Overloading and Overriding in C#
Polymorphism is one of the important concepts in C#. There are two types of polymorphism, compile time and run time. Overloading and Overriding concepts are used to achieve this respectively. In overriding, a child class can implement the parent class method in a different way but the child class method has the same name and same method signature as parent whereas in overloading there are multiple methods in a class with the same name and different parameters.
How Overriding and Overloading Works in C#?
The working of overriding and overloading in C# are explained below with examples:
Overriding
There are some keywords which we use in overriding like virtual, override and base.
Syntax:
class Parent { public virtual void Example() // base class { Console.WriteLine("parent class"); } } class Child: Parent { public override void Example() // derived class { base.Example(); Console.WriteLine("Child class"); } }
In this, virtual and override keywords are used which means the base class is virtual and child class can implement this class and override means this child class has the same name and same method signature as parent class.
Example #1
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace OverridingExample { class Subject // base class { public virtual void study() // base class method { Console.WriteLine("Study all the subjects"); } } class Mathematics: Subject // derived class { public override void study() // derived class method { Console.WriteLine("Study Mathematics"); } } class Program { // main method static void Main(string[] args) { Subject s = new Mathematics(); s.study(); Console.ReadLine(); } } }
In the above example, the methods name is the same but their implementation is different. The base class has virtual and due to that child class can implement parent class method in its own way. The child class method has keyword override which shows that this method is an override method.
Output:
Example #2
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace OverridingExample { class Subject // base class { public virtual void study() // base class method { Console.WriteLine("Study all the subjects"); } } class Mathematics: Subject // derived class { public override void study() // derived class method { base.study(); Console.WriteLine("Study Mathematics"); } } class Program { // main method static void Main(string[] args) { Mathematics m = new Mathematics(); m.study(); Console.ReadLine(); } } }
Output:
In this example, the derived class has a base keyword that is used to call the base class method. So, in that case, the derived method is called after the base class method.
Points to Remember:
- In the overriding concept, the name of the method and method signature and access modifier is always the same as parent and child class.
- The parent class method cannot be static.
Overloading
In overloading, there are multiple methods with different method signatures. Below are some examples that show how we can achieve overloading by varying the number of parameters, the order of parameters, and data types of parameters.
Example #1
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace OverloadingExample { class Demo { public int Sum(int x, int y) { int value = x + y; return value; } public int Sum(int x, int y, int z) { int value = x + y + z; return value; } public static void Main(string[] args) // main method { Demo d = new Demo(); int sum1 = d.Sum(24, 28); Console.WriteLine("sum of the two " + "integer value : " + sum1); int sum2 = d.Sum(10, 20, 30); Console.WriteLine("sum of the three " + "integer value : " + sum2); Console.ReadLine(); } } }
In the above example, there are two methods with the same name but a different number of parameters. The first method consists of two parameters while the second one has three parameters. This is called method overloading.
Output:
Example #2
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace OverloadingExample { class Demo { public int Sum(int x, int y, int z) { int value = x + y + z; return value; } public double Sum(double x, double y, double z) { double value = x + y + z; return value; } public static void Main(string[] args) // main method { Demo d = new Demo(); int sum1 = d.Sum(24, 28,7); Console.WriteLine("sum of the two " + "integer value : " + sum1); double sum2 = d.Sum(10.0, 20.0, 30.0); Console.WriteLine("sum of the three " + "integer value : " + sum2); Console.ReadLine(); } } }
In the above example, there are two methods with the same name but their data types are different. The first method has an integer data type while the second has a double data type. So in this case parameters are varying because of the different datatype.
Output:
Example #3
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace OverloadingExample { class Demo { public void Details(String name,int id) { Console.WriteLine("Name " + name + ", " + "Id " + id); ; } public void Details(int id,string name) { Console.WriteLine("Name " + name + ", " + "Id " + id); } public static void Main(string[] args) // main method { Demo d = new Demo(); d.Details("John", 10); d.Details("Joe", 20); Console.ReadLine(); } } }
In the above example, the name of the methods are the same but the order of parameters are different. The first method has a name and id resp. whereas the second one has id and name respectively.
Output:
Points to Remember:
- In an overloading concept, it is not possible to define more than one method with the same parameters in case of order, type and number.
- It is not possible to overload a method based on the different return types.
Advantages of Overloading and Overriding in C#
Following are the advantages explained.
- Overloading is one of the ways to achieve static and overriding is one of the ways by which C# achieves Dynamic polymorphism.
- It provides flexibility to the user and the cleanliness of the code.
Conclusion
Overloading and overriding play a major role in achieving polymorphism. Overriding allows derived class to implement in its own way and on the other hand overloading is about methods with the same name and various types of parameter implementations.
The above is the detailed content of Overloading and Overriding in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










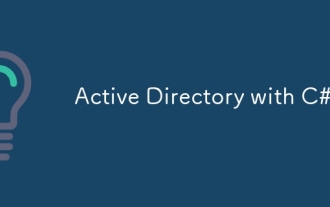
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
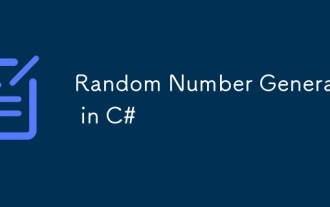
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
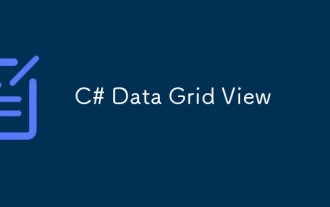
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
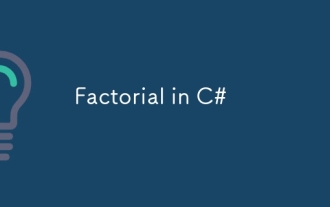
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
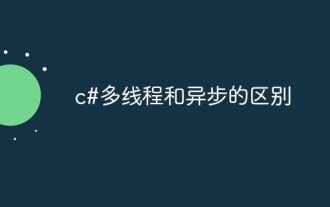
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
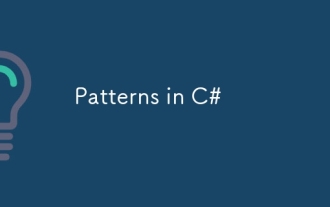
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
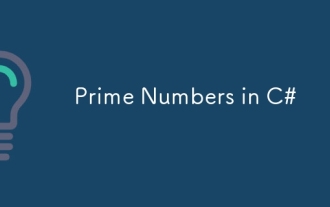
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
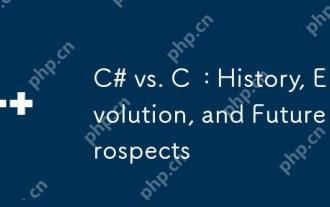
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
