Unlock the Power of JavaScript: Pro Tips and Techniques
1. Use destructuring for swapping variables
let a = 1, b = 2; [a, b] = [b, a]; console.log(a, b); // 2 1
Why: Provides a clean, one-line way to swap variable values without a temporary variable.
2. Use template literals for string interpolation
const name = "Alice"; console.log(`Hello, ${name}!`); // Hello, Alice!
Why: Makes string concatenation more readable and less error-prone than traditional methods.
3. Use the nullish coalescing operator (??) for default values
const value = null; const defaultValue = value ?? "Default"; console.log(defaultValue); // "Default"
Why: Provides a concise way to handle null or undefined values, distinguishing from falsy values like 0 or empty string.
4. Use optional chaining (?.) for safe property access
const obj = { nested: { property: "value" } }; console.log(obj?.nested?.property); // "value" console.log(obj?.nonexistent?.property); // undefined
Why: Prevents errors when accessing nested properties that might not exist, reducing the need for verbose checks.
5. Use the spread operator (...) for array manipulation
const arr1 = [1, 2, 3]; const arr2 = [4, 5, 6]; const combined = [...arr1, ...arr2]; console.log(combined); // [1, 2, 3, 4, 5, 6]
Why: Simplifies array operations like combining, copying, or adding elements, making code more concise and readable.
6. Use Array.from() to create arrays from array-like objects
const arrayLike = { 0: "a", 1: "b", 2: "c", length: 3 }; const newArray = Array.from(arrayLike); console.log(newArray); // ["a", "b", "c"]
Why: Easily converts array-like objects or iterables into true arrays, enabling use of array methods.
7. Use Object.entries() for easy object iteration
const obj = { a: 1, b: 2, c: 3 }; for (const [key, value] of Object.entries(obj)) { console.log(`${key}: ${value}`); }
Why: Provides a clean way to iterate over both keys and values of an object simultaneously.
8. Use Array.prototype.flat() to flatten nested arrays
const nestedArray = [1, [2, 3, [4, 5]]]; console.log(nestedArray.flat(2)); // [1, 2, 3, 4, 5]
Why: Simplifies working with nested arrays by flattening them to a specified depth.
9. Use async/await for cleaner asynchronous code
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error:', error); } }
Why: Makes asynchronous code look and behave more like synchronous code, improving readability and error handling.
10. Use Set for unique values in an array
const numbers = [1, 2, 2, 3, 4, 4, 5]; const uniqueNumbers = [...new Set(numbers)]; console.log(uniqueNumbers); // [1, 2, 3, 4, 5]
Why: Provides an efficient way to remove duplicates from an array without manual looping.
11. Use Object.freeze() to create immutable objects
const frozenObj = Object.freeze({ prop: 42 }); frozenObj.prop = 100; // Fails silently in non-strict mode console.log(frozenObj.prop); // 42
Why: Prevents modifications to an object, useful for creating constants or ensuring data integrity.
12. Use Array.prototype.reduce() for powerful array transformations
const numbers = [1, 2, 3, 4, 5]; const sum = numbers.reduce((acc, curr) => acc + curr, 0); console.log(sum); // 15
Why: Allows complex array operations to be performed in a single pass, often more efficiently than loops.
13. Use the logical AND operator (&&) for conditional execution
const isTrue = true; isTrue && console.log("This will be logged");
Why: Provides a short way to execute code only if a condition is true, without an explicit if statement.
14. Use Object.assign() to merge objects
const obj1 = { a: 1, b: 2 }; const obj2 = { b: 3, c: 4 }; const merged = Object.assign({}, obj1, obj2); console.log(merged); // { a: 1, b: 3, c: 4 }
Why: Simplifies object merging, useful for combining configuration objects or creating object copies with overrides.
15. Use Array.prototype.some() and Array.prototype.every() for array
checking const numbers = [1, 2, 3, 4, 5]; console.log(numbers.some(n => n > 3)); // true console.log(numbers.every(n => n > 0)); // true
Why: Provides concise ways to check if any or all elements in an array meet a condition, avoiding explicit loops.
16. Use console.table() for better logging of tabular data
const users = [ { name: "John", age: 30 }, { name: "Jane", age: 28 }, ]; console.table(users);
Why: Improves readability of logged data in tabular format, especially useful for arrays of objects.
17. Use Array.prototype.find() to get the first matching element
const numbers = [1, 2, 3, 4, 5]; const found = numbers.find(n => n > 3); console.log(found); // 4
Why: Efficiently finds the first element in an array that satisfies a condition, stopping iteration once found.
18. Use Object.keys(), Object.values(), and Object.entries() for object
manipulation const obj = { a: 1, b: 2, c: 3 }; console.log(Object.keys(obj)); // ["a", "b", "c"] console.log(Object.values(obj)); // [1, 2, 3] console.log(Object.entries(obj)); // [["a", 1], ["b", 2], ["c", 3]]
Why: Provides easy ways to extract and work with object properties and values, useful for many object operations.
19. Use the Intl API for internationalization
const number = 123456.789; console.log(new Intl.NumberFormat('de-DE').format(number)); // 123.456,789
Why: Simplifies formatting of numbers, dates, and strings according to locale-specific rules without manual implementation.
20. Use Array.prototype.flatMap() for mapping and flattening in one step
const sentences = ["Hello world", "How are you"]; const words = sentences.flatMap(sentence => sentence.split(" ")); console.log(words); // ["Hello", "world", "How", "are", "you"]
Why: Combines mapping and flattening operations efficiently, useful for transformations that produce nested results.
The above is the detailed content of Unlock the Power of JavaScript: Pro Tips and Techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










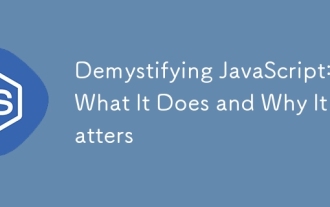
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
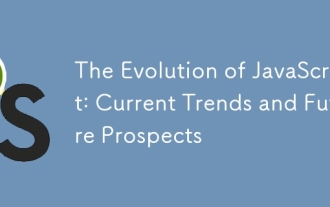
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
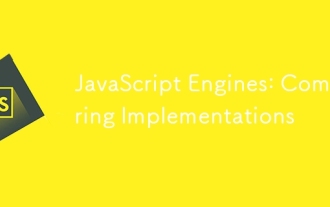
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
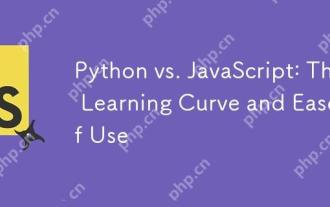
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
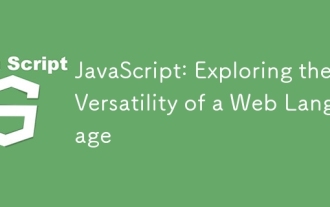
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
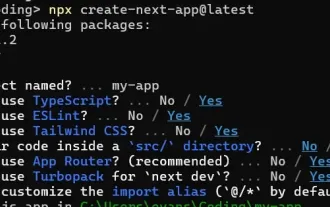
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
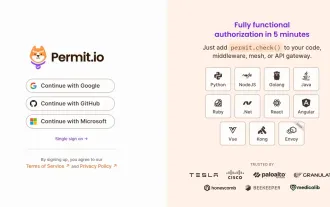
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
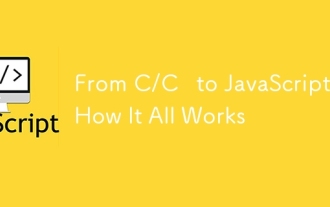
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
