Ways to Check If a Key Exists in a JavaScript Object
While working on your React project, you might encounter a situation where you need to render some data from an object. Before doing so, it’s crucial to verify whether a specific key is present in the object. But how do you check if a key exists in a JavaScript object? If you're unsure, don't worry—there are several ways to accomplish this!
1. Using the in Operator
One of the simplest ways to check if a key exists in a JavaScript object is by using the in operator. This operator checks for both own properties and properties inherited through the prototype chain.
const car = { make: 'Toyota', model: 'Corolla', year: 2020 }; console.log('make' in car); // true console.log('color' in car); // false
Pros:
- Easy to use and understand.
- Checks both own properties and inherited properties.
Cons:
- May return true for properties that are part of the prototype chain, which can sometimes lead to unexpected results.
2. Using hasOwnProperty()
The hasOwnProperty() method is another popular way to check if a key exists in a JavaScript object. It ensures that the key is an own property of the object, not something inherited.
const car = { make: 'Toyota', model: 'Corolla', year: 2020 }; console.log(car.hasOwnProperty('make')); // true console.log(car.hasOwnProperty('toString')); // false
Pros:
- Confirms that the key is an own property.
- Avoids false positives from inherited properties.
Cons:
- Requires a method call, making it slightly more verbose than the in operator.
3. Checking for undefined
You can also check if a key exists in a JavaScript object by verifying if the property value is undefined. In JavaScript, accessing a non-existent key returns undefined.
const car = { make: 'Toyota', model: 'Corolla', year: 2020 }; console.log(car.make !== undefined); // true console.log(car.color !== undefined); // false
Pros:
- Simple and intuitive.
- Useful if you also want to determine if a key’s value is undefined.
Cons:
- Doesn't differentiate between a key that doesn’t exist and a key that exists but has an undefined value.
4. Using Object.hasOwn()
Introduced in ECMAScript 2022, Object.hasOwn() provides a more modern approach to check if a key exists in a JavaScript object. It’s similar to hasOwnProperty(), but with a more concise syntax and improved reliability.
const car = { make: 'Toyota', model: 'Corolla', year: 2020 }; console.log(Object.hasOwn(car, 'make')); // true console.log(Object.hasOwn(car, 'color')); // false
Pros:
- Modern and cleaner syntax.
- Reliable even if an object overrides hasOwnProperty().
Cons:
- Requires ECMAScript 2022 or later, so it may not be supported in all environments.
5. Using Object.keys() and Array.includes()
For a more functional approach, you can convert the object’s keys to an array and use Array.includes() to check if a key exists in a JavaScript object.
const car = { make: 'Toyota', model: 'Corolla', year: 2020 }; console.log(Object.keys(car).includes('make')); // true console.log(Object.keys(car).includes('color')); // false
Pros:
- Allows for complex checks and conditions.
- Useful for scenarios where you need to work with the keys as an array.
Cons:
- Less efficient for large objects because it involves creating an array of keys.
When to Use Each Method
- in Operator: Ideal for quick checks where inherited properties are acceptable.
- hasOwnProperty(): Best when you need to confirm the key is an own property.
- undefined Check: Useful if you also need to determine whether the key's value is undefined.
- Object.hasOwn(): The preferred method in modern JavaScript for checking own properties, if supported in your environment.
- Object.keys() and Array.includes(): Suitable for more complex conditions or when working with key arrays.
Conclusion
Understanding how to efficiently check if a key exists in a JavaScript object is essential for writing robust JavaScript code. Each method has its own strengths and is suited to different scenarios, so choosing the right one depends on your specific needs. Whether you’re dealing with modern or legacy code, knowing these techniques will help you handle objects more effectively and avoid common pitfalls.
To learn more about JavaScript Objects check this.
The above is the detailed content of Ways to Check If a Key Exists in a JavaScript Object. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










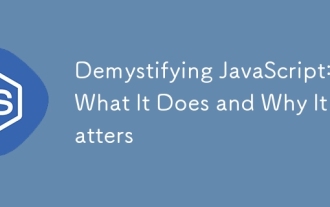
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
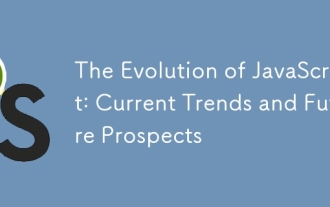
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
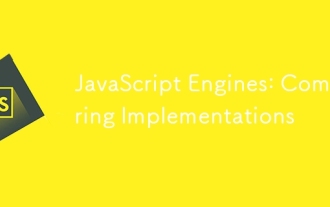
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
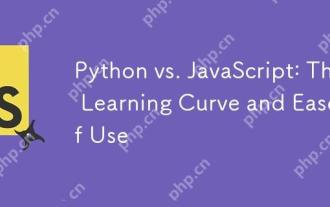
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
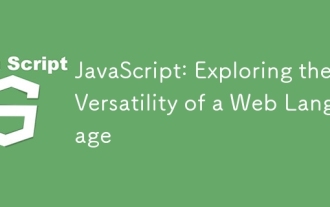
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
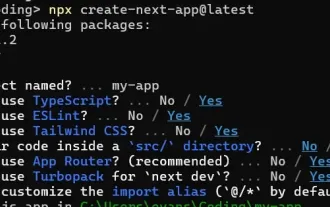
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
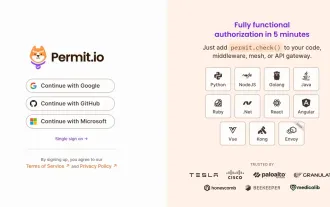
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
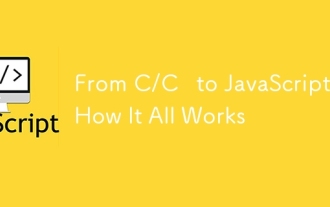
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
