ReactJS Design Patterns: Writing Robust and Scalable Components
Design patterns in ReactJS provide standardized and proven solutions to common problems in application development. Using these patterns not only makes your code more readable and maintainable but also enhances its scalability and robustness. Let's dive into some of the most popular ReactJS design patterns, with examples to illustrate their usage.
1. Container and Presentational Components Pattern
The Container and Presentational pattern separates components into two categories:
- Presentational Components: Focus on how things look (UI).
- Container Components: Focus on how things work (logic and state management).
This separation allows for better reusability, easier testing, and cleaner code.
Example: Presentational and Container Components
// Presentational Component: Displaying User List (UserList.js) import React from 'react'; const UserList = ({ users }) => ( <ul> {users.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> ); export default UserList;
// Container Component: Fetching User Data (UserContainer.js) import React, { useState, useEffect } from 'react'; import UserList from './UserList'; const UserContainer = () => { const [users, setUsers] = useState([]); useEffect(() => { const fetchUsers = async () => { const response = await fetch('https://jsonplaceholder.typicode.com/users'); const data = await response.json(); setUsers(data); }; fetchUsers(); }, []); return <UserList users={users} />; }; export default UserContainer;
Here, UserList is a presentational component that receives users as props, while UserContainer handles data fetching and state management.
2. Higher-Order Components (HOC) Pattern
A Higher-Order Component (HOC) is a function that takes a component as an argument and returns a new component. HOCs are commonly used for cross-cutting concerns like authentication, logging, or enhancing component behavior.
Example: Creating an HOC for Authorization
// withAuthorization.js (HOC for Authorization) import React from 'react'; const withAuthorization = (WrappedComponent) => { return class extends React.Component { componentDidMount() { if (!localStorage.getItem('authToken')) { // Redirect to login if not authenticated window.location.href = '/login'; } } render() { return <WrappedComponent {...this.props} />; } }; }; export default withAuthorization;
// Dashboard.js (Component Wrapped with HOC) import React from 'react'; import withAuthorization from './withAuthorization'; const Dashboard = () => <h1>Welcome to the Dashboard</h1>; export default withAuthorization(Dashboard);
By wrapping Dashboard with withAuthorization, you ensure that only authenticated users can access it.
3. Render Props Pattern
The Render Props pattern involves sharing code between components using a prop whose value is a function. This pattern is useful for dynamic rendering based on certain conditions or states.
Example: Using Render Props for Mouse Tracking
// MouseTracker.js (Component with Render Props) import React, { useState } from 'react'; const MouseTracker = ({ render }) => { const [position, setPosition] = useState({ x: 0, y: 0 }); const handleMouseMove = (event) => { setPosition({ x: event.clientX, y: event.clientY }); }; return <div onMouseMove={handleMouseMove}>{render(position)}</div>; }; export default MouseTracker;
// App.js (Using Render Props) import React from 'react'; import MouseTracker from './MouseTracker'; const App = () => ( <MouseTracker render={({ x, y }) => ( <h1> Mouse position: ({x}, {y}) </h1> )} /> ); export default App;
The MouseTracker component uses a render prop to pass mouse position data to any component, making it highly reusable.
4. Custom Hooks Pattern
Custom Hooks allow you to encapsulate and reuse stateful logic across multiple components. This pattern promotes code reusability and clean separation of concerns.
Example: Creating a Custom Hook for Fetching Data
// useFetch.js (Custom Hook) import { useState, useEffect } from 'react'; const useFetch = (url) => { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); useEffect(() => { const fetchData = async () => { const response = await fetch(url); const result = await response.json(); setData(result); setLoading(false); }; fetchData(); }, [url]); return { data, loading }; }; export default useFetch;
// App.js (Using the Custom Hook) import React from 'react'; import useFetch from './useFetch'; const App = () => { const { data, loading } = useFetch('https://jsonplaceholder.typicode.com/posts'); if (loading) return <div>Loading...</div>; return ( <ul> {data.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }; export default App;
The useFetch custom hook encapsulates the data fetching logic, which can be reused across different components.
5. Compound Components Pattern
The Compound Components pattern allows components to work together to manage state and behavior. This pattern is useful for building complex UI components like tabs, accordions, or dropdowns.
Example: Building Tabs with Compound Components
// Tabs.js (Parent Component) import React, { useState } from 'react'; const Tabs = ({ children }) => { const [activeIndex, setActiveIndex] = useState(0); return React.Children.map(children, (child, index) => React.cloneElement(child, { isActive: index === activeIndex, setActiveIndex, index }) ); }; const Tab = ({ children, isActive, setActiveIndex, index }) => ( <button onClick={() => setActiveIndex(index)}>{children}</button> ); const TabPanel = ({ children, isActive }) => (isActive ? <div>{children}</div> : null); Tabs.Tab = Tab; Tabs.TabPanel = TabPanel; export default Tabs;
// App.js (Using Compound Components) import React from 'react'; import Tabs from './Tabs'; const App = () => ( <Tabs> <Tabs.Tab>Tab 1</Tabs.Tab> <Tabs.Tab>Tab 2</Tabs.Tab> <Tabs.TabPanel>Content for Tab 1</Tabs.TabPanel> <Tabs.TabPanel>Content for Tab 2</Tabs.TabPanel> </Tabs> ); export default App;
The Tabs component manages state, while Tab and TabPanel components work together to display the tabbed content.
