


Object-Oriented Programming (OOP) in Python: Classes and Objects Explained
Object-Oriented Programming (OOP) is a key approach used in software development.
In this article, we'll explore the main ideas of OOP, particularly looking at classes, objects, inheritance, and polymorphism in Python.
By the end of this guide, you'll understand how to organize your Python code using OOP principles, making your programs more modular, reusable, and easier to maintain.
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) organizes software design around data, or objects, rather than functions and logic.
An object is like a container with unique attributes (data) and behaviors (functions). OOP focuses on several key concepts:
Encapsulation
This means bundling the data (attributes) and the methods (functions) that operate on that data into a single unit, called a class.
It also involves restricting access to some components of the object, making it more secure.
Abstraction
This is the idea of hiding the complex implementation details and showing only the essential features of the object.
It reduces complexity and allows the programmer to focus on higher-level interactions.
Inheritance
This is a mechanism for creating a new class (derived class) from an existing class (base class).
The new class inherits attributes and methods from the existing class.
Polymorphism
This is the ability to use a single interface to represent different data types.
It allows objects to be treated as instances of their parent class and makes it possible to define methods in a child class that have the same name as a method in the parent class.
OOP Basics in Python: Classes and Objects
At the core of Object-Oriented Programming (OOP) in Python are classes and objects.
Classes
A class is like a blueprint for creating objects.
It defines a set of properties (attributes) and actions (methods) that the objects will have.
In Python, you create a class using the class keyword. Here's an example:
class Car: def __init__(self, make, model, year): self.make = make self.model = model self.year = year def start_engine(self): print(f"{self.make} {self.model}'s engine started.")
Objects
An object is an instance of a class.
Once you define a class, you can create multiple objects (instances) from it.
Each object can have its own unique values for the attributes defined in the class.
Here's how you create and use an object:
my_car = Car("Toyota", "Corolla", 2020) my_car.start_engine() # Output: Toyota Corolla's engine started.
In this example, my_car is an object of the Car class.
It has its own values for make, model, and year, and you can use methods like start_engine.
Inheritance in Python
Inheritance lets one class (the child class) take on the attributes and methods of another class (the parent class).
This is great for reusing code and setting up a hierarchy between classes.
Here's an example:
class Vehicle: def __init__(self, make, model): self.make = make self.model = model def drive(self): print("Driving...") class Car(Vehicle): def __init__(self, make, model, year): super().__init__(make, model) self.year = year def start_engine(self): print(f"{self.make} {self.model}'s engine started.") my_car = Car("Honda", "Civic", 2021) my_car.drive() # Output: Driving... my_car.start_engine() # Output: Honda Civic's engine started.
In this example, the Car class inherits from the Vehicle class.
Because of this, the Car class can use the drive method that's defined in the Vehicle class.
Method Overriding
Sometimes, a child class needs to change or add to the behavior of a method it inherits from a parent class.
This is done through method overriding.
Here's an example:
class Vehicle: def drive(self): print("Driving a vehicle...") class Car(Vehicle): def drive(self): print("Driving a car...") my_vehicle = Vehicle() my_vehicle.drive() # Output: Driving a vehicle... my_car = Car() my_car.drive() # Output: Driving a car...
In this example, the drive method in the Car class overrides the drive method in the Vehicle class, allowing for customized behavior.
Multiple Inheritance
Python also supports multiple inheritance, where a class can inherit from more than one base class.
Here's an example:
class Vehicle: def __init__(self, make, model): self.make = make self.model = model def drive(self): print("Driving a vehicle...") class Electric: def charge(self): print("Charging...") class Car(Vehicle): def __init__(self, make, model, year): super().__init__(make, model) self.year = year def start_engine(self): print(f"{self.make} {self.model}'s engine started.") class HybridCar(Car, Electric): def switch_mode(self): print("Switching to electric mode...") my_hybrid = HybridCar("Toyota", "Prius", 2022) my_hybrid.start_engine() # Output: Toyota Prius's engine started. my_hybrid.drive() # Output: Driving a vehicle... my_hybrid.charge() # Output: Charging... my_hybrid.switch_mode() # Output: Switching to electric mode...
In this example, the HybridCar class inherits from both Car and Electric, allowing it to access methods from both parent classes.
Polymorphism in Python
Polymorphism is a feature that allows methods to perform different actions based on the object they are working with, even if these methods have the same name.
This is especially useful when dealing with inheritance, as it lets you use the same method name across different classes in a way that makes sense for each class.
Polymorphism with Functions
Here's an example:
class Dog: def speak(self): return "Woof!" class Cat: def speak(self): return "Meow!" def make_animal_speak(animal): print(animal.speak()) dog = Dog() cat = Cat() make_animal_speak(dog) # Output: Woof! make_animal_speak(cat) # Output: Meow!
The make_animal_speak function demonstrates polymorphism by accepting any object with a speak method.
This allows it to work with both Dog and Cat objects, despite their differences.
Polymorphism with Class Methods
Polymorphism also comes into play when working with methods in a class hierarchy.
