Java Vector Sort
Java Vector Sort is a method of Java Vector Class used to sort vector according to the order by specified Comparator. As Vector in Java maintains the order of insertion of elements, the sort(,) method is used to sort the elements in ascending or descending order. Sort() method is a part of Collections class; vector elements can also be sorted using Comparable and Comparator. Like an array, Vector is also a growable object containing elements that can be accessed using an integer index. The size of the vector can grow or shrink by adding or removing elements after the vector is created.
ADVERTISEMENT Popular Course in this category JAVA MASTERY - Specialization | 78 Course Series | 15 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax:
The below is used as a declaration of sort() method,
public void sort(Comparator<? super E> c);
Mandatory Argument ‘c’ is the comparator used to compare vector elements. Returns nothing if the method has a return type void
There are no exceptions, and this is compatible with Java 1.2 version and above
Steps to Sort Vector using Comparator
- Creating a Vector object
- Adding elements to Vector using add method(Element e)
- Sorting Vector object using Collections.sort
- Displaying the list of sorted elements
Examples of Java Vector Sort
Here are the following examples mention below
Example #1
Simple Vector sort() using Collections.sort()
Code:
import java.util.Vector; import java.util.Collections; public class SortJavaVectorExample { public static void main(String[] args) { Vector vector = new Vector(); vector.add("10"); vector.add("31"); vector.add("52"); vector.add("23"); vector.add("44"); System.out.println("Vector elements before sorting: "); for(int x=0; x<vector.size(); x++) System.out.println(vector.get(x)); Collections.sort(vector); System.out.println("Sorted Vector elements in ascending order: "); for(int y=0; y<vector.size(); y++) System.out.println(vector.get(y)); } }
Output:
Declaring the vector, adding elements to the vector and printing the elements without sorting maintains the same insertion order. Using for loop, get(x) fetches element from index x
Collections.sort() sorts the vector elements in ascending order and are displaying using for loop.
Example #2
Vector sorting for Alphabetical order
Code:
import java.util.*; public class VectrSort { public static void main(String arg[]) { Vector < String > v = new Vector < String > (); v.add("Dragon Fruit"); v.add("Apple"); v.add("Watermelon"); v.add("Orange"); v.add("Strawberry"); System.out.println("Elements of Vector are: "); for (String fruit: v) { System.out.println(" "+fruit); } Collections.sort(v); System.out.println("Vector elements after sorting are: "); for (String fruit : v) { System.out.println(" "+fruit); } } }
Output:
We shall sort vector elements in descending order, i.e. reverseOrder() method of Collections class has to be invoked, which naturally imposes reverse order of the vector elements.
Example #3
Sorting Vector Elements in Reverse Order.
Code:
import java.util.Vector; import java.util.Comparator; import java.util.Collections; public class reverseSortArray { public static void main(String[] args) { Vector v = new Vector(); v.add("ColorRed"); v.add("ColorYellow"); v.add("ColorBlue"); v.add("ColorBlack"); v.add("ColorOrange"); v.add("ColorGreen"); System.out.println("Vector Elements before sorting :"); for(int x=0; x < v.size(); x++) System.out.println(v.get(x)); Comparator comparator = Collections.reverseOrder(); Collections.sort(v,comparator); System.out.println("Vector Elements after reverse sorting :"); for(int x=0; x < v.size(); x++) System.out.println(v.get(x)); } }
Output:
So here, vector Elements are sorted in descending order based on the alphabets.
Let us look at the sorting of vector elements of Custom Class Objects.
The above-mentioned Collections.sort() method works only if the elements class is implementing Comparable Interface; if not implemented, we will face compilation error.
Here, we shall see how the custom class implements the Comparable Interface.
Example #4
Vector Sorting for Custom Class Object.
Code:
import java.util.Collections; import java.util.Vector; class Employee implements Comparable<Employee>{ private int empid; public Employee(int empid){ this.empid = empid; } public String toString(){ return "Employee[" + this.empid + "]"; } public int getempId(){ return this.empid; } public int compareTo(Employee otherEmployee) { return this.getempId() - otherEmployee.getempId(); } } public class EmployeeSortVector { public static void main(String[] args) { Vector<Employee> vEmp = new Vector<Employee>(); vEmp.add(new Employee(110)); vEmp.add(new Employee(121)); vEmp.add(new Employee(102)); vEmp.add(new Employee(145)); vEmp.add(new Employee(1)); Collections.sort(vEmp); System.out.println(vEmp); } }
Output:
To sort the above elements in reverse order, reverseComparator is to be used.
