Java Static Nested Class
Static inner classes is a nested class. This class is present as a static member under any class that acts as an outer class. It does not require a class object to be created to use member functions and variables. It can be instantiated with the help of the outer class. Also, it has a limitation that it can not access non-static member functions and member variables just like static variables.
Syntax:
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
The syntax of the JAVA static nested class is provided below. Here we need to have an outer class that will work as an umbrella class for the inner class. The inner class will lie inside the outer class loop. The inner class will have a “static” keyword before class so that the inner class can be recognized as a static inner or nested static class.
1 2 3 4 5 6 7 8 |
|
How does Java Static Nested Class Works?
JAVA static nested classes are declared by using the “static” keyword before class at the time of class definition. The static keyword is also used with methods and variables to maintain the scope of variables and methods throughout the program without them begin overridden. The same feature can be used on the class level but with the exception that it has to be inner class. The functioning of a nested static class can be well understood via examples explained in the next section.
Although there are some important points to note regarding JAVA static nested classes:
- The static word can not be used with the outer class. That means only if any class is in the inner class, then the static word can be used before class.
- The static nested class can access static member functions and static variables of the outer class along with its own. With respect to accessibility to the outer class, it can access even the private members if they are declared static.
- Static classes can access only static variables and methods.
- There is no need to create objects for static classes. There is a direct calling to member functions and variables that are assigned with the static keyword.
Examples to Implement Java Static Nested Class
Some examples, along with their explanation, are provided below for you to review.
Example #1
These examples demonstrate the use and understanding of static keywords in data flow during program processing.
Code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
Output:
Explanation: Static classes are not instantiated directly, so in this example, the static class is instantiated with the outer class’s help. The outer class “Test1” is defined with a static variable named “vnumber” and a static class called “Innerclass”. The method is defined under the inner class named “printmsg()”. This function contains a string and a variable that is defined in the outer class.
When a program is executed, the main function is called first. The outer class, along with the inner class with a dot operator, is used for instantiation. The instance named “object” is invoked. The object is then used to call function printmsg(). The printms() function brings the string stored in it and a variable with a value of 25 coming from the outer class static variable declaration. So we have used the class instantiation method to demonstrate the usage of the static nested class. One thing to note here is that the static inner class and the static variable is used, but the printmsg() function is non-static. This is the reason we instantiated in the above example. If this function would have been static, then we would have used it another way.
Example #2
Code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
Output:
Explanation: Here, we are demonstrating an example of a static nested function where the variable, class, and method all are defined statically. This is done to demonstrate the method calling directly instead of invoking an object to call a method. Since wherever the “static” keyword is used, there is not required to create an object. One can directly use it. So here, an outer class named “test2” is created with a static variable called “vnumber” and an inner class named “innerclass”. Innerclass is attached with a static keyword to demonstrate the use of the static nested class. The inner class has a static member function with its string and a variable.
The main function is called as the program is executed. Outer class, inner class, and a method are directly called via a dot operator. Here we did not create an object as we created in the previous example since the method itself is declared static.
Conclusion
Static nested classes can be useful when we want to restrict the use of member functions of outer function in an inner class. This is possible as an inner static class can access only the outer class’s static members while other non-static members of the outer class still need an object to be created if the inner class wants to use them. This is used in case the programmer is looking for some security to be inherited in his/her code. The static keyword makes sure that data is not manipulated easily. This encourages data security and reliability on the application.
The above is the detailed content of Java Static Nested Class. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










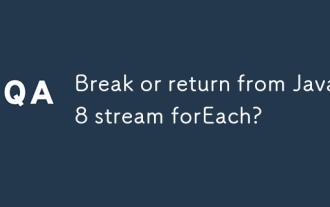
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
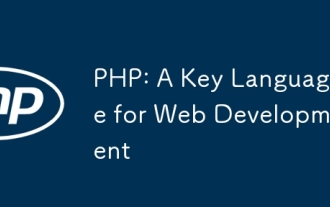
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
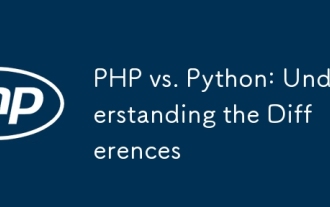
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
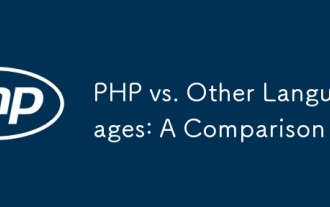
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
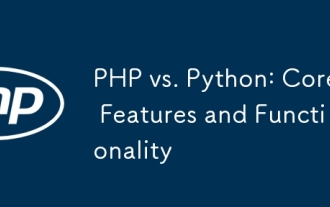
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
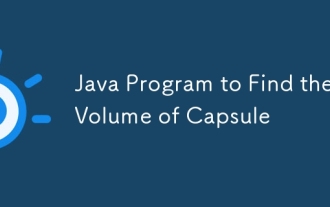
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
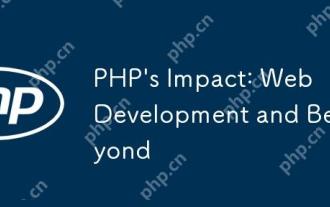
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
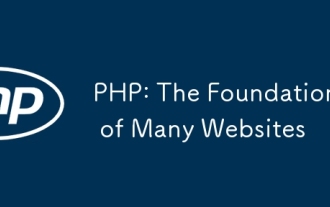
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
