functions to perform operations on lists and other iterables.
Example: Using Lambda with Map
Let's use a lambda function with map() to square all numbers in a list:
In this example, the lambda function lambda x: x ** 2 is applied to each element in the numbers list using map().
Why Do We Need Lambda Functions?
Lambda functions are useful when we need to:
- Create small, one-time use functions
- Simplify code and reduce verbosity
- Use functions as arguments to higher-order functions (like map(), filter(), and reduce())
- Create anonymous functions (functions without a declared name)
When to Use Lambda Functions
Use lambda functions when:
- You need a quick, one-time use function that doesn't warrant a full function declaration
- You want to simplify code and reduce verbosity
- You need to pass a function as an argument to another function (like map(), filter(), and reduce())
- You want to create an anonymous function
Example Scenarios
- Data processing: Use lambda functions to perform simple data transformations or filtering
- Event handling: Use lambda functions as event handlers for GUI applications or web frameworks
- Functional programming: Use lambda functions to create higher-order functions and functional pipelines
Best Practices
- Keep lambda functions short and simple
- Use lambda functions for one-time use cases
- Avoid using lambda functions for complex logic or multiple statements
- Use named functions for complex logic or reusable code
By understanding lambda functions and their use cases, you can write more concise, readable, and efficient Python code.
The above is the detailed content of Lambda functions in Python clearly explained!!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










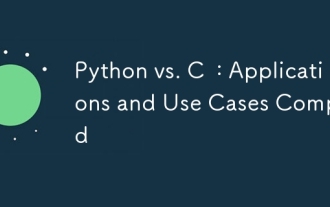
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
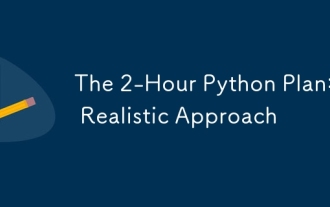
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
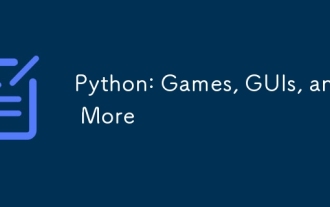
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
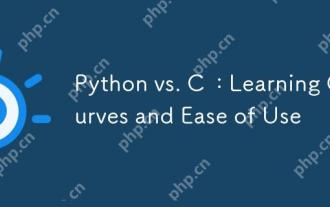
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
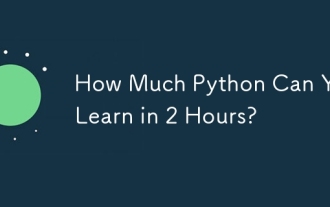
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
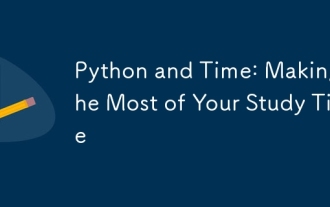
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
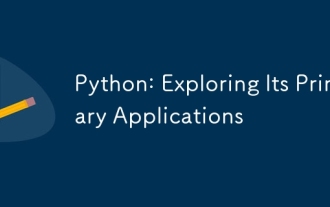
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
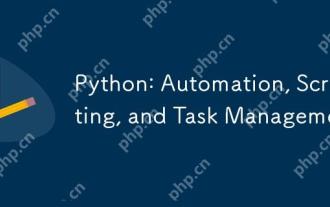
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
