hings You Should Know about the This keyword in Java.
1. What is the this Keyword in Java?
The this keyword in Java is a reference to the current object. It is used within an instance method or constructor to refer to the object that is currently being constructed or invoked.
1.1 Purpose of the this Keyword
The primary purpose of the this keyword is to distinguish between instance variables (fields) and parameters or local variables with the same name. It is also used to pass the current object as a parameter to other methods, return the current object, and invoke other constructors in a constructor.
1.2 Example: Distinguishing Between Instance Variables and Parameters
Consider the following example where this is used to differentiate between instance variables and method parameters:
public class Employee { private String name; private int age; public Employee(String name, int age) { this.name = name; // 'this.name' refers to the instance variable this.age = age; // 'this.age' refers to the instance variable } public void setName(String name) { this.name = name; // 'this.name' refers to the instance variable } public String getName() { return this.name; // 'this.name' refers to the instance variable } }
In this example, the this keyword is used to resolve the ambiguity between the instance variables name and age , and the constructor parameters name and age.
2. Using this to Pass the Current Object
The this keyword can also be used to pass the current object as a parameter to another method or constructor.
2.1 Example: Passing this as a Parameter
Here is an example that demonstrates passing this as a parameter:
class Calculator { int result; Calculator add(int value) { this.result += value; return this; // returning the current object } Calculator subtract(int value) { this.result -= value; return this; } void displayResult() { System.out.println("Result: " + this.result); } } public class Main { public static void main(String[] args) { Calculator calc = new Calculator(); calc.add(10).subtract(3).displayResult(); // chaining methods using 'this' } }
In this example, this is returned from add and subtract methods, allowing method chaining.
2.2 Constructor Chaining Using this
The this keyword can be used to call one constructor from another, facilitating constructor chaining.
public class Box { private int length, width, height; public Box() { this(0, 0, 0); // calls the three-parameter constructor } public Box(int length, int width, int height) { this.length = length; this.width = width; this.height = height; } public void displayDimensions() { System.out.println("Dimensions: " + length + "x" + width + "x" + height); } }
In this example, the no-argument constructor calls the three-argument constructor using this , setting default dimensions for the Box.
3. Using this to Return the Current Object
Returning the current object using this is a common practice in method chaining.
3.1 Example: Returning this for Method Chaining
Returning this enables fluent interfaces, which are often seen in builders or APIs.
class Person { private String firstName, lastName; Person setFirstName(String firstName) { this.firstName = firstName; return this; } Person setLastName(String lastName) { this.lastName = lastName; return this; } void displayFullName() { System.out.println("Full Name: " + this.firstName + " " + this.lastName); } } public class Main { public static void main(String[] args) { Person person = new Person(); person.setFirstName("John").setLastName("Doe").displayFullName(); } }
Here, the setFirstName and setLastName methods return this , allowing for method chaining and a more fluent code style.
4. Common Mistakes and Best Practices
Misusing the this keyword can lead to errors or code that is hard to read. It's essential to understand when and why to use this.
4.1 Avoid Overusing this
While this is helpful, avoid overusing it where it's not necessary, as it can clutter your code.
4.2 Understand Context
Ensure you fully understand the context in which this is being used, especially in complex codebases where multiple objects and methods interact.
5. Conclusion
The this keyword in Java is a powerful tool for managing object-oriented code effectively. By understanding how to use this for distinguishing instance variables, passing the current object, chaining methods, and calling constructors, you can write more fluent, readable, and maintainable code.
If you have any questions or need further clarification on the this keyword, feel free to comment below!
Read posts more at : 4 Things You Should Know about the This keyword in Java.
The above is the detailed content of hings You Should Know about the This keyword in Java.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










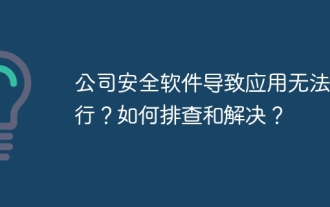
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
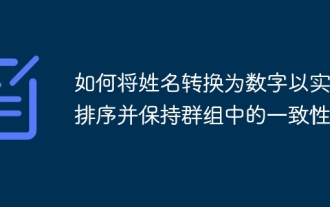
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
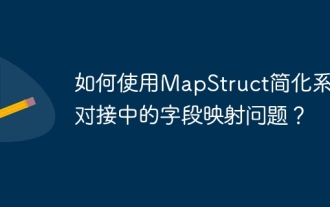
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
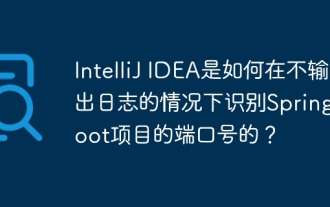
Start Spring using IntelliJIDEAUltimate version...
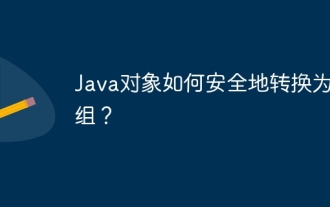
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
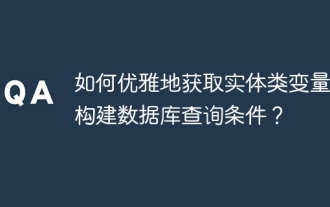
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
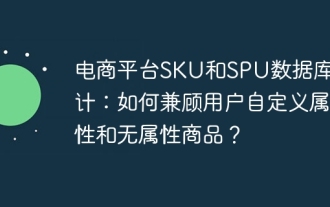
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
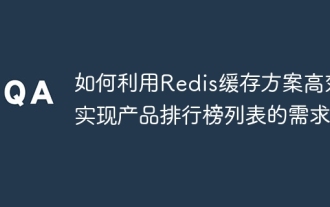
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
