


Coding Challenges: Engage and Learn Through Problem Solving
Coding Challenges: Engage and Learn Through Problem Solving
Coding challenges are a fantastic way to improve your programming skills, engage with a community, and learn new techniques. In this blog post, we'll present a coding challenge, discuss various approaches to solve it, and invite readers to share their solutions in the comments. Let's dive in!
Challenge: Find the Longest Palindromic Substring
Problem:
Given a string s, find the longest palindromic substring in s. A palindrome is a string that reads the same backward as forward.
Example:
Input: s = "babad" Output: "bab" Note: "aba" is also a valid answer.
Constraints:
- 1 <= s.length <= 1000
- s consists of only digits and English letters.
Please share your Solutions with creative approaches using different programming languages.
Step-by-Step Guide to Solve the Challenge
Step 1: Understand the Problem
Before jumping into the code, make sure you understand the problem. A palindromic substring is a sequence of characters that reads the same backward as forward. Our goal is to find the longest such substring in the given string s.
Step 2: Plan Your Approach
There are several ways to solve this problem. We'll discuss three approaches:
- Brute Force
- Expand Around Center
- Dynamic Programming
Step 3: Implement the Brute Force Approach
The brute force approach involves checking all possible substrings and determining if they are palindromes. This method is straightforward but not efficient for large strings.
def longest_palindrome_brute_force(s): def is_palindrome(sub): return sub == sub[::-1] n = len(s) longest = "" for i in range(n): for j in range(i, n): if is_palindrome(s[i:j+1]) and len(s[i:j+1]) > len(longest): longest = s[i:j+1] return longest print(longest_palindrome_brute_force("babad")) # Output: "bab" or "aba" <h4> Step 4: Implement the Expand Around Center Approach </h4> <p>This approach involves expanding around each character (and between characters) to find the longest palindrome. It's more efficient than brute force.<br> </p> <pre class="brush:php;toolbar:false">def longest_palindrome_expand_center(s): def expand_around_center(left, right): while left >= 0 and right < len(s) and s[left] == s[right]: left -= 1 right += 1 return s[left+1:right] longest = "" for i in range(len(s)): # Odd length palindromes odd_palindrome = expand_around_center(i, i) if len(odd_palindrome) > len(longest): longest = odd_palindrome # Even length palindromes even_palindrome = expand_around_center(i, i+1) if len(even_palindrome) > len(longest): longest = even_palindrome return longest print(longest_palindrome_expand_center("babad")) # Output: "bab" or "aba"
Step 5: Implement the Dynamic Programming Approach
The dynamic programming approach uses a table to store whether a substring is a palindrome, leading to an efficient solution.
def longest_palindrome_dp(s): n = len(s) if n == 0: return "" dp = [[False] * n for _ in range(n)] start, max_length = 0, 1 for i in range(n): dp[i][i] = True for length in range(2, n+1): for i in range(n-length+1): j = i + length - 1 if s[i] == s[j]: if length == 2 or dp[i+1][j-1]: dp[i][j] = True if length > max_length: start = i max_length = length return s[start:start+max_length] print(longest_palindrome_dp("babad")) # Output: "bab" or "aba"
Try optimizing the algorithms.
The above is the detailed content of Coding Challenges: Engage and Learn Through Problem Solving. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


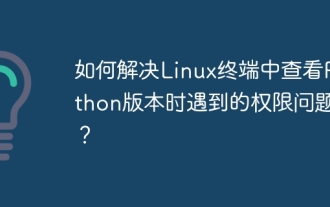
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
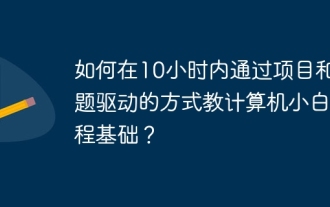
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
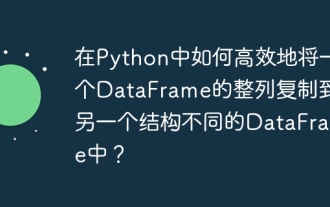
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
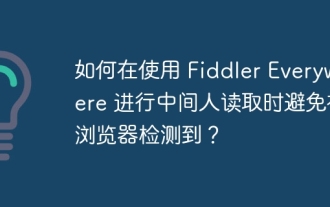
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
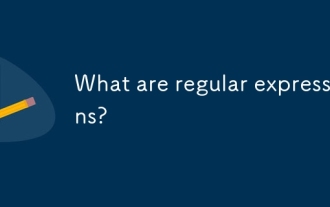
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
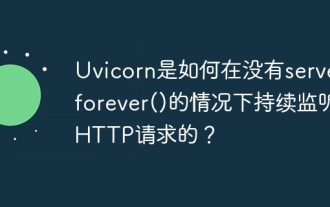
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
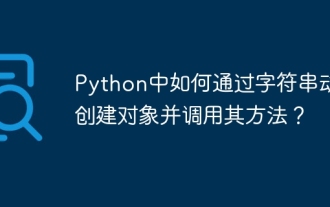
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
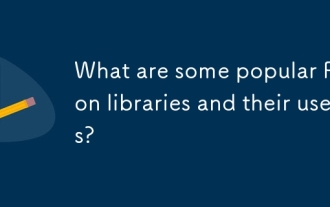
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
