Simple Guide to Integrate Juspay in Your TypeScript React App
This guide provides step-by-step instructions on how to integrate Juspay into a React app using TypeScript for both front-end and back-end parts of the payment process.
Prerequisites
Ensure you have the following credentials for Juspay:
- Merchant ID
- Client ID
- API Key
Integration Flow
Here is the flow of payment integration using Juspay:
Steps for TypeScript Integration
1. Create Payment Session (Server-Side in TypeScript)
Use Node.js/Express with TypeScript to create the payment session using Juspay's API.
Create a TypeScript PaymentSession interface to type your response:
interface PaymentSession { payment_link: string; order_id: string; status: string; }
TypeScript code for creating a session:
import axios from 'axios'; import base64 from 'base-64'; import { Request, Response } from 'express'; const createSession = async (req: Request, res: Response) => { const apiKey = 'your_api_key'; const authHeader = base64.encode(`${apiKey}:`); try { const response = await axios.post<PaymentSession>( 'https://api.juspay.in/session', { customer_id: 'customer_id_here', amount: 10000, // Amount in paise (100 INR) currency: 'INR', }, { headers: { Authorization: `Basic ${authHeader}`, }, } ); res.json(response.data); } catch (error) { res.status(500).json({ error: (error as Error).message }); } }; export default createSession;
In this code:
- PaymentSession interface ensures type safety for the session response.
- TypeScript's type assertions ensure accurate error handling (error as Error).
2. Initiate Payment from React Client (TypeScript)
In the React client, create a component that initiates the payment process using a useEffect hook and Axios for API requests.
Define an interface for the session response:
interface PaymentSession { payment_link: string; order_id: string; }
Component in TypeScript:
import React, { useState } from 'react'; import axios from 'axios'; const PaymentPage: React.FC = () => { const [paymentUrl, setPaymentUrl] = useState<string | null>(null); const initiatePayment = async () => { try { const response = await axios.post<PaymentSession>('/api/create-session', { amount: 10000, // Amount in paise (100 INR) currency: 'INR', customer_id: 'your-customer-id', }); setPaymentUrl(response.data.payment_link); } catch (error) { console.error('Error initiating payment:', error); } }; return ( <div> <h1>Initiate Payment</h1> <button onClick={initiatePayment}>Pay Now</button> {paymentUrl && ( <div> <a href={paymentUrl} target="_blank" rel="noopener noreferrer"> Complete Payment </a> </div> )} </div> ); }; export default PaymentPage;
In this code:
- PaymentSession ensures the expected structure of the response from the backend.
- The initiatePayment function sends the request to initiate the payment and handles the response.
3. Handling Return URL and Checking Payment Status
When the user is redirected back after the payment, you need to check the payment status and display it.
TypeScript Interface for Payment Status:
interface PaymentStatus { status: string; order_id: string; amount: number; }
React Component to Handle Payment Status:
import React, { useEffect, useState } from 'react'; import axios from 'axios'; const PaymentStatusPage: React.FC = () => { const [paymentStatus, setPaymentStatus] = useState<string | null>(null); useEffect(() => { const checkPaymentStatus = async () => { try { const response = await axios.get<PaymentStatus>('/api/check-status', { params: { order_id: 'your-order-id' }, }); setPaymentStatus(response.data.status); } catch (error) { console.error('Error fetching payment status:', error); } }; checkPaymentStatus(); }, []); return ( <div> <h1>Payment Status</h1> {paymentStatus ? ( <p>Payment Status: {paymentStatus}</p> ) : ( <p>Checking payment status...</p> )} </div> ); }; export default PaymentStatusPage;
In this component:
- You send the order_id to your backend to check the payment status.
- The backend should return the status based on the response from Juspay's API.
4. Handling Webhooks with TypeScript (Backend)
Juspay will send a webhook to notify you of payment status changes. Below is how to handle this in a TypeScript environment.
import { Request, Response } from 'express'; interface JuspayWebhook { order_id: string; status: string; amount: number; currency: string; } const handleWebhook = (req: Request, res: Response) => { const paymentUpdate: JuspayWebhook = req.body; console.log('Payment Update Received:', paymentUpdate); // Process the payment update (e.g., update your database) // Respond to Juspay to confirm receipt res.status(200).send('Webhook received'); }; export default handleWebhook;
5. Respond to Juspay with a 200 OK (Backend)
To confirm receipt of the webhook notification, your server should return a 200 OK status:
app.post('/api/webhook', (req: Request, res: Response) => { // Acknowledge the webhook res.status(200).send('OK'); });
Conclusion
By following these steps and leveraging TypeScript for both client and server-side code, you can integrate Juspay into your React app efficiently and safely. TypeScript adds the benefit of type safety, reducing errors and ensuring your integration is smooth.
- Client Side: You initiate payment using React components and check the status.
- Server Side: Your Node.js/Express backend handles the payment session, status, and webhook notifications.
This guide provides a complete overview of how to integrate Juspay into a modern web stack using TypeScript.
The above is the detailed content of Simple Guide to Integrate Juspay in Your TypeScript React App. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










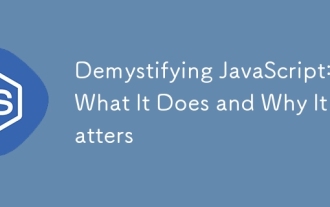
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
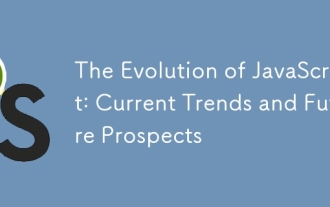
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
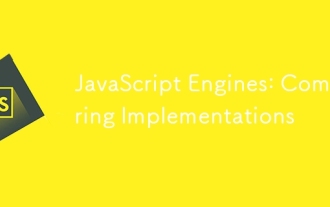
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
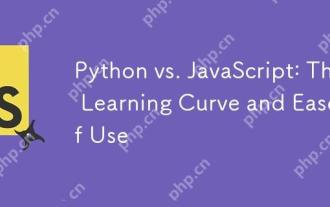
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
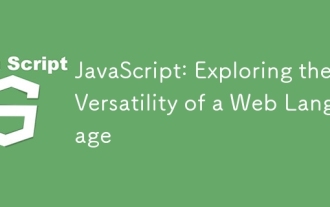
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
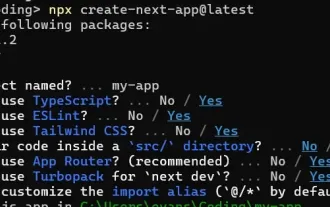
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
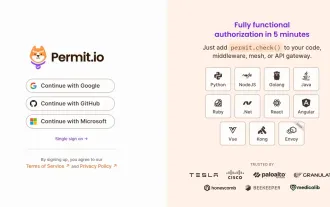
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
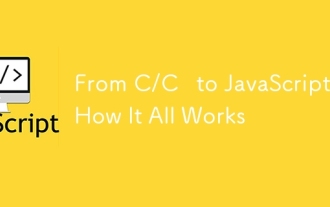
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
