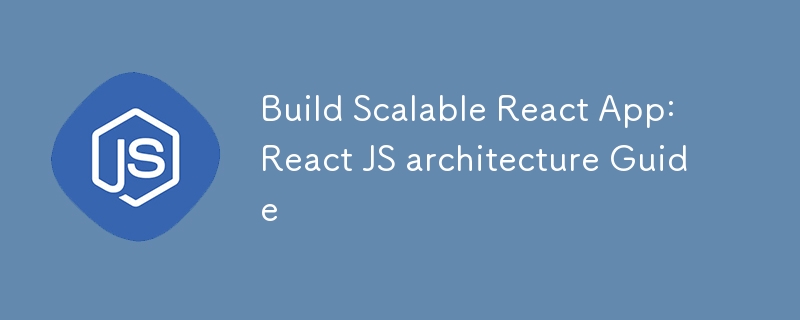
React.js has become one of the most popular JavaScript libraries for building user interfaces, with over 8 million weekly downloads on npm. As React applications grow in size and complexity, ensuring scalability and performance becomes critical to delivering a seamless user experience. Scalable React applications can handle increasing amounts of traffic and data without compromising speed or responsiveness. In this comprehensive guide, we will explore the best practices and architectural patterns that enable React applications to scale effectively.
Understanding Scalable React App Architecture
What is React JS Architecture?
React JS architecture structures components and manages data flow within a React application. It leverages a component-based system, allowing developers to build reusable UI elements. Core features include the virtual DOM for optimized rendering and various state management tools like Context API and Redux to maintain consistent data flow.
How React JS Architecture Supports Scalability
Scalability in React architecture is achieved through:
-
Component Reusability: Modular components reduce code duplication and simplify application maintenance.
-
State Management: Tools like Redux and Context API streamline data management, ensuring smooth updates across components.
-
Separation of Concerns: Dividing logic and presentation improves code organization and enables independent development of different parts.
-
Lazy Loading and Code Splitting: These techniques load only necessary components, improving performance and scalability as the application grows.
These elements contribute to creating a scalable React app that can handle increased complexity while maintaining performance.
Why Choosing the Right Architecture Matters
Choosing the right React JS architecture is essential for:
-
Maintainability: A clear architecture simplifies updates and modifications.
-
Performance: Optimized architecture ensures responsive performance, even in large apps.
-
Collaboration: Well-structured code enhances teamwork and efficiency.
-
Scalability: It supports new features and scaling as the app grows.
The right architecture is key to architecting React applications that are scalable, efficient, and future-proof, ensuring long-term success.
Key Principles for Scalable React Design
What Are Scalable React Design Principles?
Scalable React design principles provide a roadmap for building applications that can grow in complexity and user base while maintaining performance. Key principles include:
-
Separation of Concerns: Distinguish between UI components and business logic to improve modularity.
-
Component Reusability: Create reusable components to reduce redundancy and simplify updates.
-
State Management: Use Redux or Context API to ensure consistent and predictable state management.
-
Performance Optimization: Implement code splitting and lazy loading to boost load times and scalability.
-
Loose Coupling: Ensure components are independent to allow for easier updates and maintenance.
When to Adopt Scalable React Strategies
Adopt scalable React design strategies at different stages:
-
From the Start: Begin with a scalable React app architecture to avoid future refactoring.
-
Adding Features: Align new features with scalable React design principles.
-
Performance Optimization: Address bottlenecks by revisiting design strategies.
-
User Growth: Reassess architecture as user demand increases to maintain performance.
By applying these principles, you’ll ensure your React JS architecture is scalable, maintainable, and ready for future growth.
Architecting React Applications: Best Practices
What Are the Best Practices for Architecting React applications?
Architecting scalable and maintainable React applications requires following key best practices:
-
Modular Component Design: Create reusable, self-contained components that encapsulate specific functionality and UI elements.
-
Separation of Concerns: Clearly separate presentation logic (UI components) from business logic (data handling) to enhance modularity and maintainability.
-
Centralized State Management: Use state management solutions like Redux or Context API to maintain a consistent and predictable application state.
-
Performance Optimization: Implement techniques like code splitting and lazy loading to improve load times and responsiveness as the application scales.
-
Automated Testing: Establish a robust testing framework to ensure components function correctly and maintain performance as the application evolves.
How to Implement These Practices Effectively
To effectively implement these best practices:
- Plan a modular architecture from the outset, defining clear folder structure and choosing appropriate state management solutions.
- Leverage design patterns like Container/Presentational components and Higher-Order Components (HOCs) to separate concerns and enable reusability.
- Utilize tools like Redux or Apollo Client to manage state and fetch data efficiently, ensuring smooth data flow between components.
- Optimize rendering with techniques like memoization (e.g., React.memo) to prevent unnecessary re-renders and improve performance.
- Conduct regular code reviews to ensure adherence to best practices, catch potential issues, and maintain a high standard of code quality.
Why These Practices are Essential for Scalability
Adopt these best practices to build scalable React applications:
- Modular design and separation of concerns make it easier to manage growing complexity and facilitate independent development of features.
- Centralized state management and performance optimizations ensure the application can handle increasing amounts of data and traffic without degradation.
- Automated testing and code reviews help maintain high-quality code and prevent regressions as new features are added, enabling the application to scale reliable.