Here's an example:
class Animal: def speak(self): raise NotImplementedError("Subclass must implement abstract method") class Dog(Animal): def speak(self): return "Woof!" class Cat(Animal): def speak(self): return "Meow!" animals = [Dog(), Cat()] for animal in animals: print(animal.speak())
In this example, both Dog and Cat are subclasses of Animal.
The speak method is implemented in both subclasses, allowing polymorphism to take effect when iterating through the list of animals.
Encapsulation and Data Hiding
Encapsulation is the practice of combining data and the methods that work on that data into a single unit, called a class.
It also involves restricting access to certain parts of the object, which is crucial for protecting data in Object-Oriented Programming (OOP).
Private and Public Attributes
In Python, you can indicate that an attribute is private by starting its name with an underscore.
While this doesn't actually prevent access from outside the class, it's a convention that signals that the attribute should not be accessed directly.
Here's an example:
class Account: def __init__(self, owner, balance=0): self.owner = owner self._balance = balance # Private attribute def deposit(self, amount): self._balance += amount def withdraw(self, amount): if amount <= self._balance: self._balance -= amount else: print("Insufficient funds") def get_balance(self): return self._balance my_account = Account("John", 1000) my_account.deposit(500) print(my_account.get_balance()) # Output: 1500
In this example, the Account class has a private attribute _balance, which is manipulated through methods like deposit, withdraw, and get_balance.
Direct access to _balance from outside the class is discouraged.
Advanced OOP Concepts
For those who want to deepen their understanding of Object-Oriented Programming (OOP) in Python, here are a few advanced topics:
Class Methods
These are methods that are connected to the class itself, not to individual instances of the class.
They can change the state of the class, which affects all instances of the class.
class Car: total_cars = 0 def __init__(self, make, model): self.make = make self.model = model Car.total_cars += 1 @classmethod def get_total_cars(cls): return cls.total_cars
Static Methods
These are methods that belong to the class but do not change the state of the class or its instances.
They are defined using the @staticmethod decorator.
class MathOperations: @staticmethod def add(x, y): return x + y
Property Decorators
Property decorators in Python provide a way to define getters, setters, and deleters for class attributes in a more Pythonic manner.
class Employee: def __init__(self, name, salary): self._name = name self._salary = salary @property def salary(self): return self._salary @salary.setter def salary(self, value): if value < 0: raise ValueError("Salary cannot be negative") self._salary = value
In this example, the salary attribute is accessed like a regular attribute but is managed by getter and setter methods.
Conclusion
Object-Oriented Programming (OOP) in Python is a powerful way to organize and manage your code.
By learning the principles of OOP, such as classes, objects, inheritance, polymorphism, and encapsulation, you can write Python programs that are well-organized, reusable, and easy to maintain.
Whether you're working on small scripts or large applications, using OOP principles will help you create more efficient, scalable, and robust software.
The above is the detailed content of Object-Oriented Programming (OOP) in Python: Classes and Objects Explained. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










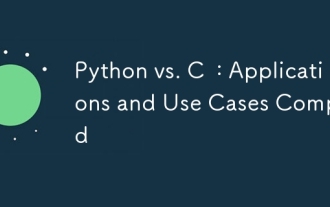
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
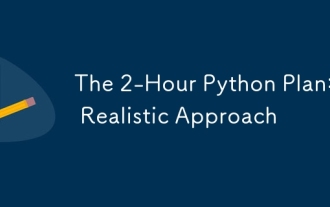
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
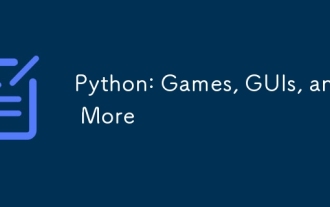
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
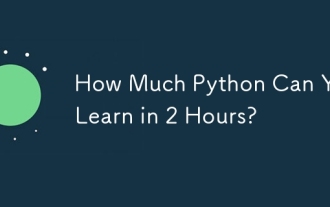
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
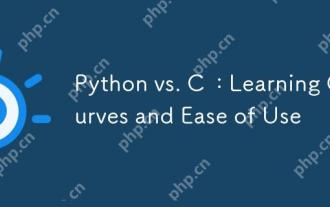
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
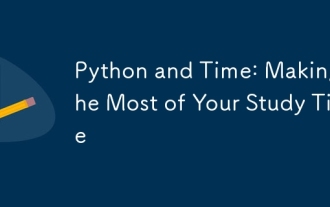
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
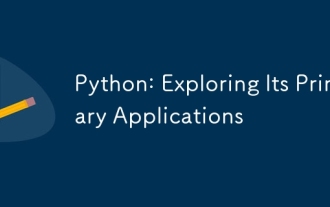
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
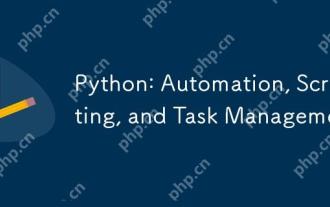
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
