Java Default Method
In Java 8, a new concept named the default method is introduced in order to perform backward compatibility that controls lambda expressions by old interfaces. Moreover, interfaces are allowed to have functions with implementation without causing any issues to the classes that interface will be implemented. Before the introduction of Java 8, only abstract methods are allowed by interfaces. Moreover, functions had to be offered in different classes. In the following sections, syntax, working, and examples of the default method will be explained.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax
Below is the syntax of a default method.
public interface animal { default void sound() { System.out.println("This is a sample default method. . .!"); }}
How does the Default Method work in Java?
As we know, interfaces such as List and collections do not have the method forEach. If it is added, framework implementation of collections will break. Since the default method is introduced in Java 8, a default implementation can be done for the method forEach. In addition to that, a class that implements 2 interfaces with the same default functions can be done. Let us see how the ambiguity of the code is resolved.
public interface livingthings { default void sound() { . . . System.out.println("Living things too make noise . . .") ; } } public interface animals { default void sound() { . . . System.out.println("animals too make noise . . .") ; } }
There are two solutions to this ambiguity.
1. To override the default method implementation, create your own method.
public class dogs implements livingthings,animals { default void sound() { . . . System.out.println("dogs bark . . .") ;} }
2. Using super, call the default method
public class dogs implements livingthings,animals { default void sound() { . . . livingthings.super.print("dogs bark . . .") ; } }
In Java, normally n interfaces can be implemented by a class. Moreover, an interface can be extended by another interface. Suppose there are two interfaces for a class and there is a default method implemented; the particular class may get confused in choosing which method to be considered for calling. In order to resolve these conflicts, the following points can be done.
- Overridden methods in the class are matched and called.
- If the method with the same method is provided, the most appropriate one will be chosen. Suppose there are two interfaces, A and B are present for a particular class. If A extends B, then A is the most specific here, and the default method will be selected from A. If A and b are independent interfaces, a severe conflict situation will occur, and a complaint will be raised by a compiler that it is unable to choose. In this situation, the user has to help the compiler by offering extra details from which A or B the default method has to be chosen. e.g.
A.super.demo() ;
or
B.super.demo() ;
Differences Between Normal Method and Default Method
Now, let us see some of the differences between a normal method and a default method
- The default method goes along with a default modifier, unlike normal methods.
- Arguments of the interfaces do not have any particular state for default methods.
- Normal methods can use as well as alter the arguments of the methods and class fields.
- New functionality can be added to the existing interfaces instead of breaking the previous implementation of those particular interfaces.
On extending an interface that consists of a default method, the following can be performed.
- The default method won’t be overridden, and it will be inherited.
- Default methods will be overridden, which are similar to methods that are overridden in subclasses.
- The default method should be again declared as abstract, which forces the subclass to be overridden.
Examples to Implement Java Default Method
The following are the sample programs mentioned:
Example #1
Java program to implement the default method
Code:
//Java program to implement default method public class DefExample { //main method public static void main(String args[]) { //create an object for the interface animal Animals obj = new Dog(); //call the print method obj.print( ); } } //create an interface animal interface Animals { //default method default void print( ) { System.out.println("I have four legs. . . ! !"); } static void sound() { System.out.println("I used to bark alot!!!") ; } } //create an interface <u>carnivores</u> interface Carnivores { //default method default void print( ) { System.out.println("I eat non veg. . .! !") ; } } //class that implements the other two interfaces class Dog implements Animals, Carnivores { public void print( ) { //call the print method of Animals using super Animals.super.print( ) ; //call the print method of Carnivores using super Carnivores.super.print( ); //call the sound method of Animals Animals.sound(); System.out.println("I am a Dog . . .!"); } }
Output:
Explanation: In this program, two interfaces, Animals and Carnivores, has the same default method print(), and they are called using super.
Example #2
Java program to implement the default method
Code:
//Java program to implement default method interface Sampleinterface{ // Since this is declared as a default method, this has to be implemented in the implementation classes default void sammethod(){ System.out.println("a default method which is newly added to the program"); } // existing public as well as abstract methods has to be implemented in the implementation classes void oldmethod(String s); } public class DefExample implements Sampleinterface{ // abstract method implementation public void oldmethod(String s){ System.out.println("The string given is: "+ s); } public static void main(String[] args) { DefExample obj = new DefExample(); //call the default method obj.sammethod(); //call the abstract method obj.oldmethod("I am the old method in the program"); } }
Output:
Explanation: In this program, an interface Sampleinterface is present, and it has a default method sammethod(), and it is called.
Conclusion
In Java 8, a new concept named the default method is offered for performing backward compatibility where old interfaces control lambda expressions. Moreover, interface arguments do not have any specific state for default methods. In this article, syntax, working, and examples of the default method are explained in detail.
The above is the detailed content of Java Default Method. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




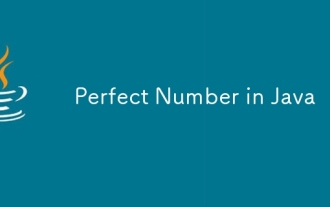
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
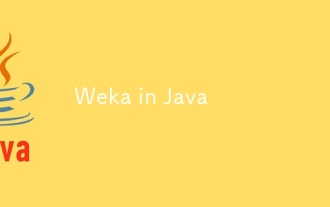
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
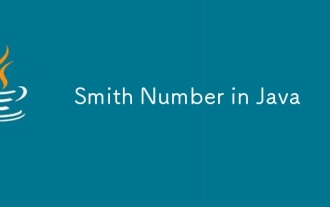
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
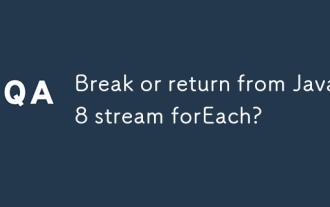
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
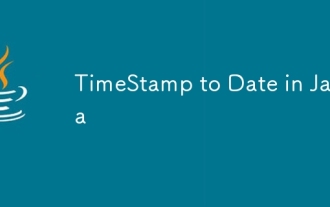
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
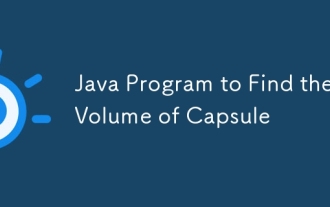
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
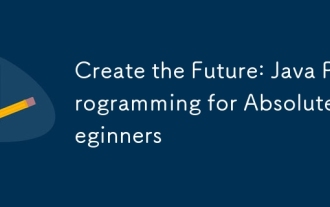
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
