


PART# Efficient File Transfer System Using HTTP for Large Datasets
Let's break down the provided HTML, PHP, JavaScript, and CSS code for a chunked file upload dashboard part by part.
HTML Code:
Structure Overview:
-
Bootstrap for Layout: The code uses Bootstrap 4.5.2 to create a responsive layout with two main sections:
- Chunked Uploads Section: For uploading large files in chunks.
- Downloads Section: For listing and downloading previously uploaded files.
Key Elements:
- : This input allows multiple file selection.
- : Placeholder for displaying upload progress.
- : Placeholder to show selected files before uploading.
- : Button to initiate the upload process.
- : Button to fetch and display the list of downloadable files.
PHP Code (chunked-file-upload.php):
This file handles the server-side logic of uploading files in chunks.
Key Parts:
-
Receive Chunk and Metadata:
- $_FILES['fileChunk']: Receives the chunk of the file being uploaded.
- $_POST['chunkIndex']: The index of the current chunk.
- $_POST['totalChunks']: The total number of chunks for the file.
-
Chunk Storage:
- $chunkFilePath = $targetDir . "$fileName.part$chunkIndex": Each chunk is saved as a separate .part file until the final file is assembled.
-
Merging Chunks:
- if ($chunkIndex == $totalChunks - 1): When the last chunk is uploaded, the script merges all chunks into the final file.
-
Return Response:
- A JSON response is returned to the client with the upload status and file details.
Why use chunking?
- Large files are broken into smaller chunks to prevent timeouts and memory issues, making the upload process more reliable.
PHP Code (download.php):
Handles file downloads.
Key Parts:
- $_GET['file']: Retrieves the file name from the URL query string.
-
File Path Construction:
- $filePath = $targetDir . $fileName: Constructs the full path of the file.
-
File Download:
- readfile($filePath): Sends the file to the client as a downloadable stream.
Why use it?
- Enables users to download files directly from the server. The server sends proper headers to prompt the browser to download the file rather than displaying it.
PHP Code (get_files.php):
Lists all uploaded files available for download.
Key Parts:
- scandir($targetDir): Scans the directory for all uploaded files.
- array_diff(): Filters out . and .. from the list of files.
- echo json_encode(array_values($files)): Returns the file list as a JSON array.
Why use it?
- Provides a dynamic list of files available for download, which is updated on each request.
JavaScript Code (chunked-file-upload.js):
Handles the client-side logic of chunked file uploads and file downloads.
Key Parts:
-
Drag and Drop:
- preventDefaults(e): Prevents the browser from opening files when dropped.
- handleDrop(e): Handles dropped files and displays them.
-
Display File List:
- displayFileList(files): Shows the selected files with their sizes and statuses in the file list before upload.
-
Uploading Files in Chunks:
- for (let file of files): Loops through each selected file.
- const chunk = file.slice(start, end): Slices a portion of the file to upload as a chunk.
- uploadChunk(): Recursively uploads each chunk, updates the progress bar and status, and merges the chunks on the server.
-
Download Files:
- $.ajax({url: './src/get_files.php'}): Sends an AJAX request to get the list of uploaded files.
- downloadFile(fileName): Initiates the download by redirecting the user to download.php.
Why use chunked upload in JS?
- For large files, uploading in smaller chunks ensures the process continues even if one chunk fails. It improves reliability by allowing chunk-by-chunk retries.
CSS Code (chunked-file-upload.css):
Key Styles:
-
#drop-area:
- Dashed Border: Visually indicates the area where users can drop files.
- Highlight Effect: The border color changes when a file is dragged over.
-
Progress Bar:
- #progress-bar: The width dynamically updates to reflect the upload progress.
-
File List:
- #file-list .file-status: Adds spacing and styles to display the file name, size, and status.
Why style these elements?
- A user-friendly interface helps users understand what actions they can perform (drag-and-drop, file selection) and gives feedback on the status of their uploads/downloads.
Conclusion:
This system allows large files to be uploaded reliably in chunks and provides users with feedback through a progress bar and status updates. Additionally, the download section gives users the ability to download uploaded files. Combining Bootstrap, JavaScript, and PHP ensures that the system is both user-friendly and functional.
Connecting Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
- GitHub
Source Code
The above is the detailed content of PART# Efficient File Transfer System Using HTTP for Large Datasets. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










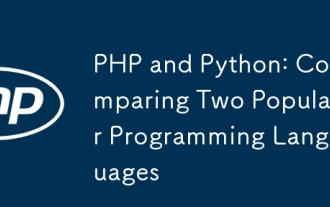
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
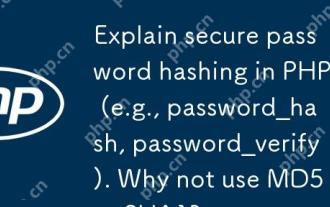
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
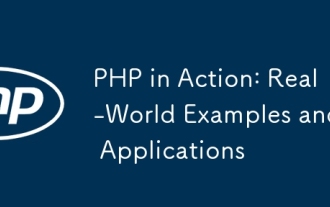
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
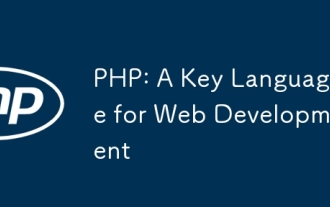
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
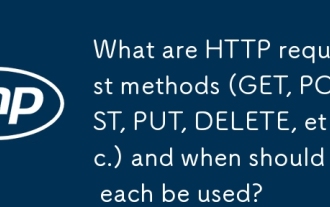
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
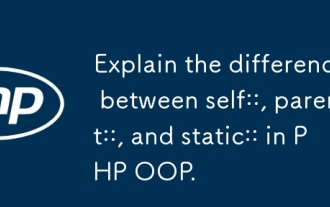
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
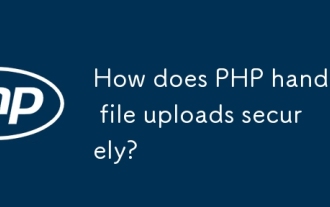
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
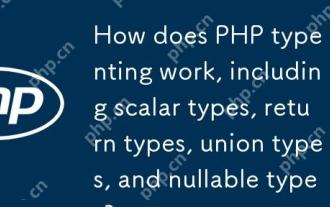
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
