Enhancing React Applications with GraphQL Over REST APIs
在快速變化的 Web 開發世界中,最佳化和擴展應用程式始終是一個問題。 React.js 作為一種工具在前端開發方面取得了非凡的成功,它提供了一種創建使用者介面的強大方法。但隨著應用程式的不斷增長,情況會變得複雜,特別是當涉及多個 REST API 端點時。諸如過度獲取之類的問題,其中超出所需的數據可能會成為效能瓶頸和糟糕的用戶體驗的根源。
這些挑戰的解決方案之一是在 React 應用程式中使用 GraphQL。如果您的後端有多個 REST 端點,那麼引入在內部調用 REST API 端點的 GraphQL 層可以增強您的應用程序,防止過度獲取並簡化您的前端應用程式。在本文中,您將了解如何使用它、這種方法的優點和缺點、各種挑戰;以及如何解決這些問題。我們也將深入探討一些實際範例,說明 GraphQL 如何協助您改善資料處理方式。
REST API 中的過度獲取
在 REST API 中,當 API 傳遞給客戶端的資料量超過客戶端所需的資料量時,就會發生過度取得。這是 REST API 的常見問題,它通常會傳回固定的物件或回應架構。為了更好地理解這個問題,讓我們考慮一個例子。
考慮一個使用者個人資料頁面,其中只需要顯示使用者的姓名和電子郵件。使用典型的 REST API,取得使用者資料可能如下所示:
fetch('/api/users/1') .then(response => response.json()) .then(user => { // Use the user's name and profilePicture in the UI });
API 回應將包含不必要的資料:
{ "id": 1, "name": "John Doe", "profilePicture": "/images/john.jpg", "email": "john@example.com", "address": "123 Denver St", "phone": "111-555-1234", "preferences": { "newsletter": true, "notifications": true }, // ...more details }
雖然應用程式只需要使用者的姓名和電子郵件字段,但 API 會傳回整個使用者物件。這些額外的資料通常會增加有效負載大小,佔用更多頻寬,並且在資源有限或網路連接速度慢的裝置上使用時最終會減慢應用程式的速度。
GraphQL 作為解決方案
GraphQL 透過允許客戶端準確請求他們需要的資料來解決過度獲取問題。透過將 GraphQL 伺服器整合到您的應用程式中,您可以建立一個靈活且有效率的資料擷取層,與您現有的 REST API 進行通訊。
它是如何運作的
- GraphQL 伺服器設定:您引入了一個 GraphQL 伺服器,它充當 React 前端和 REST API 之間的中介。
- 架構定義:您定義一個 GraphQL 架構,指定前端所需的資料類型和查詢。
- 解析器實作:您在 GraphQL 伺服器中實作解析器,從 REST API 取得資料並僅傳回必要的欄位。
- 前端整合:您更新 React 應用程式以使用 GraphQL 查詢,而不是直接 REST API 呼叫。
這種方法可讓您最佳化資料獲取,而無需徹底檢修現有的後端基礎設施。
在 React 應用程式中實作 GraphQL
讓我們看看如何設定 GraphQL 伺服器並將其整合到 React 應用程式中。
安裝依賴項:
npm install apollo-server graphql axios
定義架構
建立一個名為 schema.js 的檔案:
const { gql } = require('apollo-server'); const typeDefs = gql` type User { id: ID! name: String email: String // Ensure this matches exactly with the frontend query } type Query { user(id: ID!): User } `; module.exports = typeDefs;
此架構定義了一個使用者類型和一個透過 ID 取得使用者的使用者查詢。
實作解析器
建立一個名為resolvers.js的檔案:
const resolvers = { Query: { user: async (_, { id }) => { try { const response = await fetch(`https://jsonplaceholder.typicode.com/users/${id}`); const user = await response.json(); return { id: user.id, name: user.name, email: user.email, // Return email instead of profilePicture }; } catch (error) { throw new Error(`Failed to fetch user: ${error.message}`); } }, }, }; module.exports = resolvers;
使用者查詢的解析器從 REST API 取得資料並僅傳回必填欄位。
我們將使用 https://jsonplaceholder.typicode.com/ 作為我們的假 REST API。
設定伺服器
建立 server.js 檔案:
const { ApolloServer } = require('apollo-server'); const typeDefs = require('./schema'); const resolvers = require('./resolvers'); const server = new ApolloServer({ typeDefs, resolvers, }); server.listen({ port: 4000 }).then(({ url }) => { console.log(`GraphQL Server ready at ${url}`); });
啟動伺服器:
node server.js
您的 GraphQL 伺服器位於 http://localhost:4000/graphql,如果您查詢您的伺服器,它將帶您進入此頁面。
與 React 應用程式集成
我們現在將更改 React 應用程式以使用 GraphQL API。
安裝 Apollo 客戶端
npm install @apollo/client graphql
配置 Apollo 客戶端
import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'http://localhost:4000', cache: new InMemoryCache(), });
撰寫 GraphQL 查詢
const GET_USER = gql` query GetUser($id: ID!) { user(id: $id) { id name email } } `;
現在將上述程式碼片段與您的 React 應用程式整合。下面是一個簡單的 React 應用程序,它允許用戶選擇 userId 並顯示資訊:
