Configuring AWS SDK for PHP with S3
Amazon Web Services (AWS) is a powerful platform that offers a wide range of services for developers and businesses. Among these services, Amazon Simple Storage Service (S3) is one of the most popular and widely used. To interact with S3 programmatically, you can use the AWS SDK for PHP. In this article, we will guide you through the process of configuring the AWS SDK for PHP with S3.
Prerequisites
Before we begin, make sure you have the following:
- An AWS account
- AWS Access Key ID and Secret Access Key
- PHP 5.6 or higher
- Composer installed
Installation
To install the AWS SDK for PHP, you can use Composer. Run the following command in your terminal:
composer require aws/aws-sdk-php
This command will install the latest version of the AWS SDK for PHP in your project.
Configuration
Once you have installed the SDK, you need to configure it with your AWS Access Key ID and Secret Access Key. You can do this by creating a configuration file or by setting environment variables.
Configuration File
Create a new file named config.php in your project and add the following code:
<?php require 'vendor/autoload.php'; use Aws\Sdk; $sdk = new Sdk([ 'region' => 'us-east-1', 'version' => 'latest', 'credentials' => [ 'key' => 'YOUR_ACCESS_KEY_ID', 'secret' => 'YOUR_SECRET_ACCESS_KEY', ] ]); $s3Client = $sdk->createS3();
Replace YOUR_ACCESS_KEY_ID and YOUR_SECRET_ACCESS_KEY with your actual AWS Access Key ID and Secret Access Key.
Environment Variables
Alternatively, you can set the AWS Access Key ID and Secret Access Key as environment variables:
export AWS_ACCESS_KEY_ID=YOUR_ACCESS_KEY_ID export AWS_SECRET_ACCESS_KEY=YOUR_SECRET_ACCESS_KEY
Then, create the S3 client as follows:
<?php require 'vendor/autoload.php'; use Aws\Sdk; $sdk = new Sdk([ 'region' => 'us-east-1', 'version' => 'latest', ]); $s3Client = $sdk->createS3();
Ready to learn more about AWS and PHP? Check out our other articles on AWS configure SSO and Fixing laravel permission denied errors.
Usage
Now that you have configured the AWS SDK for PHP with S3, you can start using it to interact with your S3 buckets. Here's an example of how to list all the buckets in your account:
$buckets = $s3Client->listBuckets(); foreach ($buckets['Buckets'] as $bucket) { echo $bucket['Name'] . PHP_EOL; }
Sure, here are some additional examples and best practices for using the AWS SDK for PHP with S3.
Uploading a File
To upload a file to an S3 bucket, you can use the putObject method. Here's an example:
$bucketName = 'my-bucket'; $keyName = 'my-file.txt'; $filePath = '/path/to/my-file.txt'; $result = $s3Client->putObject([ 'Bucket' => $bucketName, 'Key' => $keyName, 'SourceFile' => $filePath, ]); echo $result['ObjectURL'] . PHP_EOL;
This code will upload the file located at /path/to/my-file.txt to the my-bucket bucket and print the URL of the uploaded file.
Downloading a File
To download a file from an S3 bucket, you can use the getObject method. Here's an example:
$bucketName = 'my-bucket'; $keyName = 'my-file.txt'; $filePath = '/path/to/downloaded-file.txt'; $result = $s3Client->getObject([ 'Bucket' => $bucketName, 'Key' => $keyName, 'SaveAs' => $filePath, ]); echo $result['ContentLength'] . ' bytes downloaded.' . PHP_EOL;
This code will download the file with the key my-file.txt from the my-bucket bucket and save it to /path/to/downloaded-file.txt.
Listing Objects
To list the objects in an S3 bucket, you can use the listObjects method. Here's an example:
$bucketName = 'my-bucket'; $result = $s3Client->listObjects([ 'Bucket' => $bucketName, ]); foreach ($result['Contents'] as $object) { echo $object['Key'] . PHP_EOL; }
This code will list all the objects in the my-bucket bucket and print their keys.
Best Practices - AWS SDK + PHP + S3
Here are some best practices to keep in mind when using the AWS SDK for PHP with S3:
- Use IAM roles and policies to manage access to your S3 resources.
- Use versioning to keep multiple versions of your objects and protect against accidental deletion.
- Use lifecycle policies to automatically manage the storage and retention of your objects.
- Use transfer acceleration to improve the performance of your uploads and downloads.
- Use server-side encryption to protect your data at rest.
- Use event notifications to trigger actions based on changes to your S3 objects.
Sure, here are some additional tips for using the AWS SDK for PHP with S3 in Laravel.
