What Does It Mean to Minify CSS, Javascript? Why, and When Do It?
Optimizing web performance is crucial for delivering a fast and seamless user experience. One effective way to achieve this is through minification and combining of CSS, JavaScript, and HTML files. Today, we will explore what minification and combining mean, why they are important, and how they can be implemented with practical examples
Minification
Minification is the process of removing unnecessary characters from code without changing its functionality. This includes:
- Removing whitespace: Spaces, tabs, and line breaks.
- Removing comments: Any non-functional text meant for developers.
- Shortening variable names: Using shorter names for variables and functions.
Minification Example
Original Code
CSS File (styles.css)
/* Main Styles */ body { background-color: #f0f0f0; /* Light gray background */ font-family: Arial, sans-serif; } /* Header Styles */ header { background-color: #333; /* Dark background for header */ color: #fff; padding: 10px; } header h1 { margin: 0; }
JavaScript File (script.js)
// Function to change background color function changeBackgroundColor(color) { document.body.style.backgroundColor = color; } // Function to log message function logMessage(message) { console.log(message); }
Minified Code
Minified CSS (styles.min.css)
cssbody{background-color:#f0f0f0;font-family:Arial,sans-serif}header{background-color:#333;color:#fff;padding:10px}header h1{margin:0}
Minified JavaScript (script.min.js)
javascript function changeBackgroundColor(a){document.body.style.backgroundColor=a}function logMessage(a){console.log(a)}
Explanation:
- CSS: Whitespace and comments are removed. The property names and values are shortened where possible.
- JavaScript: Comments and unnecessary whitespace are removed. Variable names are shortened.
Why Do It:
- Reduce File Size: Smaller files mean less data to download, which improves load times.
- Improve Performance: Faster file transfers result in quicker page load times and better user experience.
- Decrease Bandwidth Usage: Smaller files reduce the amount of data transferred, saving bandwidth and potentially lowering costs.
When To Do It:
- Before Deployment: Minify files as part of your build process before deploying to production. This ensures that the code served to users is optimized for performance.
- On Every Release: Incorporate minification into your continuous integration/continuous deployment (CI/CD) pipeline to automatically minify files with every release.
Combining Files
Combining files refers to merging multiple CSS or JavaScript files into a single file. For example:
- Combining CSS Files: Instead of having multiple CSS files, you combine them into one.
- Combining JavaScript Files: Similarly, multiple JavaScript files are combined into one.
Combining Files Example
Original Files
CSS Files
- reset.css
- typography.css
- layout.css
JavaScript Files
- utils.js
- main.js
- analytics.js
Combined Files
Combined CSS (styles.css)
css/* Reset styles */ body, h1, h2, h3, p { margin: 0; padding: 0; } /* Typography styles */ body { font-family: Arial, sans-serif; } h1 { font-size: 2em; } /* Layout styles */ .container { width: 100%; max-width: 1200px; margin: 0 auto; }
Combined JavaScript (scripts.js)
javascript// Utility functions function changeBackgroundColor(color) { document.body.style.backgroundColor = color; } function logMessage(message) { console.log(message); } // Main application logic function initApp() { console.log('App initialized'); } window.onload = initApp; // Analytics function trackEvent(event) { console.log('Event tracked:', event); }
Explanation:
- CSS: Multiple CSS files are merged into a single file, preserving their order and combining styles.
- JavaScript: Multiple JavaScript files are merged into a single file, keeping functions and logic organized.
Why Do It:
- Reduce HTTP Requests: Each file requires a separate HTTP request. Combining files reduces the number of requests the browser needs to make, which can significantly improve load times.
- Improve Page Load Speed: Fewer HTTP requests mean less overhead and faster loading, as browsers can handle fewer connections and process fewer files.
- Simplify Management: Fewer files can simplify your file structure and make it easier to manage dependencies.
When To Do It:
- During the Build Process: Like minification, combining files should be part of your build process, usually handled by task runners or build tools (e.g., Webpack, Gulp, or Parcel).
- In Production: Combine files before deploying to production to ensure that users receive the optimized versions.
Tools and Techniques
- Minification Tools: Tools like UglifyJS, Terser (for JavaScript), and CSSNano (for CSS) are commonly used for minification.
- Build Tools: Task runners like Gulp or Webpack can automate both minification and file combining.
- CDNs: Many Content Delivery Networks (CDNs) offer built-in minification and combination features.
By minifying and combinSure! Let's walk through some practical examples of minifying and combining CSS and JavaScript files.
Why This Matters
- Minification: Reduces the size of individual files, which decreases the amount of data the browser needs to download.
- Combining: Reduces the number of HTTP requests, which decreases load time and improves performance.
Tools for Combining and Minifying:
- Gulp: A task runner that can automate minification and combining.
- Webpack: A module bundler that can combine and minify files as part of its build process.
- Online Tools: Websites like CSS Minifier and JSCompress can also be used for minification.
By following these practices, you optimize the performance of your web application, leading to a faster and smoother user experience.ing CSS and JavaScript files, you streamline the delivery of your web assets, leading to faster load times and a better overall user experience.
The above is the detailed content of What Does It Mean to Minify CSS, Javascript? Why, and When Do It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










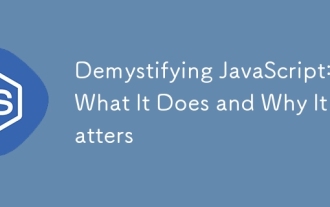
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
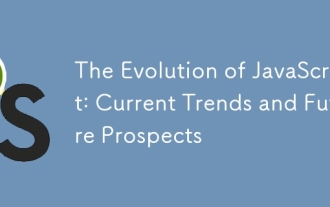
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
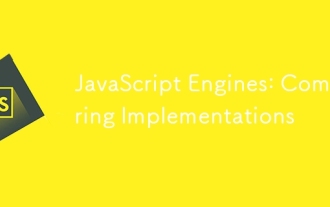
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
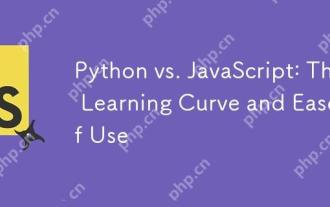
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
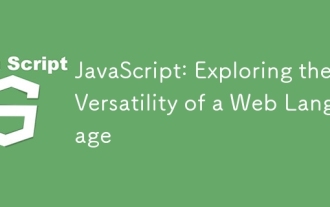
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
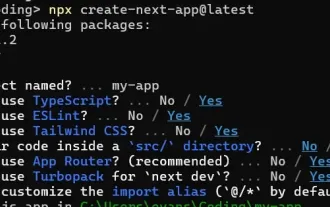
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
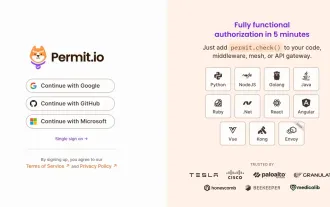
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
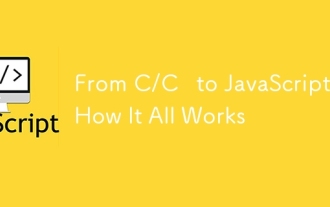
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
