Set Interface in Java
Java provides an interface to store and manipulate data known as Collection Interface. The collection is the super interface for a Set interface that helps store any type of object and manipulate it. Set interface stands out as a Collection that does not allows duplicate data in it, i.e. if d1 and d2 are two data entries in the same Set, then the result of d1.equals(d2) should be false. Almost one null element is allowed in Set. Set models the mathematical set abstraction.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Some of the implementations of sets are HashedSet, LinkedHashSet, or TreeSet as sorted representation.
Examples to Implement Set Interface in Java
Below are the examples of Set Interface in Java:
1. HashSet
Code:
import java.util.*; public class Main{ public static void main(String[] args) { // Set demonstration using HashSet Set<Integer > hash = new HashSet<Integer>(); hash.add(1); hash.add(4); hash.add(1); hash.add(3); hash.add(2); System.out.print("Set output without duplicates"); System.out.println(hash); } }
Output:
2. TreeSet
Code:
import java.util.*; public class Main{ public static void main(String[] args) { // Set demonstration using TreeSet Set<Integer> tree = new TreeSet<Integer>(); tree.add(1); tree.add(4); tree.add(1); tree.add(3); tree.add(2); System.out.print("Set output without duplicates and sorted data "); System.out.println(tree); } }
Output:
Methods of Set Interface in Java
Methods supported by Set for various data object storage and manipulation.
- add(Element e): Adds a specified element in the set.
- addAll(Collection> c): Adds all the elements present in the specified collection.
- clear(): Removes all the elements from the set.
- contains(Object o): Returns true if Set contains the same object as the specified object.
- containsAll(Collection> c): Returns true if set contains all of the elements in the specified collection.
- size(): Returns the number of elements in the Set.
- equals(Object o): It compares and returns true if our object is equal to the object specified.
- hashCode(): It returns the hashcode value for the set.
- isEmpty(): It returns true if the set contains no element.
- iterator(): It returns an iterator on the set, which helps to trace through the complete set.
- remove(Object o): Removes the specified element from the existing set.
- removeAll(Collection> c): Removes the specified collection from the existing set.
- toArray(): It returns the particular array containing all the elements as in Set.
Usage of methods in Our Code:
Code:
import java.util.LinkedHashSet; public class Main { public static void main(String[] args) { LinkedHashSet<String> linked = new LinkedHashSet<String>(); // Adding element to LinkedHashSet linked.add("Apple"); linked.add("Ball"); linked.add("Cat"); linked.add("Dog"); // Cannot add new element as Apple already exists linked.add("Apple"); linked.add("Egg"); System.out.println("Size of LinkedHashSet: " + linked.size()); System.out.println("Old LinkedHashSet:" + linked); System.out.println("Remove Dog from LinkedHashSet: " + linked.remove("Dog")); System.out.println("Trying Remove Zoo which is not present "+ "present: " + linked.remove("Zoo")); System.out.println("Check if Apple is present=" + linked.contains("Apple")); System.out.println("New LinkedHashSet: " + linked); } }
Output:
Converting HashSet to TreeSet
HashSet is generally used for search, delete and insert operations. HashSet is faster than TreeSet and uses a hash table. TreeSet whereas used for storing purpose due to its sorted data storage property. TreeSet uses TreeMap from the backend on Java. In order to store sorted data, insert elements into a hashmap and then insert data into a tree to get it sorted.
There are 3 ways to do this:
1. Pass the HashSet created
Code:
import java.util.*; public class Main { public static void main(String[] args) { Set<Integer > hash = new HashSet<Integer>(); hash.add(1); hash.add(4); hash.add(1); hash.add(3); hash.add(2); System.out.print("HashSet"); System.out.println(hash); //adding HashSet as a parameter to TreeSet constructor Set< Integer> treeSet = new TreeSet<>(hash); // Print TreeSet System.out.println("TreeSet: " + treeSet); } }
Output:
2. Using addAll() method
Code:
import java.util.*; public class Main { public static void main(String[] args) { Set<Integer > hash = new HashSet<Integer>(); hash.add(1); hash.add(4); hash.add(1); hash.add(3); hash.add(2); System.out.print("HashSet"); System.out.println(hash); //converting HashSet to TreeSet using addAll() method Set<Integer> treeSet = new TreeSet<>(); treeSet.addAll(hash); // Print TreeSet System.out.println("TreeSet: " + treeSet); } }
Output:
3. Using for-each loop
Code:
import java.util.*; public class Main { public static void main(String[] args) { Set<Integer > hash = new HashSet<Integer>(); hash.add(1); hash.add(4); hash.add(1); hash.add(3); hash.add(2); System.out.print("HashSet"); System.out.println(hash); //converting HashSet to TreeSet using for each loop Set<Integer> treeSet = new TreeSet<>(); for (Integer i : hash) { treeSet.add(i); } // Print TreeSet System.out.println("TreeSet: " + treeSet); } }
Output:
Recommended Article
This is a guide to Set Interface in Java. Here we discuss the Introduction to Set Interface and how it is used to store and manipulate data in Java and its methods. You can also go through our other suggested articles to learn more –
- Layout in Java
- Java Compilers
- Java Parallel Stream
- Java BufferedReader
The above is the detailed content of Set Interface in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










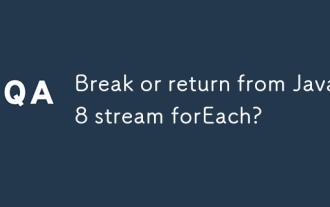
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
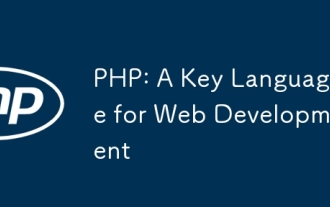
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
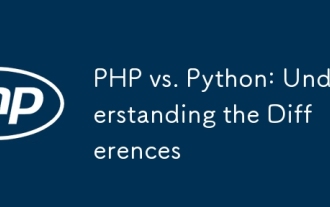
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
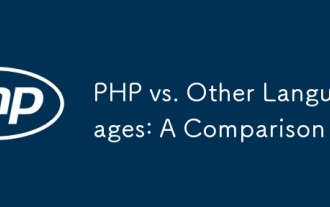
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
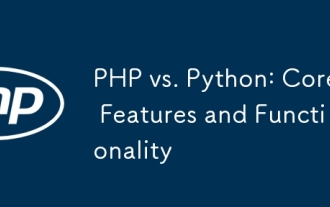
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
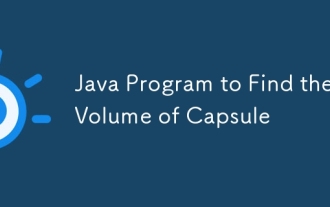
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
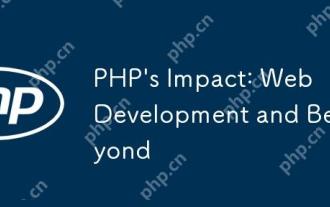
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
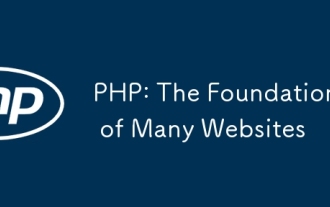
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
