Java Ecosystem Overview
Table of Contents
- Introduction
-
JVM (Java Virtual Machine)
-
Architecture of the JVM
- Class Loader
-
JVM Memory
- Method Area
- Heap
- Stack Area
- Program Counter (PC) Register
- Native Method Stack
-
Execution Engine
- Interpreter
- Just-In-Time (JIT) Compiler
- Garbage Collector
-
Architecture of the JVM
-
JRE (Java Runtime Environment)
-
Key Components of the JRE
- Execution Tasks
- Class Libraries
- Java Native Interface (JNI)
- Security Manager
-
Key Components of the JRE
-
JDK (Java Development Kit)
-
Core Features of the JDK
- javac (Java Compiler)
- java (Java Application Launcher)
- jdb (Java Debugger)
- jar (Java Archive Tool)
- javadoc (Java Documentation Generator)
-
Core Features of the JDK
- JVM vs JRE vs JDK: What's the Difference?
- JDK, JRE, JVM Hierarchy
Introduction
The Java ecosystem is the broad set of tools, technologies, libraries, and frameworks that surround and support the Java programming language. It encompasses everything needed to develop, deploy, and manage Java applications. It revolves around JDK, JRE, JVM
JVM (Java Development Kit)
The JVM acts like a translator that allows your computer to run Java programs and other languages compiled into Java bytecode. It translates the code into something your computer's hardware can understand and execute.
Architecture of the JVM
Class Loader
Loading load
Load .class files into memory. Locates, loads, and links class files (Java bytecode) for execution.-
Linking
- Verification: Verifies the bytecode.
- Preparation: Allocates memory for static variables and initializes the memory to default values.
- Resolution: Resolves symbolic references to direct references.
Initialization
Initialization is the final step where the JVM prepares a class or interface for use. This step happens after the class has been loaded (into memory) and linked.
JVM Memory
-
Method Area
Method area Stores class-level data such as methods and variables, the runtime constant pool, and code for methods.
public class Person { private String name; public void setName(String name) { this.name = name; } }
Copy after loginCopy after loginWhen you define a class Person, the Method Area stores the structure of the Person class, including its methods (setName) and fields (name), and the runtime constant pool which contains references like method names and constant values.
-
Heap
Heap is where the runtime memory objects are allocated. The heap is shared among all threads and is where the garbage collection process occurs.
Person p = new Person();
Copy after loginCopy after loginWhen you create a new Person object, it is allocated on the Heap.
-
Stack Area
Stack area stores frames, which contain local variables, operand stacks, and references to the runtime constant pool of the class being executed. Each thread has its own stack.
public void someMethod() { int a = 10; int b = 20; int sum = a + b; }
Copy after loginCopy after loginEach time someMethod is called, a new frame is pushed onto the Stack Area. This frame includes local variables (a, b, and sum), an operand stack for intermediate calculations, and a reference to the method’s class in the Runtime Constant Pool.
Program Counter (PC) Register
PC contains the address of the current JVM instruction being executed. Each thread has its own PC register.Native Method Stack
Similar to the Java stack, but used for native methods.
Execution Engine
Interpreter
Interpreter reads Java bytecode and executes it line by line, converting each bytecode instruction into a sequence of machine-level instructions that can be executed by the CPU.Just-In-Time (JIT) Compiler
Converts bytecode into native machine code at runtime to improve performance.Garbage Collector
Garbage collector is responsible for automatically managing memory in the JVM. It identifies and deallocates memory that is no longer in use, freeing it up for new objects.
