Item Give preference to functions without side effects in streams
Introduction to using streams:
- New users may find it difficult to express calculations in stream pipelines.
- Streams are based on functional programming, offering expressiveness, speed and parallelization.
Structuring of the calculation:
- Structure calculations as sequences of transformations using pure functions.
- Pure functions depend only on their inputs and do not change state.
Side effects:
- Avoid side effects in functions passed to stream operations.
- Improper use of forEach that changes external state is a "bad smell".
Example 1: Code with side effects
Map<String, Long> freq = new HashMap<>(); try (Stream<String> words = new Scanner(file).tokens()) { words.forEach(word -> { freq.merge(word.toLowerCase(), 1L, Long::sum); }); }
Problem: This code uses forEach to modify the external state (freq). It is iterative and does not take advantage of streams.
Example 2: Code without side effects
Map<String, Long> freq; try (Stream<String> words = new Scanner(file).tokens()) { freq = words.collect(Collectors.groupingBy(String::toLowerCase, Collectors.counting())); }
Solution: Uses the Collectors.groupingBy collector to create the frequency table without changing the external state. Shorter, clearer and more efficient.
Appropriation of the streams API:
- Code that imitates iterative loops does not take advantage of streams.
- Use collectors for more efficient and readable operations.
Collectors:
- Simplify collecting results into collections such as lists and sets.
- Collectors.toList(), Collectors.toSet(), Collectors.toCollection(collectionFactory).
Example 3: Extracting a list of the ten most frequent words
List<String> topTen = freq.entrySet().stream() .sorted(Map.Entry.<String, Long>comparingByValue().reversed()) .limit(10) .map(Map.Entry::getKey) .collect(Collectors.toList());
Explanation:
- Orders the frequency map entries in descending order of value.
- Limits the stream to 10 words.
- Collects the most frequent words in a list.
Complexity of the Collectors API:
- API has 39 methods, but many are for advanced use.
- Collectors can be used to create maps (toMap, groupingBy).
Maps and collection strategies:
- toMap(keyMapper, valueMapper) for unique key-values.
- Strategies for dealing with key conflicts using the merge function.
- groupingBy to group elements into categories based on classifier functions.
Example 4: Using toMap with merge function
Map<String, Long> freq; try (Stream<String> words = new Scanner(file).tokens()) { freq = words.collect(Collectors.toMap( String::toLowerCase, word -> 1L, Long::sum )); }
Explanation:
- toMap maps words to their frequencies.
- Merge function (Long::sum) deals with key conflicts by summing the frequencies.
Example 5: Grouping albums by artist and finding the best-selling album
Map<Artist, Album> topAlbums = albums.stream() .collect(Collectors.toMap( Album::getArtist, Function.identity(), BinaryOperator.maxBy(Comparator.comparing(Album::sales)) ));
Explanation:
- toMap maps artists to their best-selling albums.
- BinaryOperator.maxBy determines the best-selling album for each artist.
String Collection:
Collectors.joining to concatenate strings with optional delimiters.
Example 6: Concatenating strings with delimiter
String result = Stream.of("came", "saw", "conquered") .collect(Collectors.joining(", ", "[", "]"));
Explanation:
- Collectors.joining concatenates strings with a comma as delimiter, prefix and suffix.
- Result: [came, saw, conquered].
Conclusion:
- Essence of streams is in functions without side effects.
- forEach should only be used to report results.
- Knowledge about collectors is essential for effective use of streams.
The above is the detailed content of Item Give preference to functions without side effects in streams. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










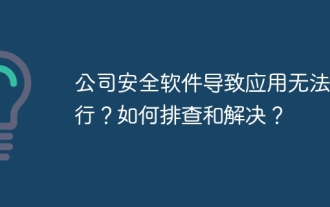
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
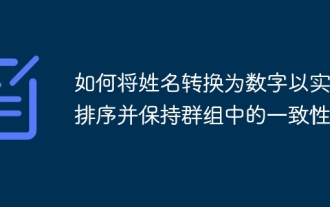
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
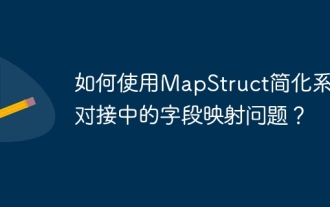
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
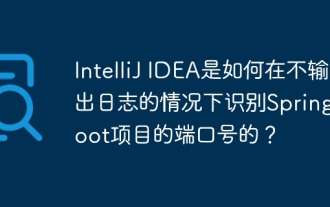
Start Spring using IntelliJIDEAUltimate version...
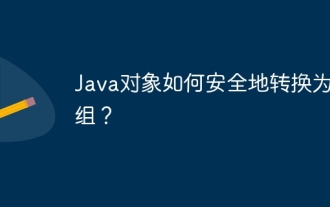
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
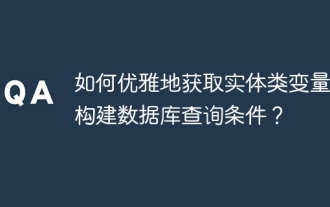
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
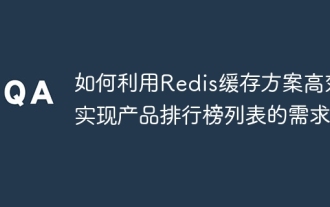
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
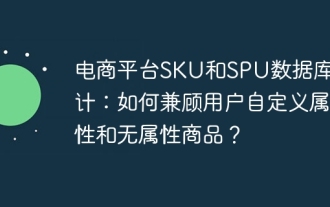
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
