Decrypt Go: empty struct
In Go, a normal struct typically occupies a block of memory. However, there's a special case: if it's an empty struct, its size is zero. How is this possible, and what is the use of an empty struct?
This article is first published in the medium MPP plan. If you are a medium user, please follow me in medium. Thank you very much.
type Test struct { A int B string } func main() { fmt.Println(unsafe.Sizeof(new(Test))) fmt.Println(unsafe.Sizeof(struct{}{})) } /* 8 0 */
The Secret of the Empty Struct
Special Variable: zerobase
An empty struct is a struct with no memory size. This statement is correct, but to be more precise, it actually has a special starting point: the zerobase variable. This is a uintptr global variable that occupies 8 bytes. Whenever countless struct {} variables are defined, the compiler assigns the address of this zerobase variable. In other words, in Go, any memory allocation with a size of 0 uses the same address, &zerobase.
Example
package main import "fmt" type emptyStruct struct {} func main() { a := struct{}{} b := struct{}{} c := emptyStruct{} fmt.Printf("%p\n", &a) fmt.Printf("%p\n", &b) fmt.Printf("%p\n", &c) } // 0x58e360 // 0x58e360 // 0x58e360
The memory addresses of variables of an empty struct are all the same. This is because the compiler assigns &zerobase during compilation when encountering this special type of memory allocation. This logic is in the mallocgc function:
//go:linkname mallocgc func mallocgc(size uintptr, typ *_type, needzero bool) unsafe.Pointer { ... if size == 0 { return unsafe.Pointer(&zerobase) } ...
This is the secret of the Empty struct. With this special variable, we can accomplish many functionalities.
Empty Struct and Memory Alignment
Typically, if an empty struct is part of a larger struct, it doesn't occupy memory. However, there's a special case when the empty struct is the last field; it triggers memory alignment.
Example
type A struct { x int y string z struct{} } type B struct { x int z struct{} y string } func main() { println(unsafe.Alignof(A{})) println(unsafe.Alignof(B{})) println(unsafe.Sizeof(A{})) println(unsafe.Sizeof(B{})) } /** 8 8 32 24 **/
When a pointer to a field is present, the returned address may be outside the struct, potentially leading to memory leaks if the memory is not freed when the struct is released. Therefore, when an empty struct is the last field of another struct, additional memory is allocated for safety. If the empty struct is at the beginning or middle, its address is the same as the next variable.
type A struct { x int y string z struct{} } type B struct { x int z struct{} y string } func main() { a := A{} b := B{} fmt.Printf("%p\n", &a.y) fmt.Printf("%p\n", &a.z) fmt.Printf("%p\n", &b.y) fmt.Printf("%p\n", &b.z) } /** 0x1400012c008 0x1400012c018 0x1400012e008 0x1400012e008 **/
Use Cases of the Empty Struct
The core reason for the existence of the empty struct struct{} is to save memory. When you need a struct but don't care about its contents, consider using an empty struct. Go's core composite structures such as map, chan, and slice can all use struct{}.
map & struct{}
// Create map m := make(map[int]struct{}) // Assign value m[1] = struct{}{} // Check if key exists _, ok := m[1]
chan & struct{}
A classic scenario combines channel and struct{}, where struct{} is often used as a signal without caring about its content. As analyzed in previous articles, the essential data structure of a channel is a management structure plus a ring buffer. The ring buffer is zero-allocated if struct{} is used as an element.
The only use of chan and struct{} together is for signal transmission since the empty struct itself cannot carry any value. Generally, it's used with no buffer channels.
// Create a signal channel waitc := make(chan struct{}) // ... goroutine 1: // Send signal: push element waitc <- struct{}{} // Send signal: close close(waitc) goroutine 2: select { // Receive signal and perform corresponding actions case <-waitc: }
In this scenario, is struct{} absolutely necessary? Not really, and the memory saved is negligible. The key point is that the element value of chan is not cared about, hence struct{} is used.
Summary
- An empty struct is still a struct, just with a size of 0.
- All empty structs share the same address: the address of zerobase.
- We can leverage the empty struct's non-memory-occupying feature to optimize code, such as using maps to implement sets and channels.
References
- The empty struct, Dave Cheney
- Go 最细节篇— struct{} 空结构体究竟是啥?
The above is the detailed content of Decrypt Go: empty struct. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


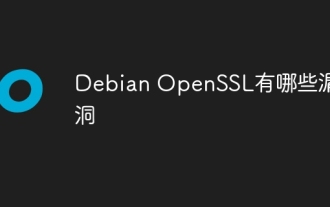
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
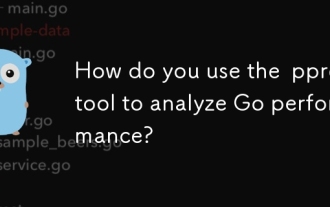
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
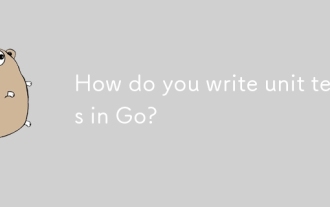
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
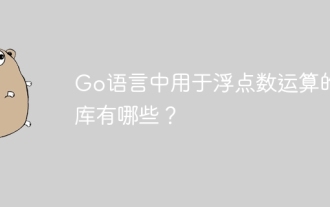
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
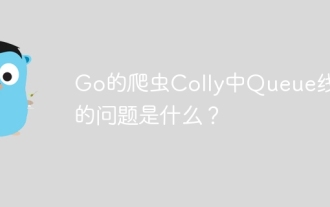
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
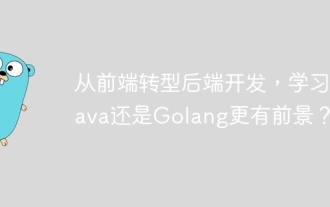
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
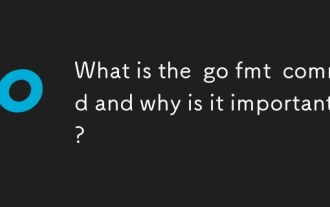
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
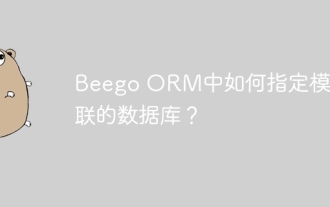
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