Collections.sort(vEmp, Collections.reverseOrder())
We can also sort custom class object using a custom Comparator. Previously, we saw how Comparable Interface is implemented; now, we will create a custom comparator for the class objects.
Example #5
Vector Sort of a custom class object using Custom Comparator.
Code:
import java.util.Collections; import java.util.Comparator; import java.util.Vector; class Employee{ private int empid; public Employee(int empid){ this.empid = empid; } public String toString(){ return "Employee[" + this.empid + "]"; } public int getempId(){ return this.empid; } } class EmployeeComparator implements Comparator<Employee>{ public int compare(Employee emp1, Employee emp2) { return emp1.getempId() - emp2.getempId(); } } class EmployeeComparatorDesc implements Comparator<Employee>{ public int compare(Employee emp1, Employee emp2) { return emp2.getempId() - emp1.getempId(); } } public class SortJavaVectorExample { public static void main(String[] args) { Vector<Employee> vEmp = new Vector<Employee>(); vEmp.add(new Employee(346)); vEmp.add(new Employee(111)); vEmp.add(new Employee(211)); vEmp.add(new Employee(533)); vEmp.add(new Employee(211)); vEmp.add(new Employee(25)); Collections.sort(vEmp, new EmployeeComparator()); System.out.println(vEmp); Collections.sort(vEmp, new EmployeeComparatorDesc()); System.out.println(vEmp); } }
Output:
With this, we shall conclude our topic ‘Java Vector Sort’. We have seen what is Vector sorting in Java and how it is implemented. Its Syntax with the required arguments is also explained. Also discussed on How Vector Sorting is done and have seen all the types of examples, Sorting in Ascending order using Collections.sort(), descending order with Collections.reverseOrder() method. On top of this, we have also sorted for custom class objects using Comparable and also have customized the comparable for custom class objects. I hope we have covered the maximum areas of the topic. Thanks! Happy Learning!
The above is the detailed content of Java Vector Sort. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










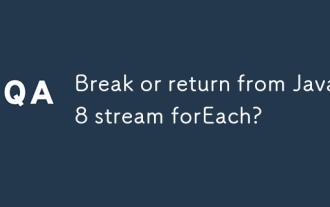
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
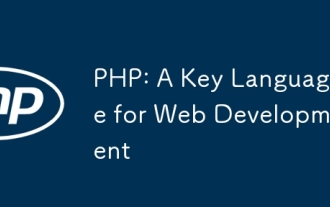
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
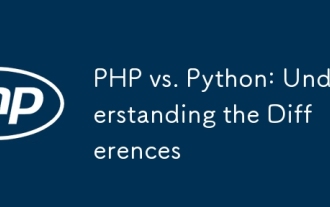
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
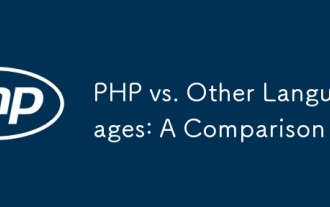
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
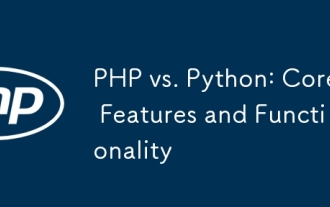
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
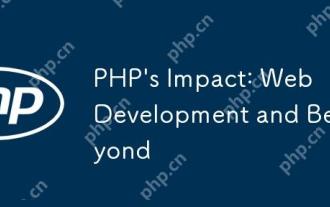
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
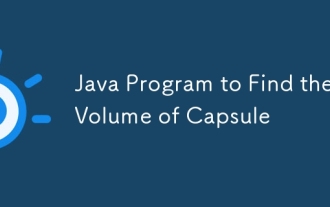
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
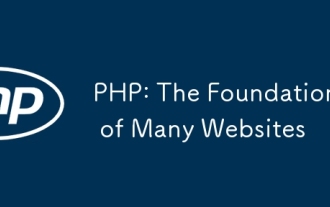
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
