Determine Available Selections Depending on Available Product SKUs
If you work or have worked in an e-commerce application in the past, you probably encountered having to deal with the product show page. This is the page the user sees before they decide to add a product to the cart or not. As with any other pages, this page has to load fast and it also has to display crucial information about the product like description, pictures and available options.
Available options are the different available variations of a product. For example, a men's shirt usually comes in different sizes, however sometimes the store may run out of a given size. For situations like this, it is beneficial to disable the selection so that the user knows ahead of time that this variations is not available for this given product.
One of two ways to approach this is to make an api call to the backend with the current selections to determine which options are available based on these selections. For example, if I select the color green, I should only see sizes that are available for that specific color. If size medium is not available in green then the option to select medium should be disabled as long as green is previously selected. With this first approach the database would be queried to determine which are the rest of available options based on the currently selected options. This would query the database for ProductSkus, ProductSkuOptions, and ConfigurableOptions along with 10 different queries on these tables. This would be done for every single user selection made.
The second way is for the backend to return a list of the available variations in form of SKUs ('ZARA-001-RED-S', 'ZARA-001-BLUE-S', 'ZARA-001-GREEN-S', 'ZARA-001-RED-M', 'ZARA-001-BLUE-M'). This list of SKUs can be part of the product details api call and it would add a single database query which is ProductSkus.where(product_id:). This query (ruby on rails) returns the list of skus tied to a product.
The first approach entails having to have a loading state between selections which is doable but not ideal to modern web development standards. The second approach is faster and executes virtually instantaneously, no need for loading states. The first approach delegates the heavy work to the backend while the second approach does all the heavy work in the frontend, however the frontend will execute much faster since there is no need for database communication.
I will focus on the second approach for this post.
const updateUIBasedOnSelection = () => { const newAvailableOptions = filterAvailableOptions( selectedOptions, Object.keys(availableOptions), product.available_skus ) // Go through each selection and see what is available according to the other selections Object.keys(availableOptions).forEach(type => { const selectedOptionsCopy = { ...selectedOptions } delete selectedOptionsCopy[type] // remove the current selection so we can see what is available according to the other selections const newAvailableOptionsWithSelf = filterAvailableOptions( selectedOptionsCopy, Object.keys(availableOptions), product.available_skus ) newAvailableOptions[type] = new Set([...newAvailableOptions[type], ...newAvailableOptionsWithSelf[type]]) return newAvailableOptionsWithSelf }) setAvailableOptions(newAvailableOptions) }
This code runs on a hook that watches for selectedOptions changes. This code along with the filterAvailableOptions function determines which options will be disabled. The data structures used here are objects with variants name as key (e.g., 'color' and 'size') and javascript sets (Set), similar to arrays but values are unique, values cannot repeat in a Set.
Available options is constructed from all the available skus and it initializes with the values:
{ 'color': new Set('RED', 'BLUE', 'GREEN'), 'size': new Set('S', 'M') }
Another more feasible approach is to also use the variant id as the key instead of the variant type.
{ 1: new Set('RED', 'BLUE', 'GREEN'), 2: new Set('S', 'M') }
This way the code is not constraint to having a variant that may display the same type. Maybe there can be two color selections for example.
Inventory
In addition to the sku existing, you may also want to do a stock check of all the possible options the user can select, this way the user can tell at a glance if an option is available or not. For this you can find all the skus that match the current selection so far.
If the user has already chosen the color red, look for all the skus that contain the color red and do a stock check of all the skus that match the color red. This way you would be able to tell if the next possible chosen option is available or not.
However, the user may want to change her/his mind and instead of deciding color red then size xs s/he could choose the color red, change her/his mind and change to color green. Your algorithm should be flexible enough that it always fetches the sku. Sometimes it would be needed to fetch all available options as the user makes selection. Walmart for example does a stock check whenever the user makes a selection.
Another thing to keep in mind is the backend portion of this. Sometimes the upcoming selections could be as many as hundreds. Your backend should be fast and accurate enough to handle such amount of possible selections. A quick GPT chat revealed many ways of making this fast and accurate, many of which consisted in using event driven code that updates the inventory whenever a transaction occurs. This is sensitive as it may be out of sync if not done correctly, remember mutex and avoid race conditions where two customers may be purchasing the item at the same time. If I had to choose, I would choose a combination of Kafka for events and Redis for caching the inventory values.
In my personal case I did not have to choose either of, only had to optimize a backend query to make sure it ran 20 skus per 2secs. I narrow down the skus as the user makes selections, so the more selections the user has made the less the skus I have to check the inventory for and the quicker the api call is.
Anyways, I still have to figure out if I should be fetching all sku matches or the remaining skus waiting to be selected. All matching skus are all of the skus that match the current selection and remaining skus are the ones that are missing to be selected by the user.
In ecommerce is very important to deliver code that's performant because some people rely on the service to receive emotional comfort from the purchase experience and sometimes from the item they're purchasing. Using an app that is poorly written can ruin someone's day by resulting in an emotional need not being met, which in turn may result in poor decision making skills.
The sku check may only be done at the beginning of the product show page load but the stock check can be done as the user makes selections. So in essence only one fetch for the skus and multiple fetches for the stock checks.
Conclusion
There are most likely multiple ways to achieve this result. This way is virtually instantaneous. There are only so many different variations of the same product so there should be no need to optimize it further. I kept part of the code to myself to not get in trouble with the company I am working for at the moment but I'd be happy to discuss your intended approach.
Long story short fetch all skus (which should change as the user selection changes) and build the available options selection elements by looking at the different sku options.
The above is the detailed content of Determine Available Selections Depending on Available Product SKUs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


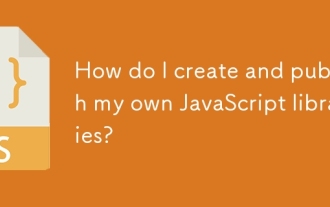
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
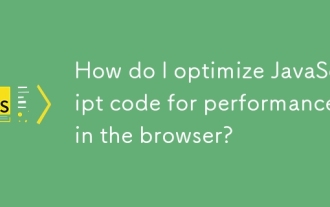
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
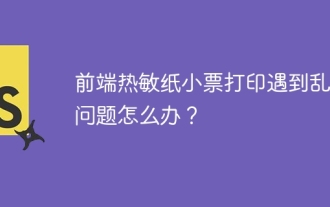
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
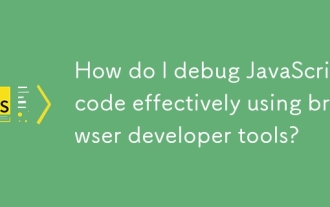
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
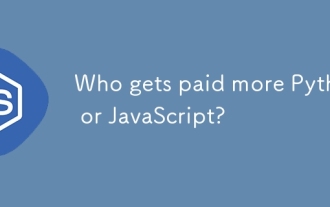
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
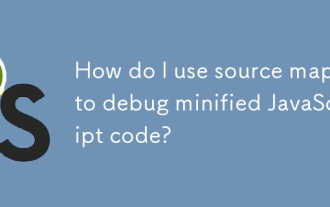
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
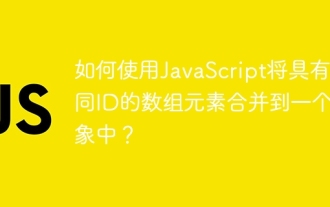
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
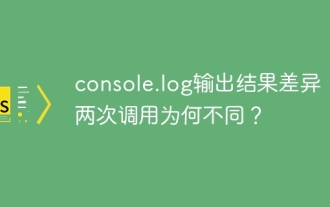
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
