


Transform Your Java Code: Unlock the Power of Immutability with Lombok in Just Minutes!
Why Immutable Classes?
Immutable classes are beneficial because they are inherently thread-safe, easy to reason about, and prevent accidental changes to the object's state. An immutable object’s state cannot be modified after it is created, making it a valuable design pattern, especially in multi-threaded environments.
Traditional Approach to Creating an Immutable Class
Consider the following Employee class:
final class Employee { private final long id; private final String name; private final double salary; public Employee(long id, String name, double salary) { this.id = id; this.name = name; this.salary = salary; } public long getId() { return id; } public String getName() { return name; } public double getSalary() { return salary; } }
In this traditional approach:
- The class is marked final to prevent subclassing.
- All fields are private and final, ensuring they cannot be changed after initialization.
- Only getter methods are provided to access the field values.
While this approach works well, it involves writing boilerplate code for constructors, getters, and sometimes equals, hashCode, and toString methods.
Using Lombok to Eliminate Boilerplate
Lombok can drastically reduce the amount of code you need to write. Here's how you can achieve the same functionality with Lombok:
import lombok.AllArgsConstructor; import lombok.Getter; @AllArgsConstructor @Getter final class Employee { private final long id; private final String name; private final double salary; }
This version uses Lombok annotations to generate the constructor and getters automatically:
- @AllArgsConstructor generates a constructor with all fields as parameters.
- @Getter generates getter methods for each field.
Simplifying Even Further with @Value
Lombok’s @Value annotation is a more powerful alternative that combines multiple features to create an immutable class:
import lombok.Value; @Value class Employee { long id; String name; double salary; }
With @Value, Lombok automatically:
- Makes the class final.
- Makes all fields private and final by default.
- Generates an all-arguments constructor.
- Generates getters for all fields.
- Implements equals, hashCode, and toString methods.
This reduces your class definition to just the fields, with all the necessary code automatically generated.
Handling Field Updates with @With
Immutable objects do not allow modification of their state. However, in certain cases, you may need to create a modified copy of the object, such as updating an employee's salary. Without Lombok, this could look like:
@Value class Employee { long id; String name; double salary; } class Main { public static void main(String... args) { var emp = new Employee(1L, "Aman", 10_000.0); emp = updateSalary(emp, 12_0000.0); } public Employee updateSalary(Employee emp, long newSalary) { return new Employee(emp.getId(), emp.getName(), newSalary); } }
This is straightforward but tedious, especially when dealing with classes that have many fields.
Lombok's @With annotation simplifies this:
import lombok.Value; import lombok.With; @Value class Employee { long id; String name; @With double salary; } class Main { public static void main(String... args) { var emp = new Employee(1L, "Aman", 10_000.0); emp = updateSalary(emp, 12_0000.0); } public Employee updateSalary(Employee emp, double newSalary) { return emp.withSalary(newSalary); } }
The @With annotation generates a method that returns a new instance of the class with the specified field updated, leaving the rest unchanged.
The Final De-lomboked Version
The de-lomboked version of our Employee class (i.e., what Lombok generates under the hood) would look like this:
final class Employee { private final long id; private final String name; private final double salary; public Employee(long id, String name, double salary) { this.id = id; this.name = name; this.salary = salary; } public Employee withSalary(double salary) { return this.salary == salary ? this : new Employee(this.id, this.name, salary); } public long getId() { return this.id; } public String getName() { return this.name; } public double getSalary() { return this.salary; } @Override public boolean equals(final Object o) { if (o == this) return true; if (!(o instanceof Employee)) return false; final Employee other = (Employee) o; if (this.getId() != other.getId()) return false; final Object this$name = this.getName(); final Object other$name = other.getName(); if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false; return Double.compare(this.getSalary(), other.getSalary()) == 0; } @Override public int hashCode() { final int PRIME = 59; int result = 1; final long $id = this.getId(); result = result * PRIME + (int) ($id >>> 32 ^ $id); final Object $name = this.getName(); result = result * PRIME + ($name == null ? 43 : $name.hashCode()); final long $salary = Double.doubleToLongBits(this.getSalary()); result = result * PRIME + (int) ($salary >>> 32 ^ $salary); return result; } @Override public String toString() { return "Employee(id=" + this.getId() + ", name=" + this.getName() + ", salary=" + this.getSalary() + ")"; } }
Common Pitfalls When Using Lombok for Immutability
While Lombok simplifies creating immutable classes, it's important to note some potential pitfalls:
- Mutable Collections: If your class contains mutable collections (like List or Map), Lombok’s @Value annotation won’t protect you from modifications to these collections. Consider using immutable versions like Collections.unmodifiableList or List.of() for initialization.
