Coding a linux-based OS
Table of Contents
- Introduction
- 1. The Linux Kernel: Foundation of Stability
- 2. Bootloader: Getting the System Up
- 3. System Initialization: Bringing the OS to Life
- 4. Drivers and Hardware Management
- 5. Filesystem and I/O
- 6. Graphical User Interface (GUI)
- 7. Shell and User Interaction
- 8. Conclusion: Final Thoughts on Linux OS Development
Introduction
Building a Linux-based operating system is a journey of configuration and customization, but with a lot of the groundwork already laid. Linux, as an operating system, has evolved to provide flexibility, stability, and immense community support. But while it may seem like a shortcut compared to developing a fully custom OS from scratch, there are still many moving parts and intricate details you have to consider.
Here, I’ll take you through the core steps of developing a Linux-based OS. From working with the kernel to configuring drivers, adding a GUI, and setting up a user shell, there’s plenty to dive into. Along the way, I’ll highlight the unique aspects of Linux OS development.
1. The Linux Kernel: Foundation of Stability
The Linux kernel is the heart of any Linux-based OS. It’s a powerful, well-maintained piece of software that manages system resources, handles memory management, and oversees process scheduling. By using the Linux kernel, you're relying on decades of development, testing, and improvements from one of the largest open-source communities in the world.
With Linux, the kernel’s modular design allows you to tailor your system for specific use cases. Whether you need to optimize for a server environment, a desktop system, or an embedded device, the kernel can be configured accordingly.
In a typical Linux-based OS, you interact with the kernel through system calls. These are interfaces between user-space applications and the kernel.
// Example of a simple Linux system call int result = fork(); // Create a new process if (result == 0) { execl("/bin/ls", "ls", NULL); // Execute the 'ls' command }
Kernel configuration is usually done using tools like make menuconfig, where you can enable or disable kernel modules depending on the features you need.
2. Bootloader: Getting the System Up
Every operating system needs a way to get from power-on to running the kernel, and that’s where the bootloader comes in. In the case of Linux-based systems, most people rely on GRUB (Grand Unified Bootloader). GRUB simplifies the process by providing an interface that loads the kernel and transfers control to it.
Configuring GRUB typically involves editing a grub.cfg file, which tells GRUB where to find the kernel and what options to pass to it. You don’t need to dive into assembly-level bootloading, which makes life a lot easier.
# Sample GRUB configuration snippet menuentry "Erfan Linux" { set root=(hd0,1) linux /vmlinuz root=/dev/sda1 ro quiet initrd /initrd.img }
3. System Initialization: Bringing the OS to Life
After the kernel takes control, the next major step is system initialization. This is where init systems like systemd, SysVinit, or runit come into play. The init system is responsible for starting all the necessary services, setting up the system environment, and bootstrapping the OS to a usable state.
In Linux, systemd has become the standard init system. It manages processes, services, logging, and more. For example, when you run a command like systemctl start apache2, it’s systemd that takes care of starting the Apache web server and ensuring it stays running.
Here’s a very simple service configuration for systemd:
[Unit] Description=My Custom Service [Service] ExecStart=/usr/bin/my_custom_service [Install] WantedBy=multi-user.target
Without an init system like systemd, you’d be handling process initialization manually, which involves more low-level system management, creating process control mechanisms, and dealing with service dependencies.
4. Drivers and Hardware Management
One of the trickiest parts of building any operating system is hardware management. With a Linux-based OS, you’re working with a kernel that already includes support for a vast range of hardware devices—from network interfaces to storage controllers to input devices. Many drivers are already bundled with the kernel, and any additional drivers can be loaded dynamically.
For example, you can load a driver for a specific device using the modprobe command:
modprobe i915 # Load Intel graphics driver
Linux also uses the udev device manager to detect hardware changes on the fly and load the appropriate drivers. This makes managing hardware much smoother compared to writing device drivers from scratch.
But, as always, not all drivers come bundled with the Linux kernel. Sometimes, you’ll need to compile and install third-party drivers, especially for cutting-edge or proprietary hardware.
5. Filesystem and I/O
The filesystem is the backbone of any operating system. It’s where the OS stores all its data, from system configuration files to user documents. With Linux-based systems, you have a choice between several filesystems like ext4, Btrfs, and XFS.
