NGINX with Node.js: Load Balancing, Serving Static Content, and SSL
NGINX is a powerful and versatile web server that plays a critical role in scaling Node.js applications. It is commonly used as a reverse proxy to handle load balancing, serve static content, and manage SSL termination. In this article, we’ll explore how to use NGINX in conjunction with Node.js, explaining how each of these functions works with practical examples.
Why Use NGINX with Node.js?
While Node.js excels at building scalable, event-driven applications, it may not be the most efficient way to handle tasks like load balancing, serving static content, or SSL termination. This is where NGINX comes in. NGINX is optimized for handling a large number of concurrent connections efficiently, making it the perfect companion for Node.js applications that need to scale.
Key Benefits of Using NGINX with Node.js:
- Load Balancing: NGINX can distribute traffic across multiple Node.js instances, ensuring no single server is overwhelmed.
- Serving Static Content: It efficiently serves static files (e.g., CSS, JS, images), freeing Node.js to focus on dynamic content.
- SSL Termination: NGINX handles SSL/TLS encryption and decryption, reducing the load on Node.js.
1. Load Balancing with NGINX
When scaling horizontally, you’ll need to run multiple instances of your Node.js application. NGINX can distribute incoming traffic across these instances, ensuring an even load.
Step 1: Install NGINX
On an Ubuntu system, you can install NGINX with the following command:
sudo apt update sudo apt install nginx
Step 2: NGINX Configuration for Load Balancing
The nginx.conf file is where you define how NGINX handles incoming requests. Here’s how you can set up NGINX to load balance across three Node.js instances.
http { upstream node_app { server 127.0.0.1:3000; server 127.0.0.1:3001; server 127.0.0.1:3002; } server { listen 80; location / { proxy_pass http://node_app; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; } } }
Explanation:
- The upstream block defines the pool of Node.js instances that NGINX will balance traffic between.
- The proxy_pass directive forwards requests to one of the Node.js instances.
Step 3: Start Multiple Node.js Instances
node app.js --port 3000 & node app.js --port 3001 & node app.js --port 3002 &
Step 4: Start NGINX
Once NGINX is configured, start it using:
sudo systemctl start nginx
Test the Setup:
Now, visiting your server’s IP address or domain should distribute requests among the three Node.js instances.
2. Serving Static Content with NGINX
Node.js applications often need to serve static files (like images, CSS, and JavaScript). NGINX is much more efficient at this task, as it is designed to handle large numbers of static file requests.
Step 1: NGINX Configuration for Static Files
Modify the nginx.conf file to define a location for static content.
server { listen 80; # Serve static content directly location /static/ { root /var/www/html; } # Proxy dynamic requests to Node.js location / { proxy_pass http://localhost:3000; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; } }
Explanation:
- The location /static/ block tells NGINX to serve files from /var/www/html/static/ when requests are made to /static/.
- Other requests are proxied to the Node.js application.
Step 2: Move Static Files
Move your static files (e.g., images, CSS, JavaScript) to the /var/www/html/static/ directory.
sudo mkdir -p /var/www/html/static sudo cp -r path/to/static/files/* /var/www/html/static/
Now, requests for /static resources will be handled directly by NGINX, improving the performance of your Node.js server.
3. SSL Termination with NGINX
SSL (Secure Sockets Layer) is critical for securing communication between your users and your application. NGINX can offload SSL termination, encrypting and decrypting requests, so your Node.js application doesn’t need to handle SSL itself.
Step 1: Obtain an SSL Certificate
You can obtain an SSL certificate for free using Let’s Encrypt:
sudo apt install certbot python3-certbot-nginx sudo certbot --nginx -d yourdomain.com
Step 2: NGINX SSL Configuration
Once the SSL certificate is issued, you can configure NGINX to handle SSL traffic.
server { listen 80; server_name yourdomain.com; return 301 https://$server_name$request_uri; } server { listen 443 ssl; server_name yourdomain.com; ssl_certificate /etc/letsencrypt/live/yourdomain.com/fullchain.pem; ssl_certificate_key /etc/letsencrypt/live/yourdomain.com/privkey.pem; location / { proxy_pass http://localhost:3000; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; } }
Explanation:
- The first server block redirects all HTTP traffic to HTTPS.
- The second block listens on port 443 (HTTPS), handling SSL encryption with the certificate issued by Let’s Encrypt.
Step 3: Test SSL Setup
Visit your domain (e.g., https://yourdomain.com), and your Node.js app should now be served over HTTPS.
Additional NGINX Configurations for Optimizing Node.js
1. Handling Timeouts
To prevent long-running requests from being prematurely closed, configure NGINX’s timeout settings.
server { proxy_read_timeout 90s; proxy_send_timeout 90s; send_timeout 90s; }
2. Rate Limiting
Rate limiting can help prevent abuse and manage high traffic by limiting the number of requests a user can make in a given time.
http { limit_req_zone $binary_remote_addr zone=mylimit:10m rate=1r/s; server { location / { limit_req zone=mylimit burst=5; proxy_pass http://localhost:3000; } } }
Explanation:
- The limit_req_zone directive defines a rate limit of 1 request per second.
- The burst parameter allows a small number of requests to exceed the limit before throttling kicks in.
Conclusion
NGINX is a powerful tool that can significantly enhance the performance, security, and scalability of your Node.js applications. By offloading tasks such as load balancing, serving static content, and handling SSL termination to NGINX, your Node.js server can focus on what it does best: processing dynamic content and handling real-time events.
The above is the detailed content of NGINX with Node.js: Load Balancing, Serving Static Content, and SSL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










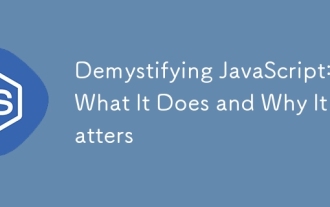
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
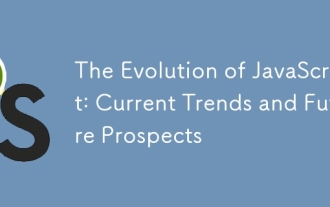
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
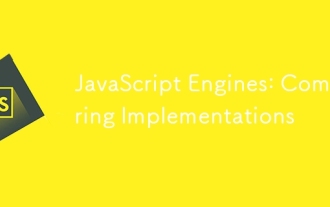
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
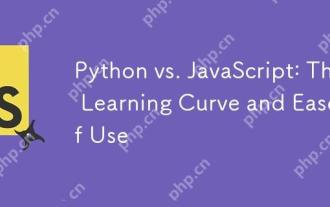
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
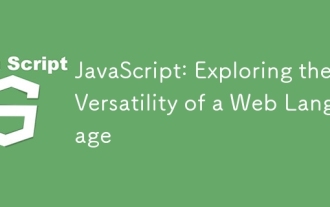
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
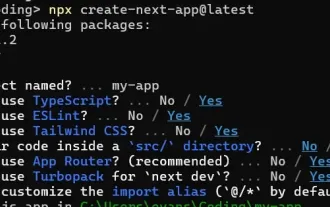
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
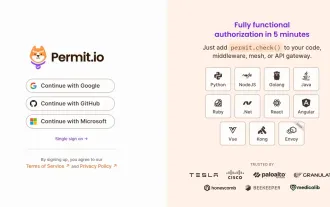
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
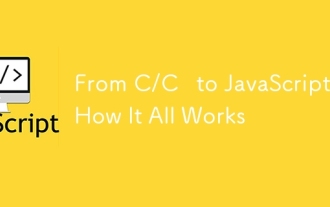
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
