Comparison between ECMAScript ESand ECMAScript ES6)
1. Declaration of Variables
ES5:
- Use of var to declare variables. It has function as a scope and can give rise to hoisting.
var nombre = 'Maria';
ES6:
- Introduces let and const that have block as scope, improving security in the handling of variables.
let nombre = 'Maria'; // Variable que puede cambiar const edad = 30; // Constante, no puede cambiar
2. Arrow Functions
ES5:
- Traditional functions require more code and your handling of this can be confusing.
var suma = function(a, b) { return a + b; };
ES6:
- Arrow functions are more concise and do not change the context of this.
const suma = (a, b) => a + b;
3. Template Strings
ES5:
- String concatenation is done using the . operator
var saludo = 'Hola ' + nombre + ', tienes ' + edad + ' años.';
ES6:
- backticks (`) are used to create string templates, allowing interpolation.
const saludo = `Hola ${nombre}, tienes ${edad} años.`;
4. Default Parameters
ES5:
- There was no support for default parameters, so it was implemented manually.
function saludo(nombre) { nombre = nombre || 'Invitado'; return 'Hola ' + nombre; }
ES6:
- The default parameters are declared directly in the function signature.
function saludo(nombre = 'Invitado') { return `Hola ${nombre}`; }
5. Classes
ES5:
- The concept of classes did not exist. Builder functions and prototypes were used.
function Persona(nombre, edad) { this.nombre = nombre; this.edad = edad; } Persona.prototype.saludar = function() { return 'Hola, soy ' + this.nombre; };
ES6:
- The classes are introduced, a cleaner syntax closer to other programming languages.
class Persona { constructor(nombre, edad) { this.nombre = nombre; this.edad = edad; } saludar() { return `Hola, soy ${this.nombre}`; } }
6. Modules (Import and Export)
ES5:
- There was no native support for modules. Libraries such as RequireJS or CommonJS were used.
// CommonJS var modulo = require('modulo'); module.exports = modulo;
ES6:
- Introduces native support for modules with import and export.
// Exportar export const suma = (a, b) => a + b; // Importar import { suma } from './modulo';
7. Promises
ES5:
- There was no native promise handling. Callbacks were relied on to handle asynchrony, leading to problems like "Callback Hell".
function hacerAlgo(callback) { setTimeout(function() { callback('Hecho'); }, 1000); } hacerAlgo(function(resultado) { console.log(resultado); });
ES6:
- promises are introduced to handle asynchronous operations more cleanly.
const hacerAlgo = () => { return new Promise((resolve, reject) => { setTimeout(() => resolve('Hecho'), 1000); }); }; hacerAlgo().then(resultado => console.log(resultado));
8. Rest and Spread Operator
ES5:
- There was no support for easily combining or separating arrays or objects. Techniques such as the use of apply were used.
function sumar(a, b, c) { return a + b + c; } var numeros = [1, 2, 3]; sumar.apply(null, numeros);
ES6:
- The rest and spread operators are introduced for easier handling of argument lists and arrays.
// Spread const numeros = [1, 2, 3]; const resultado = sumar(...numeros); // Rest function sumar(...numeros) { return numeros.reduce((a, b) => a + b, 0); }
9. Destructuring
ES5:
- Extracting values from objects or arrays was manual and error-prone.
var persona = { nombre: 'Maria', edad: 30 }; var nombre = persona.nombre; var edad = persona.edad;
ES6:
- destructuring is introduced to extract values from objects and arrays in a cleaner way.
const { nombre, edad } = persona;
Conclusion
ECMAScript 6 (ES6) brings a large number of syntactic and functional improvements that simplify development in JavaScript, making it more readable, maintainable and efficient compared to ECMAScript 5 (ES5).
The above is the detailed content of Comparison between ECMAScript ESand ECMAScript ES6). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










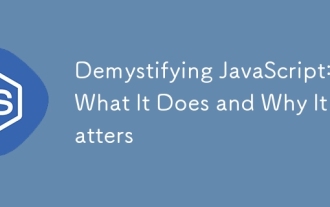
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
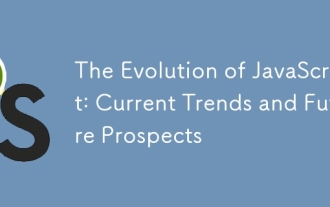
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
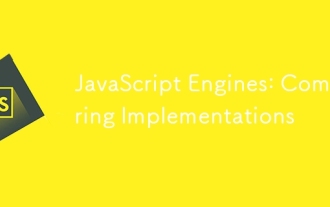
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
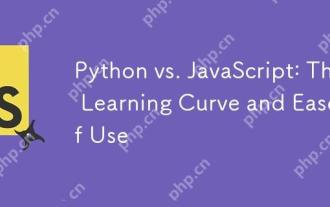
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
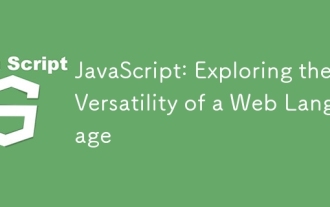
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
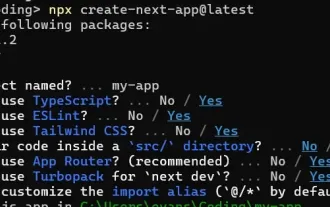
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
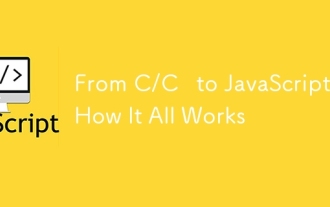
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
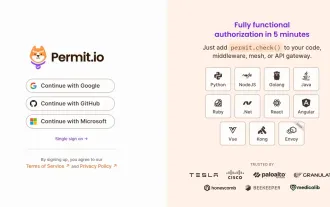
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
