UseState and UseEffect Hook in React
In React, useState and useEffect are two fundamental hooks used to manage state and handle side effects in functional components.
1. useState Hook
The useState hook allows you to add state to functional components. It returns an array with two elements:
- The current state value.
- A function to update that state value.
Example:
import React, { useState } from 'react'; function Counter() { // Declare a state variable called 'count' with an initial value of 0 const [count, setCount] = useState(0); return ( <div> <p>You clicked {count} times</p> {/* Update state using the setCount function */} <button onClick={() => setCount(count + 1)}>Click me</button> </div> ); } export default Counter;
In this example:
- useState(0) initializes the count state variable with a value of 0.
- setCount is used to update the state when the button is clicked.
2. useEffect Hook
The useEffect hook allows you to perform side effects in your components, such as data fetching, subscriptions, or manually changing the DOM. It takes two arguments:
- A function that contains the side effect logic.
- An optional array of dependencies that determines when the effect should run.
Example:
import React, { useState, useEffect } from 'react'; function Example() { const [count, setCount] = useState(0); // useEffect runs after every render, but the dependency array makes it run only when `count` changes useEffect(() => { document.title = `You clicked ${count} times`; // Cleanup function (optional) return () => { console.log('Cleanup for count:', count); }; }, [count]); // Dependency array return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}>Click me</button> </div> ); } export default Example;
In this example:
- useEffect updates the document title whenever count changes.
- The effect runs after every render if count changes, because count is included in the dependency array.
- The optional cleanup function runs before the effect runs again or when the component unmounts, which is useful for cleaning up subscriptions or timers.
Both hooks help manage state and side effects in functional components effectively, making React development more concise and powerful.
.
.
.
Let's Summaries....
.
.
.
Here’s a summary of the useState and useEffect hooks in React:
useState Hook
- Purpose: Manages state in functional components.
- Syntax: const [state, setState] = useState(initialValue);
-
Parameters:
- initialValue: The initial state value.
-
Returns:
- An array with two elements:
- state: The current state value.
- setState: Function to update the state.
Example Usage:
const [count, setCount] = useState(0);
useEffect Hook
- Purpose: Handles side effects in functional components, such as data fetching, subscriptions, or manual DOM updates.
- Syntax: useEffect(() => { /* effect logic */ }, [dependencies]);
-
Parameters:
- Effect Function: Contains the code to run as a side effect.
- Dependency Array (optional): List of dependencies that trigger the effect when changed. If empty, the effect runs only once after the initial render. If omitted, the effect runs after every render.
- Cleanup Function (optional): Returned function from the effect function to clean up resources.
Example Usage:
useEffect(() => { document.title = `You clicked ${count} times`; return () => { // Cleanup logic here }; }, [count]);
Key Points:
- useState simplifies state management in functional components.
- useEffect handles side effects and can optionally clean up after itself.
- Both hooks work together to create dynamic, stateful functional components in React.
The above is the detailed content of UseState and UseEffect Hook in React. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










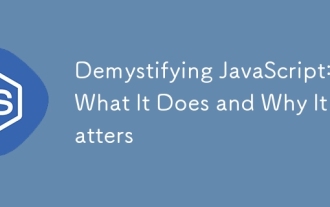
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
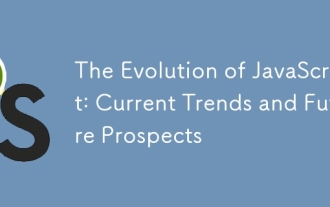
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
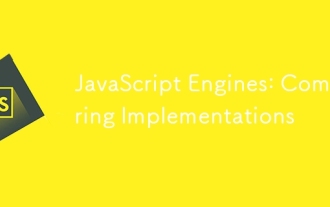
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
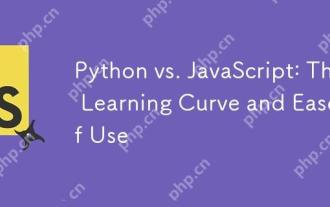
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
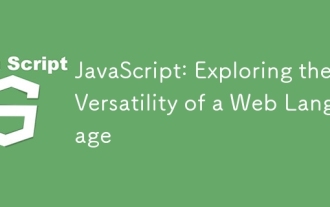
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
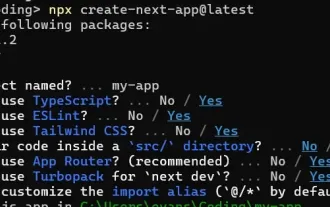
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
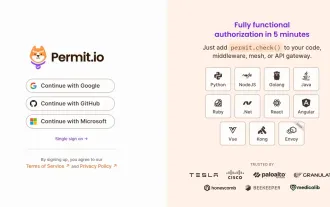
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
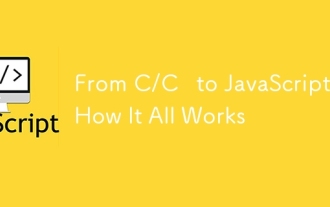
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