import { useState } from 'react'; import { ApolloClient, InMemoryCache, ApolloProvider, gql, useQuery } from '@apollo/client'; import './App.css'; // Link to the updated CSS const client = new ApolloClient({ uri: 'http://localhost:4000', // Ensure this is the correct URL for your GraphQL server cache: new InMemoryCache(), }); const GET_USER = gql` query GetUser($id: ID!) { user(id: $id) { id name email } } `; const User = ({ userId }) => { const { loading, error, data } = useQuery(GET_USER, { variables: { id: userId }, }); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <div className="user-container"> <h2>{data.user.name}</h2> <p>Email: {data.user.email}</p> </div> ); }; const App = () => { const [selectedUserId, setSelectedUserId] = useState("1"); return ( <ApolloProvider client={client}> <div className="app-container"> <h1 className="title">GraphQL User Lookup</h1> <div className="dropdown-container"> <label htmlFor="userSelect">Select User ID:</label> <select id="userSelect" value={selectedUserId} onChange={(e) => setSelectedUserId(e.target.value)} > {Array.from({ length: 10 }, (_, index) => ( <option key={index + 1} value={index + 1}> {index + 1} </option> ))} </select> </div> <User userId={selectedUserId} /> </div> </ApolloProvider> ); }; export default App;
結果:
簡單使用者
Working with Multiple Endpoints
Imagine a scenario where you need to retrieve a specific user’s posts, along with the individual comments on each post. Instead of making three separate API calls from your frontend React app and dealing with unnecessary data, you can streamline the process with GraphQL. By defining a schema and crafting a GraphQL query, you can request only the exact data your UI requires, all in one efficient request.
We need to fetch user data, their posts, and comments for each post from the different endpoints. We’ll use fetch to gather data from the multiple endpoints and return it via GraphQL.
Update Resolvers
const fetch = require('node-fetch'); const resolvers = { Query: { user: async (_, { id }) => { try { // fetch user const userResponse = await fetch(`https://jsonplaceholder.typicode.com/users/${id}`); const user = await userResponse.json(); // fetch posts for a user const postsResponse = await fetch(`https://jsonplaceholder.typicode.com/posts?userId=${id}`); const posts = await postsResponse.json(); // fetch comments for a post const postsWithComments = await Promise.all( posts.map(async (post) => { const commentsResponse = await fetch(`https://jsonplaceholder.typicode.com/comments?postId=${post.id}`); const comments = await commentsResponse.json(); return { ...post, comments }; }) ); return { id: user.id, name: user.name, email: user.email, posts: postsWithComments, }; } catch (error) { throw new Error(`Failed to fetch user data: ${error.message}`); } }, }, }; module.exports = resolvers;
Update GraphQL Schema
const { gql } = require('apollo-server'); const typeDefs = gql` type Comment { id: ID! name: String email: String body: String } type Post { id: ID! title: String body: String comments: [Comment] } type User { id: ID! name: String email: String posts: [Post] } type Query { user(id: ID!): User } `; module.exports = typeDefs;
Server setup in server.js remains same. Once we update the React.js code, we get the below output:
Detailed User
Benefits of This Approach
Integrating GraphQL into your React application provides several advantages:
Eliminating Overfetching
A key feature of GraphQL is that it only fetches exactly what you request. The server only returns the requested fields and ensures that the amount of data transferred over the network is reduced by serving only what the query demands and thus improving performance.