Using the AWS SDK for PHP with Laravel
Laravel has built-in support for the AWS SDK for PHP, which makes it easy to use S3 in your Laravel applications. Here are some tips for using the SDK with Laravel:
- Install the AWS SDK for PHP package via Composer:
composer require aws/aws-sdk-php
- Configure your AWS credentials in your .env file:
AWS_ACCESS_KEY_ID=your_access_key_id AWS_SECRET_ACCESS_KEY=your_secret_access_key AWS_DEFAULT_REGION=your_region
- Use the Storage facade to interact with S3:
use Illuminate\Support\Facades\Storage; // Upload a file Storage::disk('s3')->put('my-file.txt', file_get_contents('/path/to/my-file.txt')); // Download a file Storage::disk('s3')->download('my-file.txt', '/path/to/downloaded-file.txt'); // List the objects in a bucket $objects = Storage::disk('s3')->listContents('my-bucket'); foreach ($objects as $object) { echo $object['path'] . PHP_EOL; }
- Use Laravel's Flysystem adapter to customize the behavior of the Storage facade:
use Illuminate\Support\ServiceProvider; use League\Flysystem\AwsS3V3\AwsS3V3Adapter; use Aws\S3\S3Client; class S3ServiceProvider extends ServiceProvider { public function register() { $this->app->singleton('filesystems.disks.s3', function ($app) { return new AwsS3V3Adapter( new S3Client([ 'region' => config('filesystems.disks.s3.region'), 'version' => 'latest', 'credentials' => [ 'key' => config('filesystems.disks.s3.key'), 'secret' => config('filesystems.disks.s3.secret'), ], ]), config('filesystems.disks.s3.bucket') ); }); } }
- Use Laravel's queue system to perform S3 operations asynchronously:
use Illuminate\Support\Facades\Storage; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Contracts\Queue\ShouldQueue; class UploadFile implements ShouldQueue { use InteractsWithQueue; protected $filePath; public function __construct($filePath) { $this->filePath = $filePath; } public function handle() { Storage::disk('s3')->put('my-file.txt', file_get_contents($this->filePath)); } }
Best Practices - AWS SDK + PHP + Laravel
Here are some best practices to keep in mind when using the AWS SDK for PHP with S3 in Laravel:
- Use Laravel's built-in support for the AWS SDK for PHP to simplify your code and reduce the amount of boilerplate code you need to write.
- Use Laravel's queue system to perform S3 operations asynchronously, which can improve the performance and scalability of your Laravel applications.
- Use Laravel's Flysystem adapter to customize the behavior of the Storage facade and to integrate S3 with other Laravel features, such as Laravel's cache system.
- Use Laravel's queue system to perform S3 operations asynchronously, which can improve the performance and scalability of your Laravel applications.
- Use Laravel's encryption features to encrypt sensitive data before storing it in S3.
- Use Laravel's logging features to log any errors or exceptions that occur when using the AWS SDK for PHP with S3.
Conclusion
In this article, we have covered the basics of configuring the AWS SDK for PHP with S3 and provided some additional examples and best practices for using the SDK with S3. We have also provided some additional tips for using the SDK with S3 in Laravel. By following these guidelines, you can ensure that your PHP applications are secure, efficient, and scalable.
Want to learn more about AWS and PHP? Check out our other articles on DevOps Mind.
The above is the detailed content of Configuring AWS SDK for PHP with S3. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










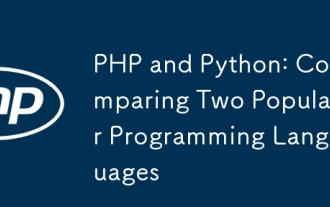
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
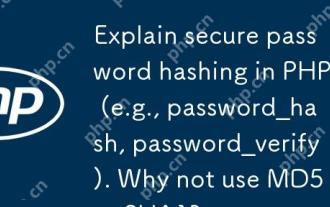
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
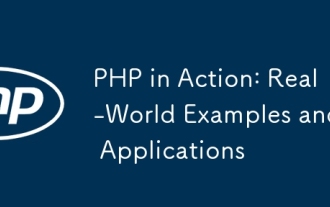
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
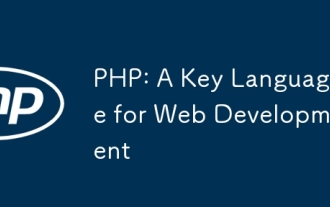
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
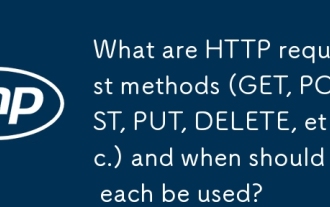
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
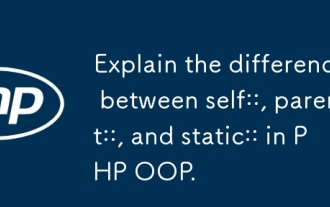
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
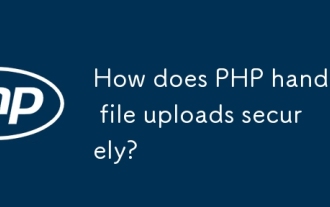
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
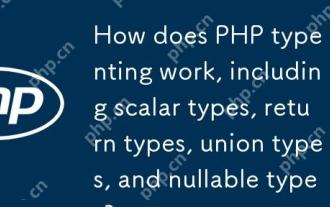
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
