PHP unset()
The following article provides an outline on PHP unset(). The primary operation of the method unset() is to destroy the variable specified as an input argument for it. In other words, it performs a reset operation on the selected variable. However, its behavior can vary depending on the type of variable that is being targeted to destroy. This function is supported by version PHP4 onwards.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax of PHP unset()
unset(mixed $selectedvar, mixed $selectedvar1,….., mixed $selectedvarN): void
- selectedvar: The mandatory argument for unset() method. At least one variable to unset, needs to be given as input argument for the method.
- selectedvarN: The optional parameter which can be given as input argument, to unset() method to reset it.
Use Cases for unset()
Given below are the different cases:
1. Applying unset() for local variable
When the local variable is passed to unset function, the function resets the variable.
Example:
Code:
<?php $input = "I have value!!!"; echo "The value of 'input' before unset: " . $input . "<br>"; unset($input); //Applying unset() method on $input variable echo "The value of 'input' after unset: " . $input; ?>
Output:
The value contained in the variable ‘input’ is destroyed on execution of the unset() method.
2. Applying unset for variable inside a function which is global variable
When user attempts to use Unset for a variable within a function and it is also defined as global variable, then unset() resets only the local one. The global one remains unaffected.
Example:
Code:
<?php function Afunction() { $Avariable = 'local value'; echo "Within the function scope before unset: ".$Avariable."<br>"; global $Avariable; unset($Avariable); //Deletes the local ‘Avariable’ echo "Within the function scope after unset: ".$Avariable."<br>"; } $Avariable = 'Global Value'; //The global ‘Avariable’ echo "Out of the function scope before unset: ".$Avariable."<br>"; Afunction(); echo "Out of the function scope after unset: ".$Avariable."<br>"; ?>
Output:
The local version of the variable ‘Avariable’ is destroyed where as the global version remains intact.
3. Applying unset for global variable within a function
If the variable within the function is also declared as global variable and user needs to destroy the global one, then it can be achieved using the array[$GLOBAL].
Example:
Code:
<?php function Afunction() { $Avariable = 'local value'; echo "Within the function scope before unset: ".$Avariable."<br>"; global $Avariable; unset($GLOBALS['Avariable']); //Resets the global ‘Avariable’ echo "Within the function scope after unset: ".$Avariable."<br>"; } $Avariable = 'Global Value'; echo "Out of the function scope before unset: ".$Avariable."<br>"; Afunction(); echo "Out of the function scope after unset: ".$Avariable."<br>"; ?>
Output:
The local version of the variable ‘Avariable’ is not affected by the execution of the unset function whereas the global version of the variable is set to null value.
4. Applying unset() for pass by reference variable
If unset() is called on a variable that is passed to the function as reference, unset() resets only the local one. The variable instance in the calling environment retains as it is.
Example:
Code:
<?php function Afunction(&$Avariable) //’Avariable’ is the pass by reference { $Avariable = 'Internal value'; echo "Within the function scope before unset: ".$Avariable."<br>"; unset($Avariable); //Resets the local ‘Avariable’ echo "Within the function scope after unset: ".$Avariable."<br>"; } $Avariable = 'External Value'; echo "Out of the function scope before unset: ".$Avariable."<br>"; Afunction($Avariable); echo "Out of the function scope after unset: ".$Avariable."<br>"; ?>
Output:
The unset() method called in the pass by reference variable ‘Avariable’ resets only the content of the variable in the local scope without affecting the content from the external scope.
5. Applying unset() for static variable
When a static variable is set as input argument to unset() method, the variable gets reset for the remaining command in the function scope after the function unset() is called.
Example:
Code:
<?php function UnsetStatic() { static $staticvar; $staticvar++; echo "Before unset() method is called: $staticvar"."<br>"; //Deletes ‘staticvar’ only for the below commands within the scope of this ‘UnsetStatic’ function unset($staticvar); echo "after unset() method is called: $staticvar"."<br>"; } UnsetStatic(); UnsetStatic(); UnsetStatic(); ?>
Output:
The variable ‘staticvar’ is reset only for the commands followed after unset() method is called.
6. Applying unset() on an array element
The application of unset() method on an array element deletes the element from the array without exhibiting re-indexing operation.
Example:
Code:
<?php $arrayinput = [0 => "first", 1 => "second", 2 => "third"]; Echo "The array elements, before unset:"."<br>"; Echo $arrayinput[0]." ". $arrayinput[1]." ". $arrayinput[2]." "."<br>"; //Unset operation is called on the second element of the array ‘arrayinput’ unset($arrayinput[1]); Echo "The array elements, after unset:"."<br>"; Echo $arrayinput[0]." ". $arrayinput[1]." ". $arrayinput[2]." "; ?>
Output:
7. Applying unset() on more than one element at a time
The unset() method supports deleting multiple variable at once.
Example:
Code:
<?php $input1 = "I am value1"; $input2 = "I am value2"; $input3 = "I am value3"; echo "The value of 'input1' before unset: " . $input1 . "<br>"; echo "The value of 'input2' before unset: " . $input2 . "<br>"; echo "The value of 'input3' before unset: " . $input3 . "<br>"; echo "<br>"; //Reseting input1, input2 and input3 together in single command unset($input1,$input2,$input3); echo "The value of 'input1' after unset: " . $input1."<br>"; echo "The value of 'input2' after unset: " . $input2."<br>"; echo "The value of 'input3' after unset: " . $input3."<br>"; ?>
Output:
The above is the detailed content of PHP unset(). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










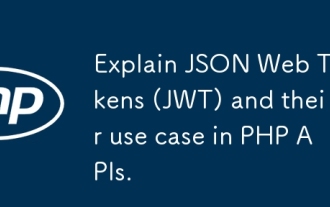
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
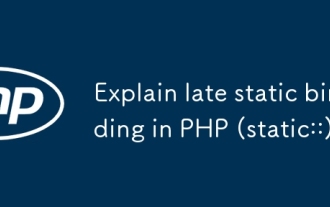
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
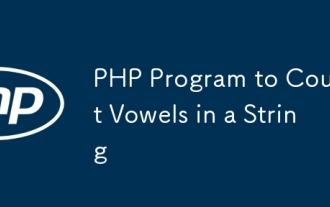
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
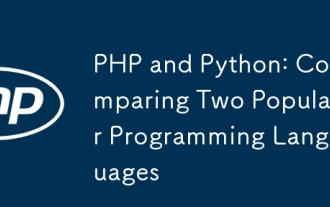
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
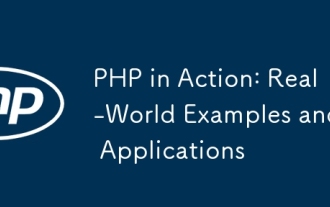
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
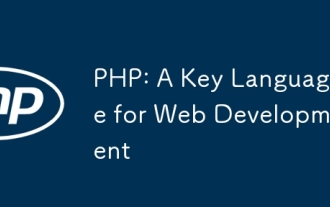
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
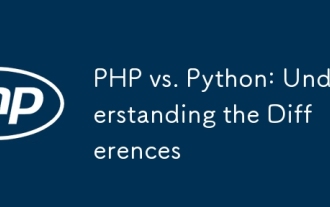
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
