Handling Multithreading in Java with Completable Future
1. Understanding Completable Future
CompletableFuture is part of the java.util.concurrent package and offers a way to write asynchronous, non-blocking code in a more readable and maintainable manner. It represents a future result of an asynchronous computation.
1.1 Creating a Simple CompletableFuture
To start with CompletableFuture , you can create a simple asynchronous task. Here’s an example:
import java.util.concurrent.CompletableFuture; public class CompletableFutureExample { public static void main(String[] args) { CompletableFuture<Void> future = CompletableFuture.runAsync(() -> { System.out.println("Running asynchronously..."); // Simulate a long-running task try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } }); future.join(); // Wait for the task to complete System.out.println("Task completed."); } }
- CompletableFuture.runAsync() executes a task asynchronously.
- future.join() blocks the main thread until the task is finished.
Demo Result:
Running asynchronously... Task completed.
1.2 Using CompletableFuture with Results
You can also use CompletableFuture to return results from asynchronous tasks:
import java.util.concurrent.CompletableFuture; public class CompletableFutureWithResult { public static void main(String[] args) { CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> { // Simulate a computation return 5 * 5; }); future.thenAccept(result -> { System.out.println("The result is: " + result); }).join(); } }
- CompletableFuture.supplyAsync() is used for tasks that return a result.
- thenAccept() processes the result once the computation is complete.
Demo Result:
The result is: 25
2. Combining Multiple CompletableFutures
Handling multiple asynchronous tasks is a common use case. CompletableFuture provides several methods to combine futures.
2.1 Combining Futures with thenCombine
You can combine results from multiple CompletableFutures :
import java.util.concurrent.CompletableFuture; public class CombiningFutures { public static void main(String[] args) { CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> 5); CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> 10); CompletableFuture<Integer> combinedFuture = future1.thenCombine(future2, (result1, result2) -> result1 + result2); combinedFuture.thenAccept(result -> { System.out.println("Combined result: " + result); }).join(); } }
- thenCombine () combines the results of two futures.
- The combined result is processed using thenAccept ().
Demo Result:
Combined result: 15
2.2 Handling Multiple Futures with allOf
When you need to wait for multiple futures to complete, use CompletableFuture.allOf():
import java.util.concurrent.CompletableFuture; public class AllOfExample { public static void main(String[] args) { CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> { // Simulate task try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } }); CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> { // Simulate another task try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } }); CompletableFuture<Void> allOfFuture = CompletableFuture.allOf(future1, future2); allOfFuture.join(); System.out.println("All tasks completed."); } }
- CompletableFuture.allOf() waits for all given futures to complete.
- join() ensures the main thread waits until all tasks are done.
Demo Result:
All tasks completed.
3. Error Handling with CompletableFuture
Handling errors is essential in asynchronous programming. CompletableFuture provides methods to manage exceptions.
3.1 Handling Exceptions with exceptionally
Use exceptionally () to handle exceptions in asynchronous tasks:
import java.util.concurrent.CompletableFuture; public class ExceptionHandlingExample { public static void main(String[] args) { CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> { throw new RuntimeException("Something went wrong!"); }).exceptionally(ex -> { System.out.println("Exception occurred: " + ex.getMessage()); return null; }); future.join(); } }
- exceptionally () catches and handles exceptions.
- It allows you to provide a fallback result or handle the error.
Demo Result:
Exception occurred: Something went wrong!
4. Advantages and Disadvantages of CompletableFuture
4.1 Advantages
- Asynchronous Execution : Efficiently handles tasks that run concurrently without blocking the main thread.
- Improved Readability : Provides a more readable and maintainable way to handle asynchronous code compared to traditional callback approaches.
- Rich API : Offers a variety of methods to combine, handle, and compose multiple futures.
4.2 Disadvantages
- Complexity : While powerful, CompletableFuture can introduce complexity in managing and debugging asynchronous code.
- Exception Handling: Handling exceptions can sometimes be tricky, especially in complex scenarios with multiple stages.
5. Conclusion
In this guide, we've explored how CompletableFuture can be used to handle concurrent requests in Java. From creating simple asynchronous tasks to combining multiple futures and handling errors, CompletableFuture provides a robust and flexible approach to asynchronous programming.
If you have any questions or need further assistance, feel free to leave a comment below. I’d be happy to help!
Read posts more at : Handling Multithreading in Java with Completable Future
The above is the detailed content of Handling Multithreading in Java with Completable Future. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










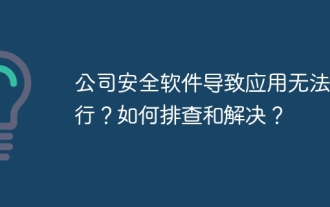
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
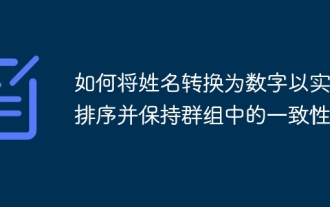
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
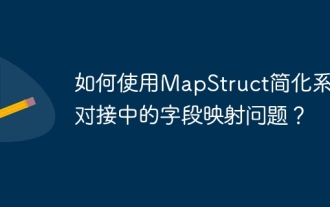
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
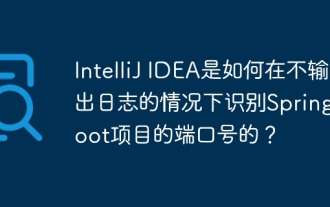
Start Spring using IntelliJIDEAUltimate version...
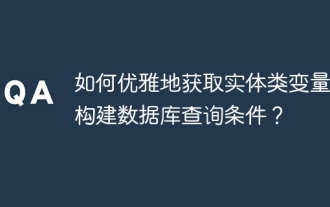
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
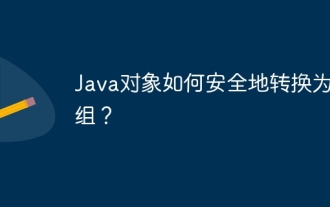
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
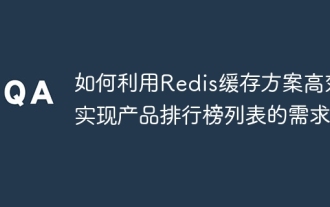
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
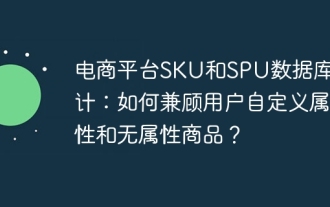
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