Choosing the right filesystem depends on your needs. Ext4 is the most common and reliable, while Btrfs offers advanced features like snapshotting and data integrity checks.
To mount a filesystem in Linux, it’s as simple as running a command like this:
mount /dev/sda1 /mnt
In addition to this, you’ll need to ensure your OS handles basic file I/O operations efficiently, using system calls like read(), write(), and open().
6. Graphical User Interface (GUI)
When you move from a headless server environment to a desktop or workstation, you need a graphical user interface (GUI). For Linux-based systems, this usually means installing X11 or Wayland for the display server and adding a desktop environment like GNOME or KDE.
Setting up a GUI on a Linux-based OS is fairly straightforward. You can use package managers to install the desktop environment and display server, then configure them to start on boot. For example, to install GNOME on Ubuntu, you would simply run:
sudo apt install ubuntu-gnome-desktop
Once installed, the user can log in and interact with the system through windows, menus, and graphical applications.
7. Shell and User Interaction
At the heart of any Linux system is the shell. Whether it’s Bash, Zsh, or another shell variant, this is where most users will interact with the system, run commands, and manage files.
Here’s an example of a basic shell interaction:
# Creating a new directory mkdir /home/user/new_directory # Listing contents of the directory ls -la /home/user
In addition to a command-line interface (CLI), many Linux-based OSes also include terminal emulators in their GUIs for those who want the power of the shell with the comfort of a graphical environment.
8. Conclusion: Final Thoughts on Linux OS Development
Developing a Linux-based operating system comes with a significant advantage: you don’t have to start from scratch. The Linux kernel handles the core system functionality, GRUB manages the boot process, and systemd handles initialization. However, this doesn’t mean the work is easy. You still need to configure, optimize, and integrate these components to create a seamless and user-friendly operating system.
The process of building a Linux-based OS is about finding the balance between customizing for your specific use case and leveraging the immense power of the Linux ecosystem. Whether you’re creating a lightweight OS for embedded systems or a feature-rich desktop environment, the journey is filled with its own set of challenges.
But hey, if it were easy, everyone would be doing it, right??
The above is the detailed content of Coding a linux-based OS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


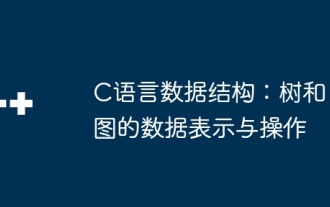
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
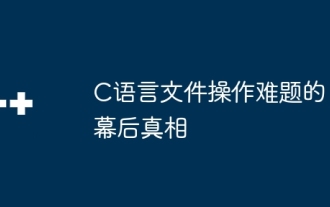
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
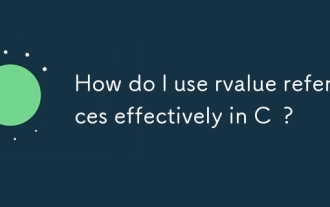
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
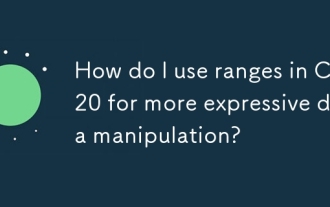
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
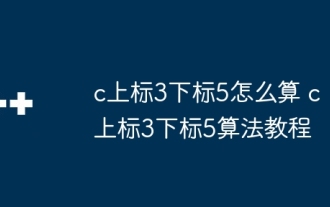
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
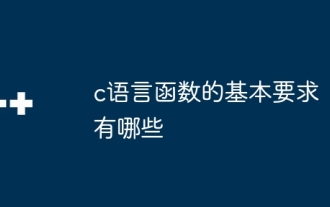
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
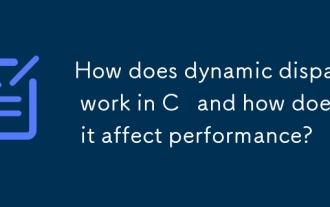
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
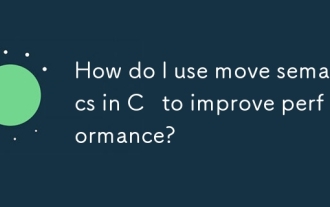
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
