Execution Context & Call Stack
Creation of global execution context for the top level code i.e code which not inside any fn. Hence, code outside the fn is executed first.
Code inside fn body of fn-decln/exprsn is only executed when its called.
Execution Context(EC)
Environment in which piece of JS is executed.
Stores all the necessary information for some code to be executed like local variables, args passed to a fn.
JS code always runs inside an EC.
Exactly one global EC irrespective of the size of JS project.
Default context, created for code not inside any fn.
Then the code is executed inside the global EC
After the top level code is executed, execution of fns & waiting for C/bs
For each fn call, a new EC is created to exectute that fn. Same goes for methods, as they are also fns attached to objects.
All these ECs together make up the Call Stack.
When all fns are executed, engine waits for CBs to arrive & execute them. Ex. click event callback, provided by the event loop.
What is inside EC
- Variable Environment consisting of
- let, const, var declarations
- Functions
arguments object : stores all the args passed to the fn in its EC.
Each fn gets its own EC as its called. And variables declared end up in variable environmentScope Chain:
Fns can access variables outside of fns using scope chain.
Contains references to the variables located outside of the current fn and to keep track of the scope chain, it is stored in each EC.Each EC also get the 'this' keyword.
All these three above are generated during "Creation Phase", right before execution. These the things necessary to run the code in top level.
For arrow fns EC:
We won't be having: arguments object, this keyword. Arrow fns use from their closest regular fn, the above two.
arguments: array like object, containing all the arguments passed into the regular fn, not arrow fn.
Call Stack Memory Heap = JS Engine
Call Stack
Place where ECs get stacked on top of each other, to keep track of where we are in the execution. The topmost EC is the one we are running. As the execution is over, its removed from the top of stack and the control is trasferred to the underlying EC.
If there is a nested fn call, the outer fn call will be paused so as to return the result of the execution of inner fn on call stack as JS has only one thread of execution. Now the prev EC will become the active EC
Then the top-most EC is popped out of Call Stack as its returned.
Lowest in Call Stack will be global EC, on top it will be fn calls as they occur in order.
Ensures order of execution never gets lost.
In the end, program will be finished and global EC will also be popped out the Call Stack.
JS code runs inside an EC, which are placed on Call Stack.
Hence, we can say that each EC has: 1. Variable environment 2. Scope chain 3. 'this' keyword
Scoping
How our program variables are organized and accessed by JS Engine.
Where do variables live
Where can we access a certain variables and where not.
Lexical Scoping:
JS has leical scoping which means scoping is controlled by placement of fns and blocks in the code.
Ex. A nested fn has access to variables of its parent fn.
Scope:
Space or env in which a certain variable is declared(variable environment in case of fns). Its the variable env which is stored in fns EC.
For fns, Var env & scope both are same.
Three scopes in JS are: 1. Global scope 2. Fn scope 3. Block scope [ES6]
Scope is a place where variables are declared. Hence, true for Fns also as fns are just values stored in variables.
Scope of a Variable
Region of our code where a certain variable can be accessed.
Scope is different from scope of a variable with subtle differences.
## Global Scope: For top level code For variables declared outside of any fn or block which are accessible from everywhere Variables in this scope are at the top of scope chain. Hence, can be used by every nested scope.
## Fn Scope: Each fn has creates its own scope Variables are accessible ONLY inside fn, NOT outside. Else Reference Error Also called local scope Fn decln, exprsn, arrow all three create their own scopes. Only way to create scope using ES5 which had only fn & global scope.
## Block Scope: Introduced in ES6, not only fn but {} also create a scope known as block scope which work only for ES6 variables i.e let-const types. DOesn't work for variables declared with 'var' as its fn scoped. Variables accessible only inside block i.e {} This only applies to variables declared with let-const only. Fns are also block scoped in ES6 (only in strict mode, should be used) variables declared using 'var' will be accessible outside the block Scoped to the current fn or the global scope. var variables only care about fn, they ignore blocks. They end up in nearest fn scope.
Every nested scope has access to variables from its outer scope & the global scope. Same is applicable to fn arguments also.
