Pure Components in React: Unlocking Performance
Aug 26, 2024 pm 09:42 PMIn modern React development, performance is often a key focus, especially as applications grow in complexity. One of the most effective ways to optimize performance is by leveraging Pure Components in React. Pure Components offer a powerful optimization technique, reducing unnecessary re-renders and ensuring your applications run faster and smoother. In this blog, we'll dive into what Pure Components are, when and how to use them, and why they are crucial for performance tuning in React applications.
What Are Pure Components in React?
In React, a Pure Component is essentially a more optimized version of a regular React component. Pure Components render the same output for the same state and props, and they implement a shallow comparison of props and state in the shouldComponentUpdate lifecycle method.
Here’s a simple example to show how a Pure Component can be implemented:
import React, { PureComponent } from 'react'; class MyComponent extends PureComponent { render() { return <div>{this.props.name}</div>; } }
In this example, MyComponent is a Pure Component. It will only re-render if there’s a change in the props or state that affects the component. The shallow comparison allows it to determine whether a re-render is necessary, saving performance.
For comparison, here’s how a regular component behaves:
import React, { Component } from 'react'; class MyComponent extends Component { render() { return <div>{this.props.name}</div>; } }
Unlike Pure Components, regular components don’t automatically check whether updates are necessary; they always re-render when the parent component re-renders. By switching to Pure Components where applicable, we can reduce these unnecessary renders.
To learn more about the core functionality of Pure Components, check out the official React documentation on PureComponent.
Why Use Pure Components?
The primary reason to use Pure Components in React is to optimize performance. React apps can become sluggish when multiple components unnecessarily re-render on every state or props update. Pure Components mitigate this by using a shallow comparison in the shouldComponentUpdate lifecycle method.
Here’s how that works:
If a Pure Component receives new props or state, it compares the new values with the previous ones. If they haven’t changed, React skips the re-render. This optimization is particularly useful in components that deal with complex data structures or perform resource-heavy operations.
Let’s look at an example:
class ParentComponent extends React.Component { state = { counter: 0 }; incrementCounter = () => { this.setState({ counter: this.state.counter + 1 }); }; render() { return ( <div> <button onClick={this.incrementCounter}>Increment</button> <MyPureComponent counter={this.state.counter} /> </div> ); } }
class MyPureComponent extends React.PureComponent { render() { console.log('MyPureComponent re-rendered'); return <div>{this.props.counter}</div>; } }
In this example, MyPureComponent only re-renders when the counter prop changes, despite any other updates in the parent component. Try it yourself: wrap a regular component instead of the Pure Component and observe the unnecessary re-renders in action.
How to Use Pure Components in React
Pure Components can be used in almost any scenario where shallow comparison suffices. The critical point is ensuring that your props and state structures are simple enough for a shallow comparison to work correctly. For example, Pure Components work well with primitive types such as strings and numbers but might not be as effective with nested objects or arrays, where changes to deep properties might be missed.
Here’s an example that highlights the limitations of shallow comparison:
class MyPureComponent extends React.PureComponent { render() { return <div>{this.props.user.name}</div>; } } const user = { name: 'John Doe' }; <MyPureComponent user={user} />
If you mutate the user object directly (e.g., by updating user.name), the shallow comparison might not detect the change because the reference to the user object hasn’t changed. To avoid such issues, always ensure that new objects or arrays are created when their contents are updated.
When to Use Pure Components in React
Pure Components are best suited for components that primarily rely on props and state that don’t change frequently or are composed of simple data structures. They work particularly well in larger React applications where reducing the number of re-renders significantly improves performance.
Here are some situations where Pure Components make the most sense:
- Stateless Components: Pure Components excel when props stay consistent over time.
- Rendering Performance: In components that are re-rendered frequently but rarely change their data, using Pure Components can improve overall app performance.
- Static Data: If your component deals with large amounts of static data, Pure Components help prevent unnecessary re-rendering of that data.
That said, they may not be appropriate for every use case. If your component relies on deep data structures or if shallow comparison isn’t sufficient to detect changes, Pure Components may lead to bugs. In such cases, consider using shouldComponentUpdate for more precise control.
Potential Pitfalls of Pure Components
While Pure Components in React can dramatically improve performance, there are a few potential pitfalls you should be aware of:
1. Shallow Comparison: As mentioned earlier, shallow comparison only checks the references of props and state. If you’re working with deeply nested objects or arrays, it may not detect changes, leading to potential bugs.
2. Over-Optimization: It’s essential to measure performance improvements before prematurely optimizing your code with Pure Components. Over-optimizing parts of your app that don’t need it can add unnecessary complexity and obscure the logic of your components.
3. Immutability Requirements: Because Pure Components rely on reference equality, maintaining immutability in your React state becomes more critical. Mutating objects or arrays directly can cause the shallow comparison to fail.
How CodeParrot AI Can Help with Pure Components in React
Integrating Pure Components into your React codebase can significantly enhance performance, but identifying the right components and implementing the optimizations can be time-consuming. This is where CodeParrot AI steps in to simplify and supercharge your development workflow.
CodeParrot AI analyzes your React project and identifies areas where Pure Components can make a real difference. It follows your coding standards, ensuring that the optimized code fits seamlessly into your existing codebase—no awkward formatting or unnecessary refactoring required. Instead of manually combing through your components to decide where Pure Components might improve performance, CodeParrot AI does the heavy lifting for you.
Conclusion
By understanding and using Pure Components in React, you can significantly optimize your application's performance. Pure Components reduce unnecessary re-renders by using a shallow comparison of props and state, making your apps more efficient and responsive. While they offer many benefits, remember to use them judiciously and only in cases where they make sense. With tools like CodeParrot AI, identifying and implementing Pure Components becomes even easier, allowing you to focus on building features rather than micro-optimizing performance.
The above is the detailed content of Pure Components in React: Unlocking Performance. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
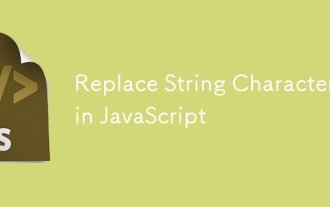
Replace String Characters in JavaScript
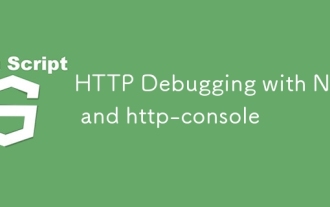
HTTP Debugging with Node and http-console
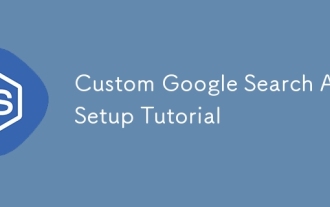
Custom Google Search API Setup Tutorial
