Understanding Local Storage in JavaScript
Local Storage is an essential browser-based API that allows developers to store, retrieve, and manage data directly in the browser. Unlike session storage, Local Storage persists even after the browser is closed, making it ideal for saving user preferences, app settings, or any kind of data that needs to stick around between sessions. However, it’s important to note that the data is limited to the browser in which it’s stored. For instance, data saved in Chrome won’t be available in Firefox.
How Local Storage Works
Before working with Local Storage, it's important to understand that it stores data in JSON format. This means that if you're saving a JavaScript object, you'll need to convert it into JSON first, and convert it back to a JavaScript object when retrieving the data.
Here’s an example:
const user = { name: "AliceDoe" }; const userToJSON = JSON.stringify(user); // Convert object to JSON
Viewing Local Storage in Your Browser
You can view and interact with the data stored in Local Storage using your browser’s Developer Tools. Here's a quick guide:
- Right-click on any webpage and select "Inspect" or press F12.
- Open the Application tab.
- In the left panel, find Local Storage under the storage section, and you'll see your stored data displayed as key-value pairs.
Creating a New Record in Local Storage
To store data in Local Storage, follow these steps:
const user = { name: "AliceDoe" }; const userToJSON = JSON.stringify(user); // Convert to JSON localStorage.setItem("user", userToJSON); // Save the item
In this example:
- The key is "user".
- The value is the stringified object in JSON format.
Reading Data from Local Storage
When you retrieve data from Local Storage, you'll need to convert the JSON string back into a JavaScript object:
const userJSON = localStorage.getItem("user"); // Retrieve data const userObject = JSON.parse(userJSON); // Convert back to JS object console.log(userObject); // { name: "AliceDoe" }
Updating Existing Data in Local Storage
Updating data in Local Storage is similar to creating a new record—essentially, you overwrite the old data:
const updatedUser = { name: "AliceDoe", age: 25 }; const updatedUserJSON = JSON.stringify(updatedUser); localStorage.setItem("user", updatedUserJSON); // Overwrite the record
Deleting Data from Local Storage
Finally, to remove a record from Local Storage, you can use the removeItem method:
localStorage.removeItem("user"); // Remove the "user" record
This will delete the record associated with the "user" key.
Conclusion
Local Storage is a powerful, easy-to-use tool for client-side data persistence in JavaScript. By understanding how to create, read, update, and delete records, you can store important data that persists across browser sessions, enhancing the user experience. However, it’s also important to remember that Local Storage is limited to a specific browser and domain, and it should not be used for sensitive data, as it’s not encrypted.
By incorporating Local Storage into your applications, you can improve their functionality without needing a full backend solution for certain tasks.
Citations:
- MDN Web Docs, "LocalStorage", https://developer.mozilla.org/en-US/docs/Web/API/Window/localStorage
The above is the detailed content of Understanding Local Storage in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


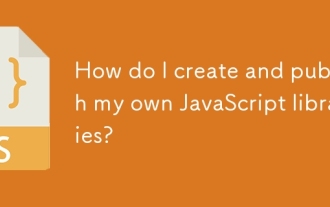
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
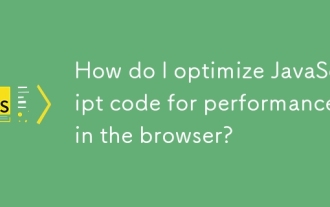
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
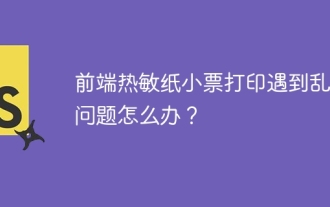
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
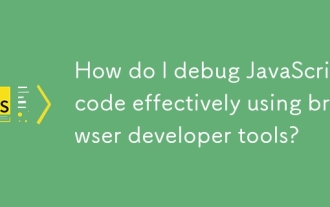
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
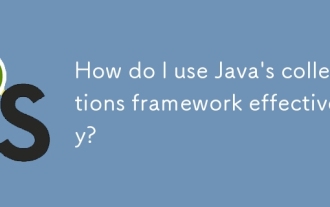
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
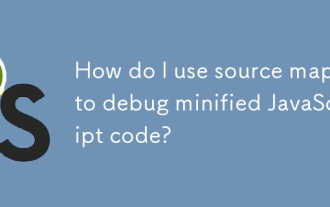
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
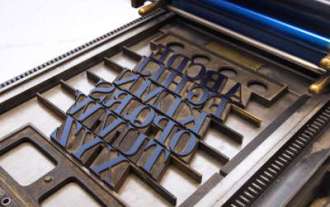
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