Simplifying Frontend Code
GraphQL enables you to get the needful information in a single query regardless of their origin. Internally it could be making 3 API calls to get the information. This helps to simplify your frontend code because now you don’t need to orchestrate different async requests and combine their results.
Improving Developer’s Experience
A strong typing and schema introspection offer better tooling and error checking than in the traditional API implementation. Further to that, there are interactive environments where developers can build and test queries, including GraphiQL or Apollo Explorer.
Addressing Complexities and Challenges
This approach has some advantages but it also introduces some challenges that have to be managed.
Additional Backend Layer
The introduction of the GraphQL server creates an extra layer in your backend architecture and if not managed properly, it becomes a single point of failure.
Solution: Pay attention to error handling and monitoring. Containerization and orchestration tools like Docker and Kubernetes can help manage scalability and reliability.
Potential Performance Overhead
The GraphQL server may make multiple REST API calls to resolve a single query, which can introduce latency and overhead to the system.
Solution: Cache the results to avoid making several calls to the API. There exist some tools such as DataLoader which can handle the process of batching and caching of requests.
Conclusion
"Simplicity is the ultimate sophistication" — Leonardo da Vinci
Integrating GraphQL into your React application is more than just a performance optimization — it’s a strategic move towards building more maintainable, scalable, and efficient applications. By addressing overfetching and simplifying data management, you not only enhance the user experience but also empower your development team with better tools and practices.
While the introduction of a GraphQL layer comes with its own set of challenges, the benefits often outweigh the complexities. By carefully planning your implementation, optimizing your resolvers, and securing your endpoints, you can mitigate potential drawbacks. Moreover, the flexibility that GraphQL offers can future-proof your application as it grows and evolves.
Embracing GraphQL doesn’t mean abandoning your existing REST APIs. Instead, it allows you to leverage their strengths while providing a more efficient and flexible data access layer for your frontend applications. This hybrid approach combines the reliability of REST with the agility of GraphQL, giving you the best of both worlds.
If you’re ready to take your React application to the next level, consider integrating GraphQL into your data fetching strategy. The journey might present challenges, but the rewards — a smoother development process, happier developers, and satisfied users — make it a worthwhile endeavor.
Full Code Available
You can find the full code for this implementation on my GitHub repository: GitHub Link.
The above is the detailed content of Enhancing React Applications with GraphQL Over REST APIs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










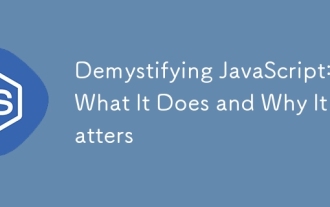
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
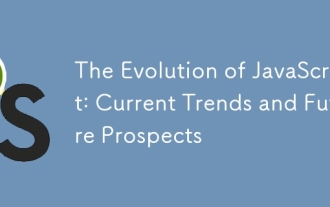
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
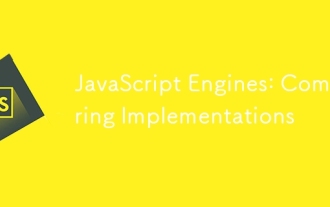
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
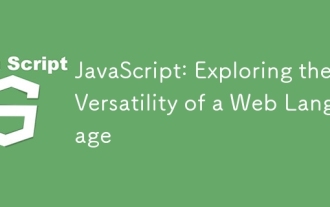
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
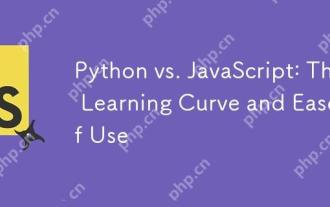
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
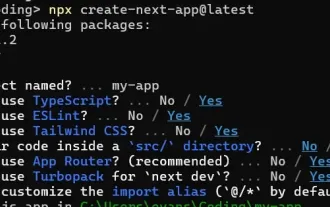
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
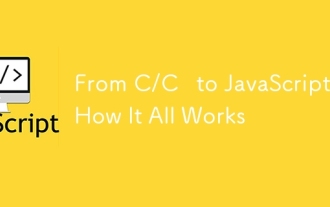
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
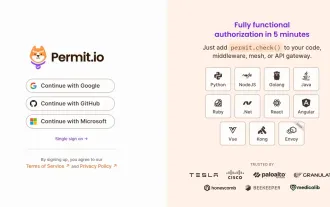
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
