Factorial in C#
In this section, we shall see the factorial in c# in detail. Factorial is a very important concept in the area of mathematics like in algebra or in mathematics analytics. It is denoted by sign of exclamation (!). Factorial is any positive integer k, which is denoted by k! It is the product of all positive integers which are less than or equal to k.
k!= k * (k-1) *(k-2) *(k-3) *(k-4) *…….3 *2 * 1.
Logic to Calculate Factorial of A Given Number
For example, if we want to calculate the factorial of 4 then it would be,
Example #1
4! = 4 * (4-1) *(4-2) * (4-3)
4! = 4 * 3 * 2 * 1
4! = 24.
So factorial of 4 is 24
Example #2
6! = 6 * (6-1)* (6-2)* (6-3) * 6-4)* (6-5)
6! = 6*5*4*3*2*1
6! = 720
So factorial of 6 is 720
Similarly, by using this technique we can calculate the factorial of any positive integer. The important point here is that the factorial of 0 is 1.
0! =1.
There are many explanations for this like for n! where n=0 signifies product of no numbers and it is equal to the multiplicative entity. {displaystyle {binom {0}{0}}={frac {0!}{0!0!}}=1.}
The factorial function is mostly used to calculate the permutations and combinations and also used in binomial. With the help of the factorial function, we can also calculate the probability. For example in how many ways we can arrange k items. We have k choices for the first thing, So for each of these k choices, we left with k-1 choices for the second things (because first choice has already been made), so that now we have k(k-1) choices, so now for the third choice we have k(k-1)(k-2) choices and so on until we get one on thing is remaining. So altogether we will have k(k-1)(k-2)(k-3)…3..1.
Another real-time example is supposed we are going to a wedding and we want to choose which blazer to take. So let’s suppose we have k blazers and but have room to pack the only n. So how many ways we can use n blazers from a collection of k blazers k!/(n!.(k-n)!).
Examples of Factorial in C#
Below are the examples to show how we can calculate factorial of any number in different ways,
Example #1
1. In these examples, for loop is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Factorial { class Program { static void Main() { int a = 7; int fact = 1; for (int x = 1; x <= a; x++) { fact *= x; } Console.WriteLine(fact); Console.ReadLine(); } } }
In this example, the variable of integer data type is initialized and for loop is used to calculate the number.
Output:
2. In this example, the user is allowed to enter the number to calculate the factorial.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace FactorialExample { class Program { static void Main() { Console.WriteLine("Enter the number: "); int a = int.Parse(Console.ReadLine()); int fact = 1; for (int x = 1; x <= a; x++) { fact *= x; } Console.WriteLine(fact); Console.ReadLine(); } } }
Output:
Example #2
1. In these examples, for loop is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Factorial { class Program { static void Main() { int a = 10; int fact = 1; while (true) { Console.Write(a); if (a == 1) { break; } Console.Write("*"); fact *= a; a--; } Console.WriteLine(" = {0}", fact); Console.ReadLine(); } } }
Output:
2. In these examples, while loop is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace FactorialExample { class Program { static void Main() { Console.WriteLine("Enter the number: "); int a = int.Parse(Console.ReadLine()); int fact = 1; while(true) { Console.Write(a); if(a==1) { break; } Console.Write("*"); fact *= a; a--; } Console.WriteLine(" = {0}", fact); Console.ReadLine(); } } }
Output:
Example #3
1. In this example, do-while is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Factorial { class Program { static void Main() { int a = 6; int fact = 1; do { fact *= a; a--; } while (a > 0); Console.WriteLine("Factorial = {0}", fact); Console.ReadLine(); } } }
Output:
2. In this example, do-while is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace FactorialExample { class Program { static void Main() { Console.Write("Enter the number: "); int a = int.Parse(Console.ReadLine()); int fact = 1; do { fact *= a; a--; } while (a > 0); Console.WriteLine("Factorial = {0}", fact); Console.ReadLine(); } } }
Output:
Example #4
1. In this example, a recursive function is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Factorial { class Program { static void Main() { int n= 5; long fact = Fact(n); Console.WriteLine("factorial is {1}", n, fact); Console.ReadKey(); } private static long Fact(int n) { if (n == 0) { return 1; } return n * Fact(n - 1); } } }
In the above example, the factorial of a number is achieved by using recursion. The idea behind the recursion is to solve the problem in small instances. So whenever a function creating a loop and calling itself, it’s called recursion.
Output:
2. In this example, a recursive function is used to calculate the factorial of a number.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace FactorialExample { class Program { static void Main() { Console.WriteLine("Enter the number"); int n = Convert.ToInt32(Console.ReadLine()); long fact = Fact(n); Console.WriteLine("factorial is {1}", n, fact); Console.ReadKey(); } private static long Fact(int n) { if (n == 0) { return 1; } return n * Fact(n - 1); } } }
Output:
Conclusion
So the concept of factorial is very important in areas of mathematics such as binomials and permutations and combinations, and this is how we can print the factorial of any number by using multiple methods such as for, while, do-while, function, etc.
The above is the detailed content of Factorial in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


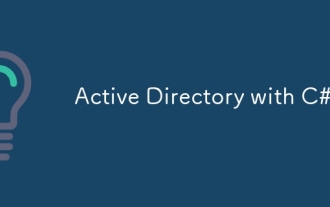
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
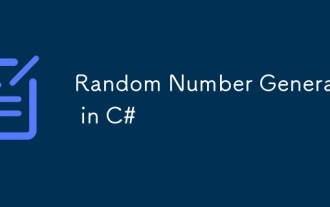
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
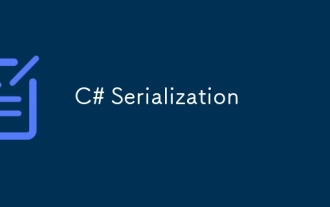
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
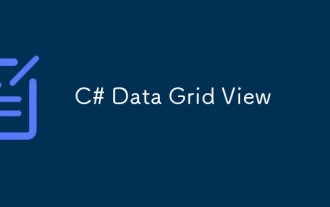
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
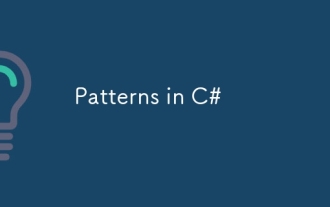
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
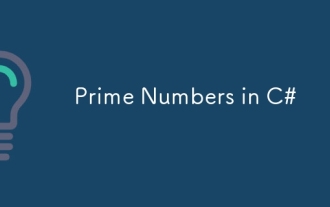
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
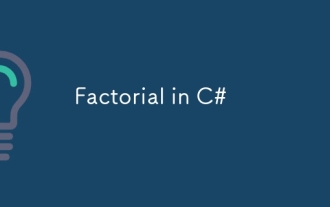
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
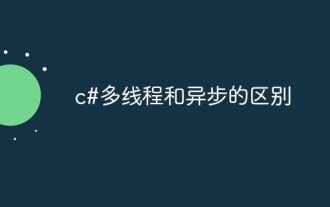
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
