Closures in JavaScript are an important and powerful feature that helps to understand the various functionalities and patterns of the JavaScript programming language. To discuss closures in detail, it is necessary to know about the concepts of scope and lexical environment (where the function is declared).
- They are discussed below:-
1. Scope:- Scope is a specific field or environment where a variable, function or object can be accessed. There are two main types of scopes in JavaScript:-
-
Global Scope: This is the default scope where any variable or function can be accessed throughout the script.
var myGlobalVar = "This is global!";
function printSomething() {
console.log(myGlobalVar);
}
printSomething(); // Output: This is global!
Copy after login
-
Local/Function Scope: It is used to access variables or functions inside a specific function or block.
function printSomething() {
var myLocalVar = "I'm local!";
console.log(myLocalVar);
}
printSomething(); // Output: I'm local!
// Not allowed
console.log(myLocalVar); // Output: Uncaught ReferenceError: myLocalVar is not defined
Copy after login
? Closures is basically related to Local/Function Scope. Local/Function Scope indicates the accessibility of variables within a function, whereas global scope refers to the access of variables throughout the entire script.
2. Lexical Environment:- Lexical Environment is an object that contains all the variables and function references of the current execution context. Each function creates its own lexical environment during execution.
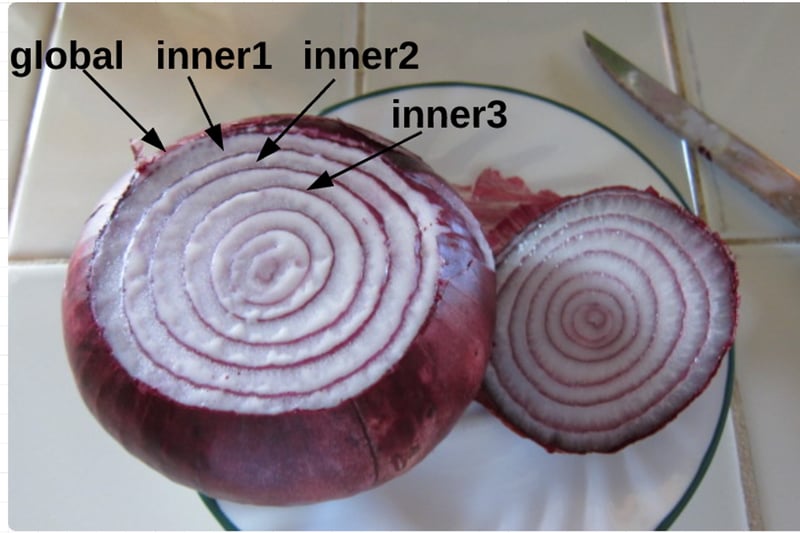
How do Closures work?
When a function is defined, that function creates a closure. This closure contains the code of the function and the variables or functions within the lexical scope of that function. When the function is called, the closure creates a new execution context and variables and functions within that context can be accessed.
- Now let's explain how Closure works with an example:
function outerFunction() {
let outerVariable = 'I am from outer function';
function innerFunction() {
console.log(outerVariable); // এখানে outerVariable-কে access করছে
}
return innerFunction;
}
const closureFunc = outerFunction();
closureFunc(); // Output: I am from outer function
Copy after login
Explanation:
- এখানে outerFunction একটি ভেরিয়েবল outerVariable ডিক্লেয়ার করেছে এবং একটি innerFunction রিটার্ন করছে।
-
innerFunctionএর মধ্যে outerVariableকে এক্সেস করা হচ্ছে, যদিও innerFunction নিজে কোনো outerVariable ডিক্লেয়ার করে নাই।
- যখন closureFunc চালানো হয়, তখন outerVariable প্রিন্ট হবে, যদিও outerFunction ইতিমধ্যে execute হয়ে শেষ হয়ে গেছে। এটি সম্ভব হয়েছে closure-এর কারণে, যা outerFunctionএর lexical environment ধরে রেখেছে।
Closures-এর ব্যবহার:
-
Data Privacy/Encapsulation:- Closures প্রাইভেট ডেটা তৈরি করতে পারে যা শুধুমাত্র নির্দিষ্ট ফাংশনের মাধ্যমে অ্যাক্সেস করা যায়।
function counter() {
let count = 0;
return function() {
count++;
return count;
};
}
const myCounter = counter();
console.log(myCounter()); // Output: 1
console.log(myCounter()); // Output: 2
console.log(myCounter()); // Output: 3
Copy after login
-
Function Currying:- একটি ফাংশন যে অন্য ফাংশনকে তার আর্গুমেন্ট হিসেবে গ্রহণ করে এবং একটি নতুন ফাংশন রিটার্ন করে, সেটি কারিং বলে।
function multiply(a) {
return function(b) {
return a * b;
};
}
const multiplyBy2 = multiply(2);
console.log(multiplyBy2(5)); // Output: 10
Copy after login
-
Asynchronous Handling Operations:- Closures প্রায়ই asynchronous callback ফাংশনের ক্ষেত্রে ব্যবহৃত হয়, যেমন setTimeout বা event listeners.
function asyncFunction() {
let message = 'Hello, World!';
setTimeout(function() {
console.log(message);
}, 1000);
}
asyncFunction(); // Output: Hello, World! (1 সেকেন্ড পরে)
Copy after login
Closures-এর সুবিধা:
-
ডেটা প্রাইভেসি: Closures sensitive ডেটা encapsulate করতে পারে এবং সেই ডেটা বাইরের জগতে অ্যাক্সেস থেকে নিরাপদ রাখে।
-
মেমরি ইফিশিয়েন্সি: একটি ফাংশন তার lexical scope ধরে রাখে, তাই closure যথাযথভাবে ব্যবহৃত হলে এটি মেমরি ইফিশিয়েন্ট হয়।
Closures-এর অসুবিধা:
-
মেমরি লিক: যদি Closures অনুপযুক্তভাবে ব্যবহৃত হয়, তাহলে এটি মেমরি লিকের কারণ হতে পারে, কারণ এটি প্রয়োজনের চেয়ে বেশি ডেটা ধরে রাখতে পারে।
-
ডিবাগিং সমস্যা: Closures জটিল হতে পারে এবং ডিবাগিং কঠিন হয়ে যায়, কারণ যখন আপনি বিভিন্ন ভেরিয়েবলের ভ্যালু ট্র্যাক করেন, তখন সেগুলি কোথায় সংরক্ষিত আছে তা স্পষ্ট নাও হতে পারে।
JavaScript-এ Closures একটি মৌলিক ও শক্তিশালী কনসেপ্ট যা আপনাকে অনেক উন্নত ও কার্যকরী কোড লিখতে সহায়তা করবে।
The above is the detailed content of Detailed discussion about JavaScript Closures. For more information, please follow other related articles on the PHP Chinese website!