JRE
JRE is a software package that provides the necessary environment to run Java applications. It is designed to execute Java bytecode on a machine, making it an essential part of the "write once, run anywhere" (WORA) principle of Java.
public class Person {
private String name;
public void setName(String name) {
this.name = name;
}
}
Copy after loginCopy after loginPerson p = new Person();
Copy after loginCopy after login
public void someMethod() {
int a = 10;
int b = 20;
int sum = a + b;
}
Copy after loginCopy after loginJDK (Java Development Kit)
│
├── JRE (Java Runtime Environment)
│ │
│ ├── JVM (Java Virtual Machine)
│ │ ├── Class Loader
│ │ ├── Bytecode Verifier
│ │ ├── Execution Engine
│ │ │ ├── Interpreter
│ │ │ ├── Just-In-Time (JIT) Compiler
│ │ │ └── Garbage Collector
│ │ └── Runtime Libraries (core libraries like java.lang, java.util, etc.)
│ │
│ └── Java APIs (Core libraries and additional libraries)
│
├── Development Tools (like javac, jdb, jar, javadoc, etc.)
└── Documentation (API docs, guides)
Copy after loginCopy after login
<🎝><🎝>Key<🎝>Components<🎝>of<🎝>the<🎝>JRE
public class Person { private String name; public void setName(String name) { this.name = name; } }
Person p = new Person();
public void someMethod() { int a = 10; int b = 20; int sum = a + b; }
JDK (Java Development Kit) │ ├── JRE (Java Runtime Environment) │ │ │ ├── JVM (Java Virtual Machine) │ │ ├── Class Loader │ │ ├── Bytecode Verifier │ │ ├── Execution Engine │ │ │ ├── Interpreter │ │ │ ├── Just-In-Time (JIT) Compiler │ │ │ └── Garbage Collector │ │ └── Runtime Libraries (core libraries like java.lang, java.util, etc.) │ │ │ └── Java APIs (Core libraries and additional libraries) │ ├── Development Tools (like javac, jdb, jar, javadoc, etc.) └── Documentation (API docs, guides)
Execution<🎝>Tasks
The<🎝>JRE<🎝>facilitates<🎝>the<🎝>execution<🎝>of<🎝>Java<🎝>applications<🎝>by<🎝>providing<🎝>the<🎝>JVM<🎝>and<🎝>the<🎝>necessary<🎝>libraries<🎝>and<🎝>resources.<🎝>JRE<🎝>ensures<🎝>that<🎝>the<🎝>JVM<🎝>has<🎝>everything<🎝>it<🎝>needs<🎝>to<🎝>perform<🎝>these<🎝>tasks<🎝>on<🎝>any<🎝>platform.<🎝>Think<🎝>of<🎝>the<🎝>JRE<🎝>as<🎝>the<🎝>complete<🎝>package<🎝>that<🎝>includes<🎝>the<🎝>JVM,<🎝>which<🎝>does<🎝>the<🎝>heavy<🎝>lifting,<🎝>and<🎝>other<🎝>components<🎝>that<🎝>support<🎝>the<🎝>execution<🎝>of<🎝>Java<🎝>applications.Class<🎝>Libraries
JRE<🎝>includes<🎝>a<🎝>set<🎝>of<🎝>standard<🎝>Java<🎝>class<🎝>libraries,<🎝>which<🎝>provide<🎝>reusable<🎝>code<🎝>for<🎝>performing<🎝>common<🎝>tasks,<🎝>like<🎝>data<🎝>structures,<🎝>I/O,<🎝>networking,<🎝>concurrency,<🎝>and<🎝>more.Java<🎝>Native<🎝>Interface<🎝>(JNI)
JNI<🎝>allows<🎝>Java<🎝>applications<🎝>to<🎝>interact<🎝>with<🎝>native<🎝>code<🎝>written<🎝>in<🎝>languages<🎝>like<🎝>C<🎝>or<🎝>C++.<🎝>This<🎝>feature<🎝>is<🎝>essential<🎝>for<🎝>integrating<🎝>platform-specific<🎝>features<🎝>or<🎝>using<🎝>existing<🎝>native<🎝>libraries.Security<🎝>Manager<🎝>
The<🎝>Security<🎝>Manager<🎝>enforces<🎝>security<🎝>policies<🎝>for<🎝>Java<🎝>applications,<🎝>restricting<🎝>actions<🎝>such<🎝>as<🎝>file<🎝>access,<🎝>network<🎝>connections,<🎝>and<🎝>the<🎝>execution<🎝>of<🎝>potentially<🎝>unsafe<🎝>code.