If a fn doesn't find the variable in its scope, it looks up the scope chain to find out the varibles in its outer scopes. This process is called variable lookup in scope chain. This doesn't work the other way around i.e we cannot access nested fn variables or scopes from outside the fn or outer scopes.
Sibling scopes cannot access each other's variables
Only the innermost scope can access its outer scopes, not the reverse.
One EC for each fn in the exact order a fn is called is placed on Call Stack with its variables inside the EC. Global EC is at the bottom of the Call Stack
Scope chain:
Its all about the order in which fns are written in the code.
Has nothing to do with order in which fns were called.
Scope chain gets the variable environment from the EC.
Order of fn calls is not relevant to the scope chain at all.
const a = 'Alice'; first(); function first(){ const b = "Hello"; second(); function second(){ const c = "Hi"; third(); } } function third(){ const d = "Hey"; console.log(d + c + b + a); // Reference Error } ## Call Stack order: third() EC - top second() EC first() EC global EC - bottom Scope Chain: second() --nested inside--> first() --nested inside--> global scope. third() is independently defined inside gloabal scope. Reference Error occurred because both 'c' as well as 'b' cannot be accessed using the scope chain.
Summary:
E-C, Var Env, Cl-Sk, Scope, Scope-chain are all different but related concepts.
Scoping asks the questions where do variables live, where can we access the variables and where not.
Lexical Scoping in JS: Rules of where we can access variables are based exactly where in the code fns and blocks are written.
Every scope has access to all the variables from all its outer scopes. This is scope chain which is a one-way street. An outer scope can never access variables of inner scope.
Scope chain of a certain scope is equal to adding together all the Var Envs of all the parent scopes.
Scope chain has nothing to do with the order in which fns are called. It does not affect the scope chain at all.
When a variable is not found in current scope, engine looks up the scope chain until it finds the variable its looking for. This is called variable look-up.
The above is the detailed content of Execution Context & Call Stack. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










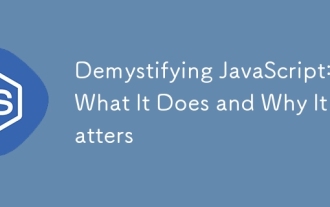
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
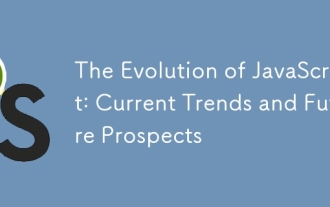
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
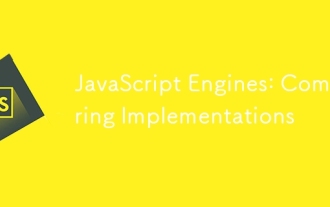
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
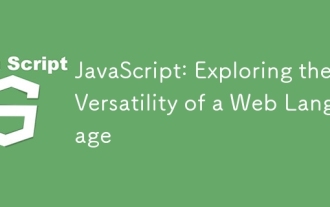
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
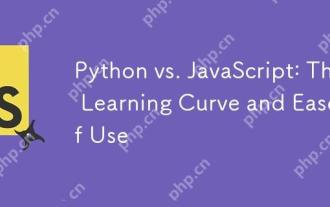
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
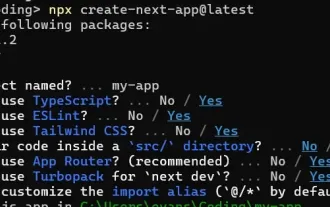
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
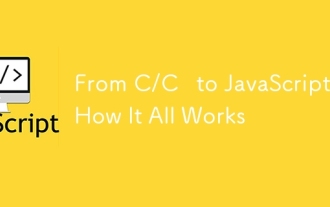
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
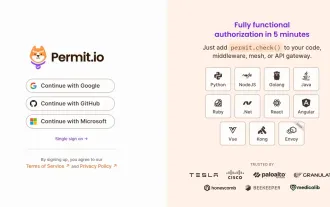
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
