Cohesion in Java
The Java Cohesion is defined as used to perform the specialized tasks (single task) instead of multiple tasks with a single java class. If a class is creating with high cohesion then it is said to targeted towards a single specific purpose, rather than performing different tasks at a time.
ADVERTISEMENT Popular Course in this category JAVA MASTERY - Specialization | 78 Course Series | 15 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Real-Time Example: Let’s assume we have to find out factorial of n numbers and display the output to the user. If we take a single class for finding factorial and displaying output. If the client always wants to change displaying output in different styles then we have to change the same class again and again without modifying factorial logic. This is bad practice in development, so take a class for factorial logic and another class for display output logic then now we can only change the display logic class always. It is said to high cohesion practice.
Types of Cohesion
There are 2 types of Cohesions
1. Low Cohesion
When class is designed to perform many different tasks instead of focusing on any specific task then that class is called “Low Cohesive class”. This kind of approach is the bad programming design approach. It required a lot of modifications for small change.
Syntax:
class Operations { //code for multiplication //code for division //code for addition //code for subtraction . . . }
Explanation: All the task are performed within the same class so that if any modification occurs we have change almost entire code.
2. High Cohesion
When class is designed to perform any specific task then that class is called as “High Cohesive class”. This kind of approach is good programming design approach. It can easily maintain and less modifiable
Syntax:
class Multiplication { //code } class Division { //code } class Addition { //code } class Multiplication { //code }
Explanation: Easy to maintain and less modifiable as each class has their own specific task.
How Does Cohesion Work in Java?
Cohesion works based on providing specific logic for each class provide better maintainability and better readability.
Syntax:
class Task { //logic for task } class Display { //display task here }
Examples of Cohesion in Java
Here are the examples mention below:
Example #1 – Multiplication Cohesion
Code:
package com.cohesion; import java.util.Scanner; //Multiplication logic class class Multiplication { // method for providing logic for multiplication of 2 numbers public static double getMultiplication(double x, double y) { // return the multiplcation output return x * y; } } // Output display class public class MultiplicationCohesion { public static void main(String args[]) { // scanner class for ask user input Scanner scanner = new Scanner(System.in); System.out.println("Enter any two numbers=>"); // ask the user 2 numbers double firstNumber = scanner.nextDouble(); double secondNumber = scanner.nextDouble(); // display the multiplication output System.out.println("Muliplication of " + firstNumber + " and " + secondNumber + " is " + Multiplication.getMultiplication(firstNumber, secondNumber)); scanner.close(); } }
Output:
Example #2 – Factorial Cohesion
Code:
package com.cohesion; import java.util.Scanner; //class for factorial class Factorial { // method for factorial logic public static int getFactorial(int inputNumber) { int factorial = 1; // calculating the factorial for (int temp = 1; temp <= inputNumber; temp++) { factorial = factorial * temp; } // returning the factorial return factorial; } } public class FactorialCohesion { public static void main(String args[]) { // scanner class for ask user input Scanner scanner = new Scanner(System.in); System.out.println("Enter any number=>"); // ask the user 1 number int number = scanner.nextInt(); // display the factorial output System.out.println("Factorial of " + number + " is " + Factorial.getFactorial(number)); scanner.close(); } }
Output:
Example #3 – Perfect number Cohesion
Code:
package com.cohesion;
import java.util.Scanner; //class for perfect number class PerfectNumber { static int sum = 0; // method for perfect number logic public static int getPerfectNumber(int inputNumber) { for (int i = 1; i < inputNumber; i++) { if (inputNumber % i == 0) { sum = sum + i; } } return sum; } } public class PerfectNumberCohesion { public static void main(String args[]) { // scanner class for ask user input Scanner scanner = new Scanner(System.in); System.out.println("Enter any number=>"); // ask the user 1 number int number = scanner.nextInt(); int output = PerfectNumber.getPerfectNumber(number); // checking sum and entered number if (output == number) { // display the perfect number output System.out.println(number + " is a Perfect Number"); } else { // display the perfect number output System.out.println(number + " is not a Perfect Number"); } scanner.close(); } }
Output:
Example #4 – Palindrome number Cohesion
Code:
package com.cohesion; import java.util.Scanner; //class for polindrome number class Polindrome { static int sum = 0; // method for polindrome number logic public static int getPolindromeNumber(int inputNumber) { int r, sum = 0, temp; temp = inputNumber; while (inputNumber > 0) { r = inputNumber % 10; // getting remainder sum = (sum * 10) + r; inputNumber = inputNumber / 10; } return sum; } } public class PolindromeCohesion { public static void main(String args[]) { // scanner class for ask user input Scanner scanner = new Scanner(System.in); System.out.println("Enter any number=>"); // ask the user 1 number int number = scanner.nextInt(); int output = Polindrome.getPolindromeNumber(number); // checking sum and entered number if (output == number) { // display the palindrome output System.out.println(number + " is a Polindrome Number"); } else { // display the palindrome output System.out.println(number + " is not a Polindrome Number"); } scanner.close(); } }
Output:
Conclusion
Java Cohesion is used to achieve better maintainability and less modifiable code by perform any specific task from each class. This is said to be High cohesion design programming. Real time applications high cohesion will be preferable.
The above is the detailed content of Cohesion in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
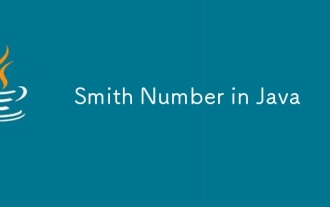
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
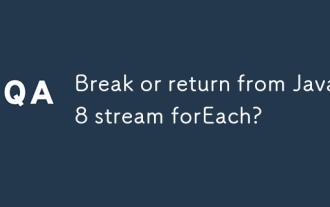
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
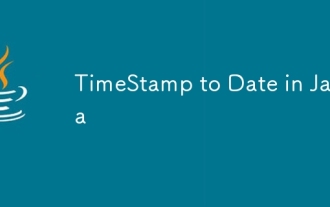
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
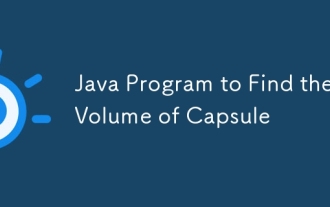
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
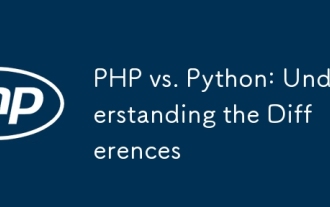
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
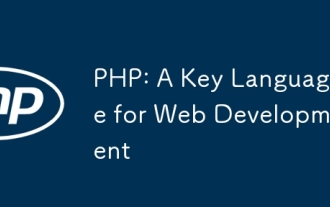
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
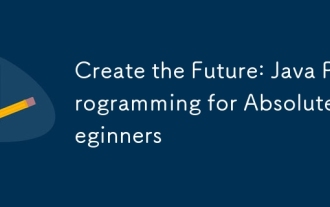
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