<🎝><🎝> <🎝><🎝> <🎝><🎝>JDK<🎝>(Java<🎝>Development<🎝>Kit)
JDK<🎝>is<🎝>a<🎝>tools<🎝>that<🎝>enables<🎝>developers<🎝>to<🎝>write,<🎝>compile,<🎝>debug,<🎝>and<🎝>run<🎝>Java<🎝>applications.<🎝>It<🎝>is<🎝>a<🎝>superset<🎝>of<🎝>JRE<🎝>and<🎝>includes<🎝>additional<🎝>tools<🎝>for<🎝>Java<🎝>development.
<🎝><🎝> <🎝><🎝> <🎝><🎝>Core<🎝>Features<🎝>of<🎝>the<🎝>JDK
javac<🎝>(Java<🎝>Compiler)
javac<🎝>is<🎝>use<🎝>to<🎝>for<🎝>converting<🎝>Java<🎝>source<🎝>code<🎝>(.java<🎝>files)<🎝>into<🎝>bytecode<🎝>(.class<🎝>files).<🎝>This<🎝>bytecode<🎝>is<🎝>then<🎝>executed<🎝>by<🎝>the<🎝>Java<🎝>Virtual<🎝>Machine<🎝>(JVM).java<🎝>(Java<🎝>Application<🎝>Launcher)
java<🎝>command<🎝>launches<🎝>a<🎝>Java<🎝>application.<🎝>It<🎝>loads<🎝>the<🎝>necessary<🎝>class<🎝>files,<🎝>interprets<🎝>the<🎝>bytecode,<🎝>and<🎝>starts<🎝>the<🎝>application.jdb<🎝>(Java<🎝>Debugger)
jdb<🎝>is<🎝>the<🎝>command-line<🎝>debugger<🎝>for<🎝>Java<🎝>programs.<🎝>It<🎝>allows<🎝>you<🎝>to<🎝>inspect<🎝>and<🎝>debug<🎝>Java<🎝>applications<🎝>at<🎝>runtime.jar<🎝>(Java<🎝>Archive<🎝>Tool)
jar<🎝>tool<🎝>packages<🎝>multiple<🎝>files<🎝>into<🎝>a<🎝>single<🎝>archive<🎝>file,<🎝>typically<🎝>with<🎝>a<🎝>.jar<🎝>extension.<🎝>These<🎝>JAR<🎝>files<🎝>are<🎝>used<🎝>to<🎝>distribute<🎝>Java<🎝>applications<🎝>and<🎝>libraries.javadoc<🎝>(Java<🎝>Documentation<🎝>Generator)
javadoc<🎝>generates<🎝>HTML<🎝>documentation<🎝>from<🎝>Java<🎝>source<🎝>code,<🎝>using<🎝>the<🎝>special<🎝>/**<🎝>*/<🎝>comments<🎝>known<🎝>as<🎝>doc<🎝>comments.
JVM vs JVE vs JDK, what's the difference?