6. Controlled and Uncontrolled Components Pattern
Controlled components are fully managed by React state, while uncontrolled components rely on the DOM for their state. Both have their uses, but controlled components are generally preferred for consistency and maintainability.
Example: Controlled vs. Uncontrolled Components
// Controlled Component (TextInputControlled.js) import React, { useState } from 'react'; const TextInputControlled = () => { const [value, setValue] = useState(''); return ( <input type="text" value={value} onChange={(e) => setValue(e.target.value)} /> ); }; export default TextInputControlled;
// Uncontrolled Component (TextInputUncontrolled.js) import React, { useRef } from 'react'; const TextInputUncontrolled = () => { const inputRef = useRef(); const handleClick = () => { console.log(inputRef.current.value); }; return ( <> <input type="text" ref={inputRef} /> <button onClick={handleClick}>Log Input Value</button> </> ); }; export default TextInputUncontrolled;
In controlled components, React fully controls the form state, while in uncontrolled components, the state is managed by the DOM itself.
7. Hooks Factory Pattern
The Hooks Factory Pattern involves creating hooks that dynamically generate and manage multiple states or behaviors, providing a flexible way to manage complex logic.
Example: Dynamic State Management with Hooks Factory
// useDynamicState.js (Hook Factory) import { useState } from 'react'; const useDynamicState = (initialStates) => { const states = {}; const setters = {}; initialStates.forEach(([key, initialValue]) => { const [state, setState] = useState(initialValue); states[key] = state; setters[key] = setState; }); return [states, setters]; }; export default useDynamicState;
// App.js (Using the Hooks Factory) import React from 'react'; import useDynamicState from './useDynamicState'; const App = () => { const [states, setters] = useDynamicState([ ['name', ''], ['age', 0], ]); return ( <div> <input type="text" value={states.name} onChange={(e) => setters .name(e.target.value)} /> <input type="number" value={states.age} onChange={(e) => setters.age(parseInt(e.target.value))} /> <p>Name: {states.name}</p> <p>Age: {states.age}</p> </div> ); }; export default App;
This hook factory dynamically creates and manages multiple states, providing flexibility and cleaner code.
Conclusion
By leveraging these design patterns, you can create React applications that are more robust, scalable, and maintainable. These patterns help you write clean, reusable code that adheres to best practices, ensuring your application is easier to develop and manage over time.
Would you like to dive deeper into any of these patterns or explore other topics?
The above is the detailed content of ReactJS Design Patterns: Writing Robust and Scalable Components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










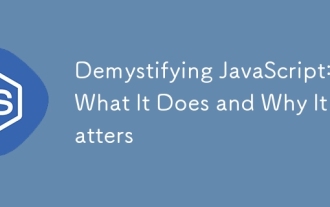
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
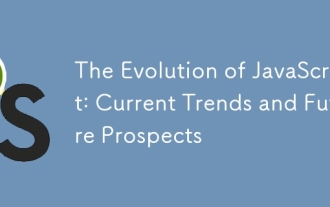
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
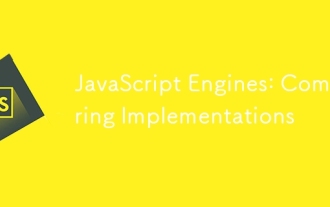
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
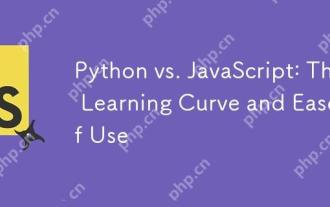
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
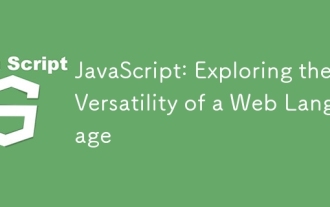
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
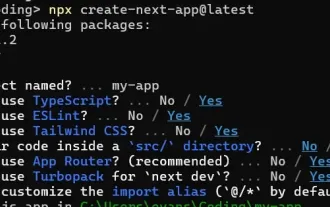
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
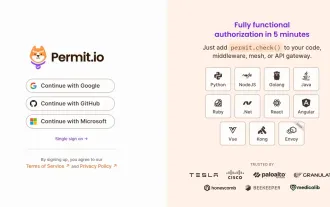
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
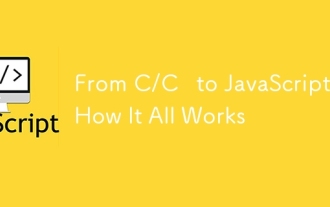
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