Follow these best practices to create React applications that are visually appealing, user-friendly, and highly scalable, performant, and maintainable, ready to adapt to future demands.
React JS App Architecture Strategies
What Are the Different React JS App Architecture Strategies?
Several architecture strategies exist for building scalable React JS applications:
-
Component-Based Architecture: Focuses on creating reusable, self-contained components for better organization and maintainability.
-
State Management Patterns: Libraries like Redux or MobX ensure predictable data flow and simplify state management.
-
Container/Presentational Pattern: Separates components into logic-based (containers) and UI-based (presentational), enhancing reusability and clarity.
-
Feature-Based Structure: Organizes code by features instead of file types, improving scalability.
-
Micro-Frontend Architecture: Allow independent development and deployment of different features, making it suitable for larger apps.
How to Choose the Right Strategy for App Needs
To choose the best React JS architecture:
-
Project Size: For smaller projects, use a simple component-based approach. For larger apps, consider micro-frontend architture.
-
Team Structure: If teams work independently, adopt a micro-frontend strategy for better collaboration.
-
Scalability: If growth is expected, prioritize modular, scalable strategies.
-
Performance: Focus on strategies that integrate state management and React JS best practices for performance.
By using these strategies, you can build a scalable React app that meets your project’s needs while ensuring maintainability and performance.
Scalable React App: Implementation Tips
How to Implement Scalability in a React App
To ensure scalability in your React JS architecture, follow these key practices:
-
Use Component Libraries: Libraries like Material-UI or Ant Design speed up development and provide consistent, reusable UI components.
-
Optimize State Management: Choose state management solutions like Redux or Zustand to manage application state efficiently and ensure smooth data updates.
-
Implement Code Splitting: Utilize React.lazy() and Suspense for on-demand component loading, improving load times and performance.
-
Adopt TypeScript: Integrate TypeScript for type safety and better handling of larger, scalable React apps.
-
Utilize Performance Monitoring Tools: Tools like React Profiler and Lighthouse help identify performance bottlenecks and optimize the app.
What Are the Common Challenges And Solutions
Scaling a scalable React app comes with challenges:
-
Performance Bottlenecks: Slow rendering can occur as the app grows.
Solution: Use React.memo to optimize rendering and manage state carefully.
-
Complex State Management: State management can become difficult as components grow.
Solution: Use centralized state management libraries like Redux or Recoil.
-
Code Maintainability: Larger codebases can be harder to maintain.
Solution: Adopt feature-based structures and consistent naming conventions for better organization.
When to Scale Your React App
Consider scaling your React architecture when:
-
User Growth: Increased traffic requires performance optimization.
-
Feature Expansion: Difficulty adding new features signals the need for scalable practices.
-
Performance Issues: Lag or slow load times call for architecture improvements.
-
Team Growth: A larger team benefits from a scalable architecture to maintain collaboration and quality.
Apply these React JS strategies to build and maintain a scalable React app that adapts efficiently to future demands.
React JS Strategies for Optimal Performance
What are effective React JS strategies for performance?
To optimize performance in your React JS architecture, apply these strategies:
-
Code Splitting: Use React.lazy() and Suspense to dynamically load components only when needed, improving initial load times.
-
Memoization: Utilize React.memo for functional components and PureComponent for class components to avoid unnecessary re-renders.
-
Virtualization: Implement libraries like react-window to render only visible parts of large lists, reducing rendering overhead.
-
Optimize State Management: Use local state where possible, and for global state, opt for libraries like Redux or Zustand to streamline updates.
-
Debounce and Throttle: Limit the frequency of input or scroll event handling with debouncing and throttling, improving responsiveness.
How to integrate these strategies into your development process
To integrate these strategies into your React JS app architecture:
-
Plan Early: Incorporate performance optimization during the architecture phase. Use code splitting and efficient state management from the start.
-
Use Monitoring Tools: Utilize tools like React Profiler and Lighthouse to track performance and spot issues early.
-
Code Reviews: Focus on performance during reviews, ensuring memoization and state management practices are followed.
-
Test Performance: Use Jest and React Testing Library to test changes and avoid performance regressions.
Why performance optimization is critical for scalability
Optimizing performance is critical for a scalable React app:
-
Improved User Experience: Fast apps retain users, which is vital as your user base grows.
-
Resource Efficiency: Optimized apps consume fewer resources, aiding scalability.
-
Bottleneck Prevention: Addressing performance issues early ensures smoother scaling.
-
Feature Expansion: Well-optimized apps handle additional features without degrading performance.
Implementing these React JS strategies ensures your app is both efficient and scalable, ready to grow with user demands.
Conclusion
This guide has outlined essential principles and strategies for building a scalable React app. By focusing on a component-based architecture, utilizing efficient state management like Redux or Zustand, and optimizing performance with techniques like code splitting and memoization, you can create applications that are both adaptable and maintainable.
Scalability should be a priority from the start, with continuous performance monitoring and architectural adjustments as your app grows. Stay updated on React JS best practices and experiment with new strategies to ensure your app is equipped to handle future challenges. By following these guidelines, you’ll build a scalable React app that is efficient, user-friendly, and ready for growth.
The above is the detailed content of Build Scalable React App: React JS architecture Guide. For more information, please follow other related articles on the PHP Chinese website!