Feature/Aspect | JVM | JRE | JDK |
---|---|---|---|
Purpose | Executes Java bytecode | Provides the environment to run Java applications | Provides tools to develop, compile, debug, and run Java applications |
Includes | JVM itself, which includes class loader, bytecode verifier, and execution engine | JVM + Core libraries (like java.lang, java.util, etc.), and other runtime components | JRE + Development tools (like javac, jdb, jar, etc.), documentation |
Components | - Class Loader - Bytecode Verifier - Execution Engine (Interpreter, JIT) |
- JVM - Core Java libraries - Java Plug-in - Java Web Start |
- JRE - Java Compiler (javac) - JAR Tool (jar) - Debugger (jdb) - Documentation Generator (javadoc) - Other development tools |
Main Functionality | Executes Java bytecode, enabling platform independence | Provides the minimum requirements to run Java applications | Allows developers to write, compile, and debug Java code |
Who Uses It? | End-users running Java applications | End-users running Java applications | Java developers writing and compiling Java applications |
Installation Size | Smallest | Larger than JVM but smaller than JDK | Largest (includes JRE and development tools) |
Developer Tools | No | No | Yes (includes compiler, debugger, profiler, etc.) |
Required to Run Java Programs | Yes | Yes | No (but needed to create Java programs) |
Platform Independence | Provides platform independence by abstracting the underlying hardware | Yes, because it includes the JVM | Yes, it includes everything from JRE |
Examples of Usage | - Running any Java application (e.g., desktop applications, servers) | - Running Java applications in production or end-user environments | - Writing and compiling Java code - Packaging applications - Debugging |
Availability | Part of JRE and JDK | Standalone or part of JDK | Standalone package |
JDK, JRE, JVM hierarchy
JDK (Java Development Kit) │ ├── JRE (Java Runtime Environment) │ │ │ ├── JVM (Java Virtual Machine) │ │ ├── Class Loader │ │ ├── Bytecode Verifier │ │ ├── Execution Engine │ │ │ ├── Interpreter │ │ │ ├── Just-In-Time (JIT) Compiler │ │ │ └── Garbage Collector │ │ └── Runtime Libraries (core libraries like java.lang, java.util, etc.) │ │ │ └── Java APIs (Core libraries and additional libraries) │ ├── Development Tools (like javac, jdb, jar, javadoc, etc.) └── Documentation (API docs, guides)
The above is the detailed content of Java Ecosystem Overview. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










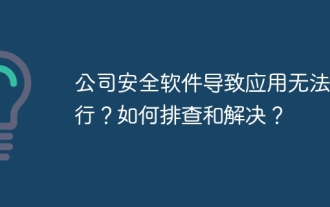
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
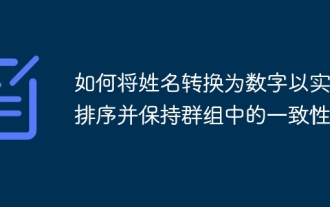
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
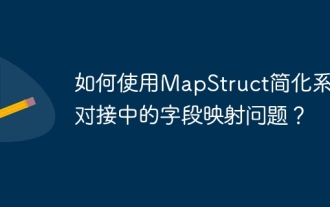
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
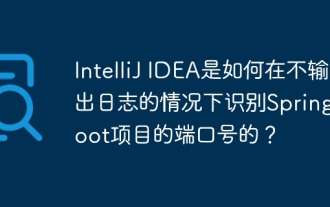
Start Spring using IntelliJIDEAUltimate version...
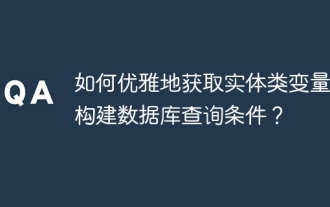
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
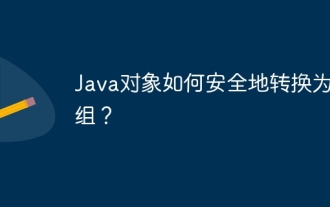
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
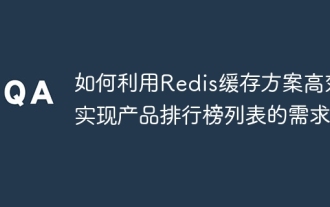
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
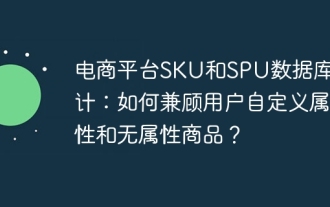
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
