


Functional vs Object-Oriented Programming in JavaScript: A Comprehensive Comparison
伟大的编程范式辩论
作为一名 JavaScript 开发人员,您可能遇到过两种主要的编程范例:函数式编程 (FP) 和面向对象编程 (OOP)。每个都有其狂热的拥护者,并且都塑造了现代 JavaScript 开发的格局。但你应该选择哪一个呢?让我们深入研究一下这种比较,揭开 JavaScript 中 FP 和 OOP 的神秘面纱。
我的范式之旅
在我作为 JavaScript 开发人员的职业生涯中,我有机会使用 FP 和 OOP 方法来处理项目。我记得在一个特定的项目中,我们重构了一个大型 OOP 代码库以纳入更多功能概念。这个过程充满挑战,但很有启发性,展示了两种范式在现实场景中的优点和缺点。
理解核心理念
函数式编程:纯粹的方法
函数式编程就是通过编写纯函数来编写程序,避免共享状态、可变数据和副作用。它是声明性的而不是命令性的,专注于解决什么而不是如何解决。
关键概念:
- 纯函数
- 不变性
- 函数组合
- 高阶函数
面向对象编程:对象的世界
面向对象编程围绕数据或对象组织软件设计,而不是函数和逻辑。它基于包含数据和代码的对象的概念。
关键概念:
- 封装
- 继承
- 多态性
- 抽象
对决:JavaScript 中的 FP 与 OOP
让我们从各个方面比较这些范例:
-
状态管理
- FP:强调不可变状态。数据转换返回新数据。
- OOP:使用可变状态,通常封装在对象内。
// FP Approach const addToCart = (cart, item) => [...cart, item]; // OOP Approach class ShoppingCart { constructor() { this.items = []; } addItem(item) { this.items.push(item); } }
-
代码组织
- FP:将代码组织成对数据进行操作的纯函数。
- OOP:将代码组织成组合数据和方法的对象。
-
继承与组合
- FP:倾向于通过功能组合进行组合。
- OOP:严重依赖继承来实现代码重用。
// FP Composition const withLogging = (wrappedFunction) => { return (...args) => { console.log(`Calling function with arguments: ${args}`); return wrappedFunction(...args); }; }; const add = (a, b) => a + b; const loggedAdd = withLogging(add); // OOP Inheritance class Animal { makeSound() { console.log("Some generic animal sound"); } } class Dog extends Animal { makeSound() { console.log("Woof!"); } }
-
副作用
- FP:旨在最大限度地减少副作用以实现可预测性。
- OOP:当对象交互并修改彼此的状态时,副作用很常见。
-
易于测试
- FP:纯函数很容易测试,因为它们总是针对给定的输入产生相同的输出。
- OOP:由于状态变化和对象之间的依赖关系,测试可能会更加复杂。
何时选择每个范式
函数式编程在以下情况下大放异彩:
- 您需要高度可预测和可测试的代码。
- 您的应用程序处理数据转换而不改变状态。
- 您正在并行或并发系统上工作。
- 您正在构建需要高可靠性的复杂系统。
面向对象编程在以下情况下表现出色:
- 您正在使用属性和行为对现实世界的对象进行建模。
- 您需要在对象的整个生命周期中维护复杂的状态。
- 您正在开发受益于封装和继承的大型系统。
- 您的团队更熟悉 OOP 概念和模式。
混合方法:两全其美
在实践中,许多 JavaScript 开发人员使用混合方法,结合了两种范式的元素。现代 JavaScript 和 React 等框架鼓励更函数式的风格,同时在有意义的情况下仍然允许面向对象的概念。
// Hybrid Approach Example class UserService { constructor(apiClient) { this.apiClient = apiClient; } async getUsers() { const users = await this.apiClient.fetchUsers(); return users.map(user => ({ ...user, fullName: `${user.firstName} ${user.lastName}` })); } } const processUsers = (users) => { return users.filter(user => user.age > 18) .sort((a, b) => a.fullName.localeCompare(b.fullName)); }; // Usage const userService = new UserService(new ApiClient()); const users = await userService.getUsers(); const processedUsers = processUsers(users);
结论:扩展您的 JavaScript 工具包
了解函数式编程和面向对象编程可以扩展您在 JavaScript 中解决问题的工具包。每种范例都有其优点,最优秀的开发人员知道如何利用两者。
记住:
- FP offers cleaner, more predictable code, especially for data transformation.
- OOP provides a natural way to model entities with state and behavior.
- A hybrid approach often leads to the most effective and maintainable code.
As you continue your JavaScript journey, experiment with both approaches. The key is to understand the strengths of each paradigm and apply them where they make the most sense in your projects.
Keep learning, keep coding, and most importantly, keep exploring new ways to make your JavaScript more elegant and efficient!
Further Reading
- Functional Programming in JavaScript
- Object-Oriented JavaScript
- Functional vs OOP Programming
The above is the detailed content of Functional vs Object-Oriented Programming in JavaScript: A Comprehensive Comparison. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


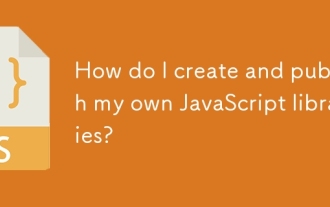
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
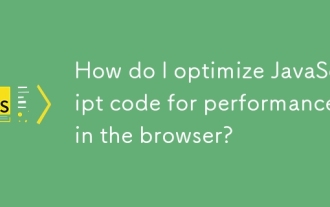
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
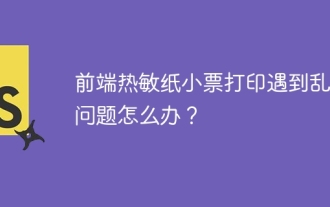
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
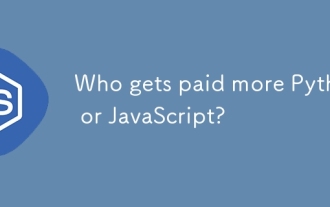
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
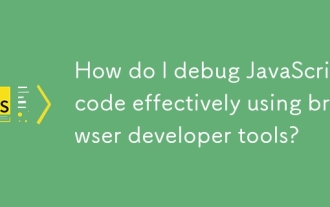
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
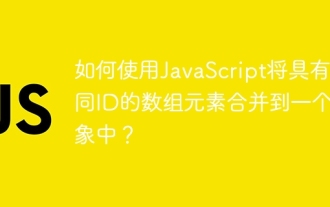
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
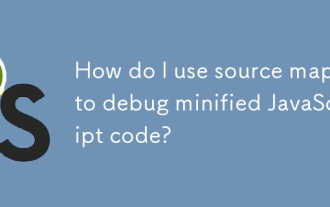
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
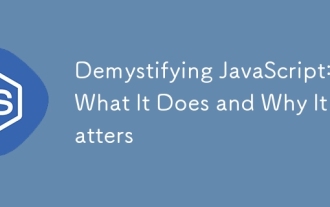
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