- Complex Objects: If your class fields are complex objects that themselves are mutable, the immutability of the class is compromised. Ensure that all fields are either primitive, immutable objects, or properly encapsulated to prevent mutation.
Performance Considerations
While immutability offers significant benefits, it’s important to consider the performance impact, particularly in scenarios involving frequent updates:
- Memory Usage: Creating new instances every time a change is needed can lead to higher memory usage. However, this trade-off is often worth it for the benefits of thread safety and reduced complexity.
- Garbage Collection: The creation of multiple short-lived objects can put additional pressure on the garbage collector. Ensure that your application’s memory management is optimized for such scenarios.
Conclusion
Lombok's @Value and @With annotations offer a powerful and concise way to create immutable classes in Java, eliminating the need for boilerplate code and making your code more readable and maintainable. By leveraging these annotations, you can focus on the logic of your application rather than the mechanics of class design.
The above is the detailed content of Transform Your Java Code: Unlock the Power of Immutability with Lombok in Just Minutes!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










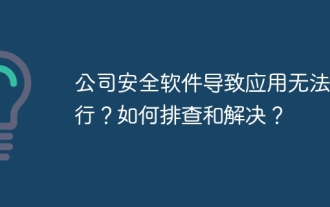
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
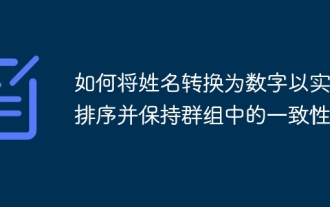
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
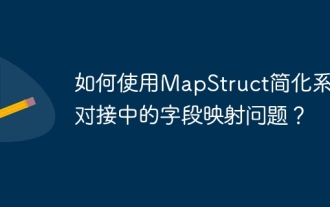
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
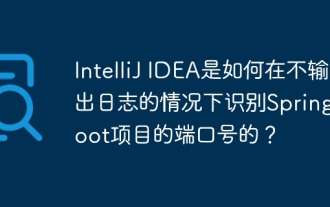
Start Spring using IntelliJIDEAUltimate version...
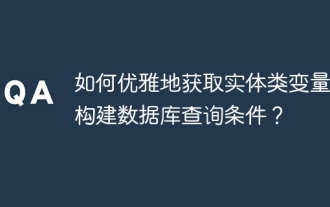
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
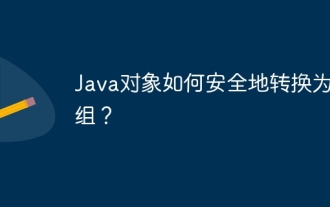
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
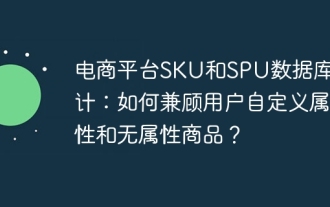
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
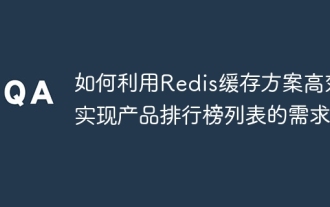
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
