Python Tuples, Sets & Dictionaries || Day #f #daysofMiva
It's Day #5 of #100daysofMiva - See GitHub Projects
.
I delved into three fundamental data structures in Python: Tuples, Sets, and Dictionaries. These structures are essential for efficiently organizing and accessing data, each with unique characteristics and use cases. Here’s a detailed report of what I learned, including the processes, technicalities, and code examples.
1. Tuples
Definition: A tuple is an immutable, ordered collection of elements. Tuples are similar to lists but with the key difference that they cannot be modified after creation.
Characteristics:
Immutable: Once created, the elements of a tuple cannot be changed (no item assignment, addition, or removal).
Ordered: Elements maintain their order, and indexing is supported.
Heterogeneous: Tuples can store elements of different types.
Creating Tuples
Tuples can be created using parentheses () or simply by separating elements with commas.
python my_tuple = (1, 2, 3) another_tuple = "a", "b", "c" singleton_tuple = (42,) # Note the comma, necessary for single element tuples
Accessing Elements
Elements can be accessed via indexing, similar to lists.
python Copy code first_element = my_tuple[0] last_element = my_tuple[-1]
Tuple Unpacking
Tuples allow for multiple variables to be assigned at once.
python Copy code a, b, c = my_tuple print(a) # 1 print(b) # 2 print(c) # 3
Why Use Tuples?
Performance: Tuples are generally faster than lists due to their immutability.
Data Integrity: Immutability ensures that the data cannot be altered, making tuples ideal for fixed collections of items.
Hashable: Because they are immutable, tuples can be used as keys in dictionaries or elements in sets.
2. Sets
Definition: A set is an unordered collection of unique elements. Sets are commonly used for membership testing and eliminating duplicate entries.
Characteristics:
Unordered: No order is maintained, so indexing is not possible.
Unique Elements: Each element must be unique; duplicates are automatically removed.
Mutable: Elements can be added or removed, although the elements themselves must be immutable.
Creating Sets
Sets are created using curly braces {} or the set() function.
python Copy code my_set = {1, 2, 3, 4} another_set = set([4, 5, 6]) # Creating a set from a list empty_set = set() # Note: {} creates an empty dictionary, not a set
Basic Set Operations
Sets support various operations like union, intersection, and difference.
python # Union union_set = my_set | another_set print(union_set) # {1, 2, 3, 4, 5, 6} # Intersection intersection_set = my_set & another_set print(intersection_set) # {4} # Difference difference_set = my_set - another_set print(difference_set) # {1, 2, 3}
Membership Testing
Sets are optimized for fast membership testing.
python print(3 in my_set) # True print(7 in my_set) # False
Why Use Sets?
Unique Elements: Ideal for storing items where uniqueness is required.
Efficient Operations: Operations like membership testing and set algebra (union, intersection) are faster compared to lists.
Eliminating Duplicates: Converting a list to a set is a common technique to remove duplicates.
3. Dictionaries
Definition: A dictionary is an unordered collection of key-value pairs. Each key in a dictionary is unique and maps to a value.
Characteristics:
Key-Value Pairs: Keys are unique and immutable, while values can be of any type.
Unordered: Prior to Python 3.7, dictionaries were unordered. From Python 3.7 onwards, they maintain insertion order.
Mutable: Dictionaries can be modified by adding, removing, or changing key-value pairs.
Creating Dictionaries
Dictionaries are created using curly braces {} with key-value pairs separated by colons.
python my_dict = {"name": "Alice", "age": 30, "city": "New York"} another_dict = dict(name="Bob", age=25, city="Los Angeles") empty_dict = {}
Accessing Values
Values are accessed using their keys.
`python
name = my_dict["name"]
age = my_dict.get("age") # Using get() to avoid KeyError`
Adding and Modifying Entries
Dictionaries are dynamic; you can add or modify entries on the fly.
python my_dict["email"] = "alice@example.com" # Adding a new key-value pair my_dict["age"] = 31 # Modifying an existing value
Removing Entries
Entries can be removed using del or the pop() method.
python del my_dict["city"] # Removing a key-value pair email = my_dict.pop("email", "No email provided") # Removes and returns the value
*Dictionary Methods
*
Dictionaries have a variety of useful methods:
python keys = my_dict.keys() # Returns a view of the dictionary's keys values = my_dict.values() # Returns a view of the dictionary's values items = my_dict.items() # Returns a view of the dictionary's key-value pairs
Why Use Dictionaries?
Key-Based Access: Ideal for scenarios where data needs to be quickly retrieved via a unique identifier (the key).
Dynamic Structure: Useful for data structures that need to grow and change over time.
Efficient: Key-based access is typically faster than searching through a list or tuple.
The above is the detailed content of Python Tuples, Sets & Dictionaries || Day #f #daysofMiva. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
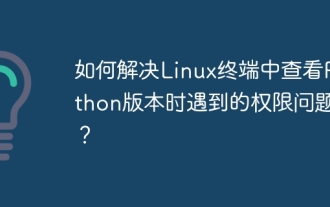
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
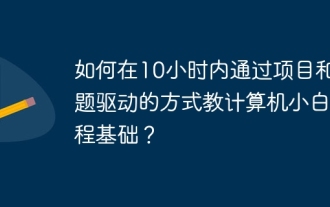
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
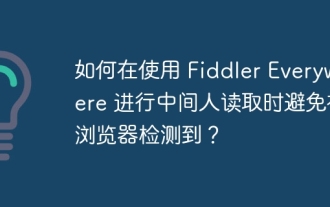
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
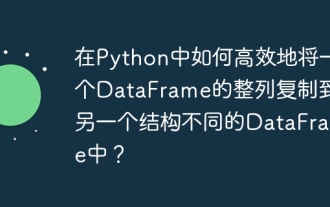
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
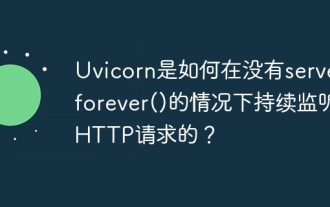
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
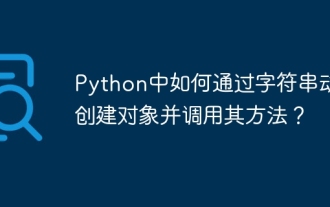
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
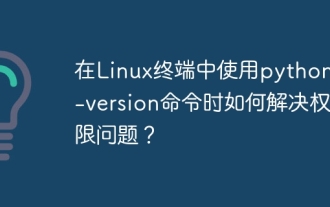
Using python in Linux terminal...
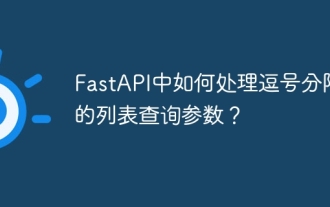
Fastapi ...
