Key Topics While Learning Django
1. Django Fundamentals
- Project Structure: Understanding the basic structure of a Django project (e.g., settings.py, urls.py, wsgi.py).
- Apps: Understanding how Django apps work within a project and how to create and manage them.
- URLs and Routing: Defining URL patterns and linking them to views.
- Views: Writing function-based views (FBVs) and class-based views (CBVs).
- Templates: Using Django's template language to create dynamic HTML pages.
2. Models and ORM (Object-Relational Mapping)
- Defining Models: Creating and managing database models in Django.
- Migrations: Understanding how Django migrations work to propagate model changes to the database.
- QuerySet API: Retrieving, filtering, and manipulating data using Django’s ORM.
3. Forms
- Django Forms: Creating and processing forms using Django's built-in form handling.
- Model Forms: Automatically creating forms based on Django models.
- Form Validation: Implementing custom validation logic.
4. Authentication and Authorization
- User Model: Working with Django's built-in User model.
- Authentication: Implementing login, logout, and password management features.
- Authorization: Managing user permissions and groups.
- Custom User Models: Extending or replacing the default User model.
5. Django Admin Interface
- Admin Customization: Customizing the Django admin panel to manage data.
- Admin Models: Registering models and customizing how they appear in the admin interface.
6. Static Files and Media Files
- Static Files: Serving CSS, JavaScript, and images in a Django application.
- Media Files: Handling user-uploaded files, including image and file uploads.
7. Middleware
- Understanding Middleware: Learning how middleware works and how to create custom middleware.
- Common Middleware: Using Django's built-in middleware for tasks like authentication, sessions, and security.
8. Django REST Framework (DRF)
- APIs with Django: Building RESTful APIs using Django REST Framework.
- Serializers: Converting Django models to JSON and vice versa.
- Viewsets and Routers: Simplifying views with DRF's viewsets and routers.
- Authentication in DRF: Implementing token-based or session-based authentication in APIs.
9. Security
- Cross-Site Scripting (XSS): Protecting your application from XSS attacks.
- Cross-Site Request Forgery (CSRF): Understanding and preventing CSRF attacks.
- SQL Injection: Preventing SQL injection through Django’s ORM.
- Authentication Best Practices: Ensuring secure login and password storage.
10. Deployment
- Deploying Django: Deploying a Django application to production environments (e.g., using platforms like Heroku, AWS, or DigitalOcean).
- WSGI and ASGI: Understanding the role of WSGI/ASGI in deploying Django applications.
- Static and Media Files in Production: Serving static and media files in a production environment.
11. Testing
- Unit Testing: Writing unit tests for your Django views, models, and forms.
- Integration Testing: Testing the interaction between different parts of your Django application.
- Test Coverage: Ensuring your tests cover all important code paths.
12. Performance Optimization
- Database Optimization: Using Django's ORM efficiently, optimizing queries, and using database indexing.
- Caching: Implementing caching strategies to improve performance.
- Scalability: Strategies to scale Django applications, including load balancing and database replication.
13. Internationalization (i18n) and Localization (l10n)
- Translating Applications: Making your application available in multiple languages.
- Timezone Support: Managing timezones within your Django application.
14. Django Signals
- Using Signals: Understanding and implementing Django signals to decouple components of your application.
15. Asynchronous Support
- Async Views: Writing asynchronous views in Django.
- Channels: Using Django Channels for WebSocket support and handling background tasks.
16. File Uploads and Management
- Handling File Uploads: Managing file uploads in Django and processing uploaded files.
- Storage Options: Using different storage backends (e.g., AWS S3) for media files.
Mastering these topics will provide a solid foundation in Django, enabling you to build complex and scalable web applications.
The above is the detailed content of Key Topics While Learning Django. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










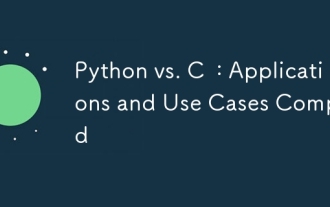
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
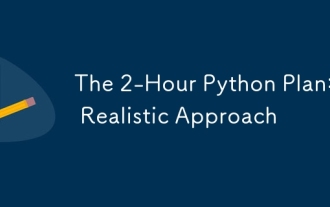
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
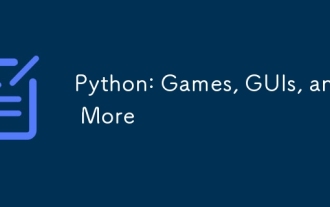
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
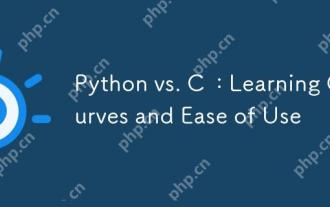
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
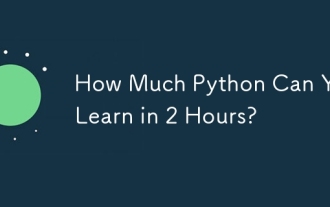
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
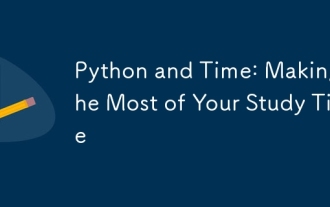
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
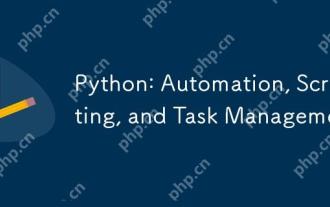
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
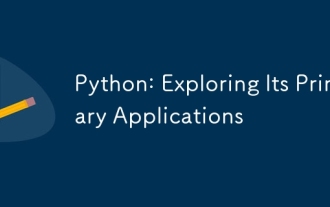
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
