Konditionner: Manage conditions in your kcustom resources
Last year, I started building operators with kubebuilder for a variety of things. Throughout the operators, I used conditions extensively to manage each step of a reconciliation process for a custom resource.
Unfortunately, the sigs.k8s.io's Conditions left me wanting more. I had some issues with conflicts, broken states, etc. And as I dug more into the problem, I started creating a sort of framework around my usage of conditions.
I finally took some times recently to package those findings into a library for others to use. I call it Konditionner. At its core, the goal of Konditionner is to offer:
- Fully extendable condition types and statuses
- Utility API to work with Conditions
- Advisory Lock
How to use
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
The MyCRD custom resource is pretty bare but it's ready to be used! Konditionner doesn't come with any type defined, as that's specific to every use. Here, I created 5 of them, which means that I'll work with 5 conditions in the reconciliation loop.
Let's pretend I have a reconciliation loop where I want to create a pod. The condition's goal is to move from Initialized and progress towards one of two outcome: Created if it was successful, Error if there was an error in the process.
Advisory Lock on condition
Since reconciliation loop work on top of a distributed database(etcd) and a cache layer, I've found it to be a lot more reliable to create a "lock" on the condition before executing a task.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
For the lock to work, the record needs adhere to the konditions.ConditionalResource interface. This is why, at the top, the custom resource has the Conditions() method defined:
1 2 3 |
|
The documentation is available on pkg.go.dev and the source is available on Github.
The above is the detailed content of Konditionner: Manage conditions in your kcustom resources. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










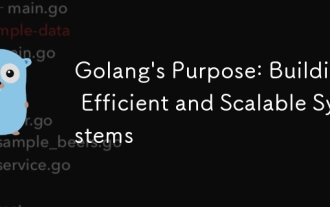
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
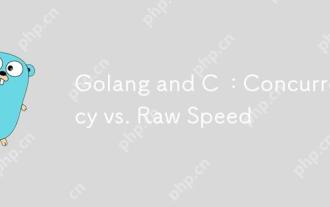
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
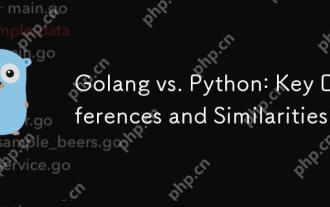
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
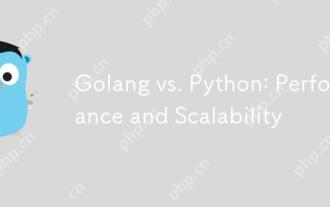
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
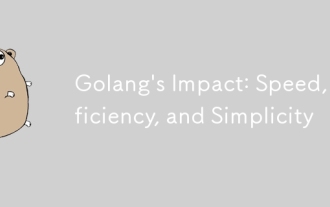
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
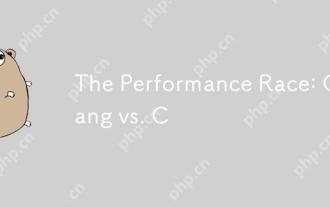
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
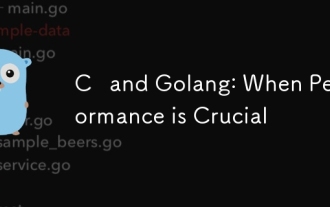
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
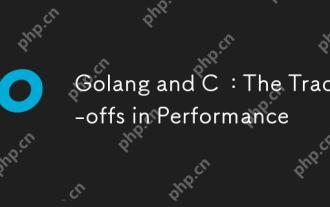
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
