Day Handling Events in React
Welcome to Day 6 of our "30 Days of ReactJS" series! Today, we’re diving into Handling Events in React. Understanding event handling is crucial for creating interactive and user-friendly applications.
What is Event Handling?
Event handling in React allows you to respond to user actions such as clicks, form submissions, or keyboard inputs. In React, events are handled in a way that is similar to how you would handle them in plain HTML/JavaScript, but with some key differences that fit within React’s declarative model.
React Event Handling Basics
In React, event handlers are passed as props to React elements. Unlike plain HTML, React event handlers use camelCase syntax instead of lowercase. For example, onClick instead of onclick.
Example: Basic Button Click Handler
import React from 'react'; function ClickButton() { const handleClick = () => { alert('Button was clicked!'); }; return ( <button onClick={handleClick}> Click Me </button> ); } export default ClickButton;
In this example, the handleClick function is executed when the button is clicked, displaying an alert.
Real-Life Example: ATM Machine
Imagine an ATM machine where you enter your PIN and select an amount to withdraw. Each button press (like entering a digit or selecting the withdrawal amount) triggers an event. In React, you handle these interactions using event handlers.
Event Objects
React event handlers receive an event object as an argument. This object contains information about the event, such as the target element and the type of event.
Example: Handling Input Change
import React, { useState } from 'react'; function InputForm() { const [value, setValue] = useState(''); const handleChange = (event) => { setValue(event.target.value); }; return ( <div> <input type="text" value={value} onChange={handleChange} /> <p>You typed: {value}</p> </div> ); } export default InputForm;
Here, the handleChange function updates the state with the input value, allowing you to see what is typed in real-time.
Binding Event Handlers
In class components, you often need to bind event handlers to the component instance. This is not necessary in functional components with hooks, as functions are automatically bound.
Example: Binding in Class Components
class MyComponent extends React.Component { constructor(props) { super(props); this.handleClick = this.handleClick.bind(this); } handleClick() { console.log('Button clicked'); } render() { return ( <button onClick={this.handleClick}> Click Me </button> ); } }
Binding ensures that this refers to the component instance inside the event handler.
Event Handling with Vite
With Vite as your development tool, handling events remains straightforward. Vite’s fast refresh helps you see changes immediately, making it easier to test and debug your event handlers.
Wrapping Up
Handling events is a fundamental aspect of React that allows your application to respond to user interactions. By attaching event handlers to elements, you can make your app dynamic and interactive.
Tomorrow, we’ll build upon this by exploring Building Your First ReactJS App, where you’ll see how event handling integrates into a complete application.
The above is the detailed content of Day Handling Events in React. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










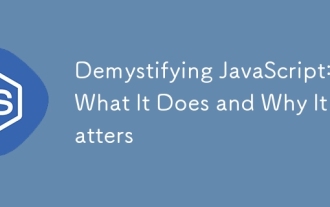
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
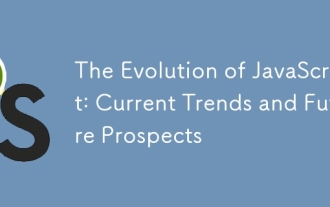
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
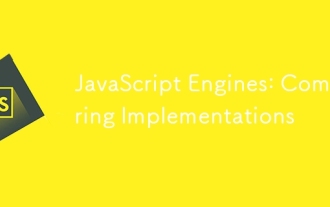
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
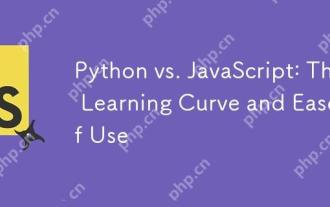
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
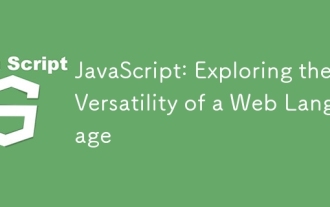
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
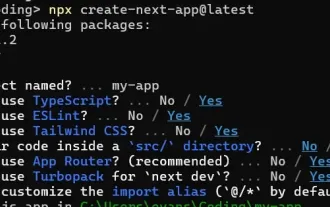
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
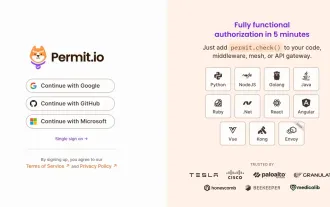
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
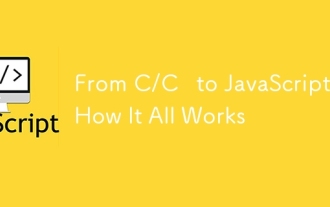
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
