


Understanding Rendering and Rerendering in React Apps: How They Work and How to Optimize Them
When we create applications in React, we often come across the terms rendering and re-rendering components. Although this may seem simple at first glance, things get interesting when different state management systems like useState, Redux, or when we insert lifecycle hooks like useEffect are involved. If you want your application to be fast and efficient, understanding these processes is key.
What is Rendering?
Rendering is the process by which React displays your user interface (UI) on the screen, based on state or props. When your component is rendered for the first time, it is called the first render.
How Does Initial Render Work?
When a component is first "mounted" to the DOM, here's what happens:
1. State initialization:
Whether you use useState, props or Redux, the initial state of the component is created.
2. Render function:
React loops through the JSX code and generates a virtual DOM based on the current state.
3. Creates a virtual DOM (Virtual DOM) for the current state of the component.
4. Comparing (diffing):
The virtual DOM is compared to the real DOM (since it is the first render, all elements will be fully rendered).
5. Display:
The component is displayed on the screen.
Once the component is rendered, the next challenge is to render it.
Re-rendering: When and Why?
Rerendering happens every time the state or props change. Did you click the button that changes the number on the screen? Changed a value in the Redux store? All of those actions can cause React to render the component again, and that's where rerendering comes in.
How Does Rerendering Work?
State change detection:
With useState, when you call setState, React knows it needs to update the component.
With Redux, when a value in the store changes, every component associated with that part of the state is re-rendered.
Render trigger:
When state changes, React creates a new virtual DOM based on that change.
Comparing (diffing):
- React compares the new virtual DOM with the old one and calculates what changes to apply. This is one way React optimizes rendering.
View changes:
- After the changes are calculated, React applies them to the actual DOM. Thus, only the changed parts of the page are displayed again.
Which Components Are Rendered?
Not all components are affected by every change. React rerenders only those components that:
Use local states:
If you use useState, the component is rerendered every time setState.
Use Redux state:
If your component depends on Redux state (via useSelector or connect), it will be re-rendered when that part of the state changes.
Use props:
If the props value changes, the component is re-rendered with the new values.
Optimization of Rendering
Of course, it's not always ideal to needlessly re-render all components. If we want the application to work quickly and efficiently, here are some optimization techniques:
1. Memoization of Components
React offers functionality for component memoization via React.memo. If your component doesn't depend on props or state changes, you can "remember" it, so it will re-render only when the relevant values change.
Example:
1 |
|
2. Memorization of Functions and Values
To avoid recreating functions or values on every render, use useCallback to memoize functions and useMemo to memoize values.
useCallback allows you to memoize a function and prevent it from being recreated until the dependencies change.
useMemo memoizes the result of the function, so it is not recomputed on each render.
Example:
1 2 3 4 5 6 7 |
|
3. Redux Optimization
If you use Redux, you can further optimize your application with memoized selectors such as reselect. This allows to avoid re-rendering components that are not affected by the state change.
Lifecycle Hook-ovi i Rerenderovanje
U klasičnim React klasama, koristili smo shouldComponentUpdate da kontrolišemo kada će se komponenta ponovo renderovati. U funkcionalnim komponentama, ovaj koncept se može simulirati pomoću useEffect i memoizacije.
Zaključak
Renderovanje i rerenderovanje su ključni za prikaz korisničkog interfejsa u React aplikacijama, ali pravilno razumevanje i optimizacija tih procesa može napraviti razliku između spore i super brze aplikacije. Ispravno korišćenje memoizacije, useCallback, useMemo, kao i pažljivo rukovanje Redux-om, pomaže da izbegnemo nepotrebne re-rendere i održimo naše aplikacije brzim i responzivnim.
Primer Koda: Renderovanje i Rerenderovanje u Akciji
Evo primera komponente koja koristi useState, Redux i memoizaciju da optimizuje renderovanje:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
|
Kao što vidimo, ovde se koristi kombinacija lokalnog state-a, Redux-a, memoizacije i useEffect hook-a da bi aplikacija bila što efikasnija.
The above is the detailed content of Understanding Rendering and Rerendering in React Apps: How They Work and How to Optimize Them. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










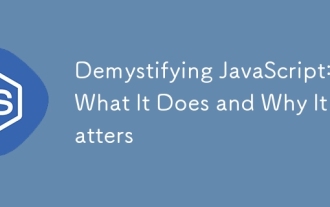
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
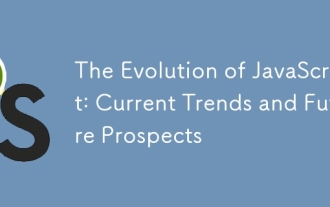
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
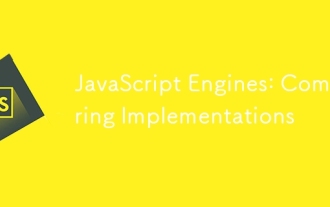
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
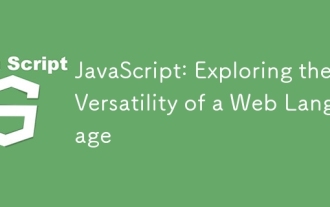
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
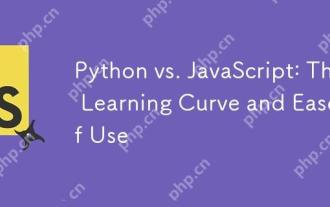
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
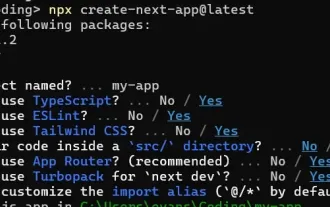
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
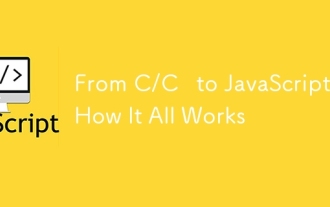
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
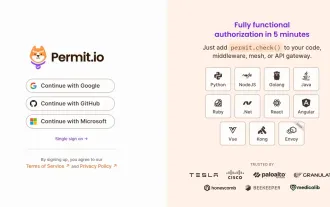
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
