Creating an LLM for testing with tensorflow in Python
Hi,
I want to test a small LLM program and I decided to do it with tensorflow .
My source code is available in https://github.com/victordalet/first_llm
I - Requirements
You need to install tensorflow and numpy
pip install 'numpy<2' pip install tensorflow
II - Create Dataset
You need to make a data string array to countain a small dataset, for example I create :
data = [ "Salut comment ca va", "Je suis en train de coder", "Le machine learning est une branche de l'intelligence artificielle", "Le deep learning est une branche du machine learning", ]
You can find a dataset on kaggle if you're not inspired.
III - Build model and train it
To do this, I create a small LLM class with the various methods.
class LLM: def __init__(self): self.model = None self.max_sequence_length = None self.input_sequences = None self.total_words = None self.tokenizer = None self.tokenize() self.create_input_sequences() self.create_model() self.train() test_sentence = "Pour moi le machine learning est" print(self.test(test_sentence, 10)) def tokenize(self): self.tokenizer = Tokenizer() self.tokenizer.fit_on_texts(data) self.total_words = len(self.tokenizer.word_index) + 1 def create_input_sequences(self): self.input_sequences = [] for line in data: token_list = self.tokenizer.texts_to_sequences([line])[0] for i in range(1, len(token_list)): n_gram_sequence = token_list[:i + 1] self.input_sequences.append(n_gram_sequence) self.max_sequence_length = max([len(x) for x in self.input_sequences]) self.input_sequences = pad_sequences(self.input_sequences, maxlen=self.max_sequence_length, padding='pre') def create_model(self): self.model = Sequential() self.model.add(Embedding(self.total_words, 100, input_length=self.max_sequence_length - 1)) self.model.add(LSTM(150, return_sequences=True)) self.model.add(Dropout(0.2)) self.model.add(LSTM(100)) self.model.add(Dense(self.total_words, activation='softmax')) def train(self): self.model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) X, y = self.input_sequences[:, :-1], self.input_sequences[:, -1] y = tf.keras.utils.to_categorical(y, num_classes=self.total_words) self.model.fit(X, y, epochs=200, verbose=1)
IV - Test
Finally, I test the model, with a test method called in the constructor of my classes.
Warning: I block generation in this test function if the word generated is identical to the previous one.
def test(self, sentence: str, nb_word_to_generate: int): last_word = "" for _ in range(nb_word_to_generate): token_list = self.tokenizer.texts_to_sequences([sentence])[0] token_list = pad_sequences([token_list], maxlen=self.max_sequence_length - 1, padding='pre') predicted = np.argmax(self.model.predict(token_list), axis=-1) output_word = "" for word, index in self.tokenizer.word_index.items(): if index == predicted: output_word = word break if last_word == output_word: return sentence sentence += " " + output_word last_word = output_word return sentence
The above is the detailed content of Creating an LLM for testing with tensorflow in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










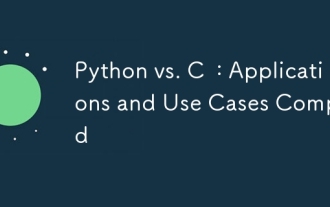
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
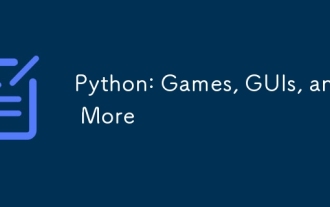
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
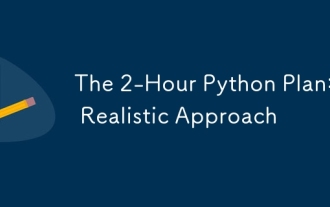
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
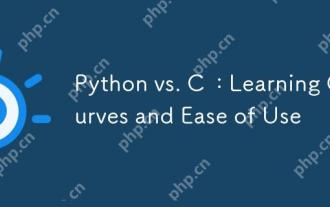
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
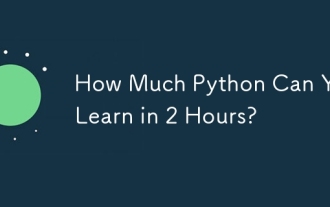
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
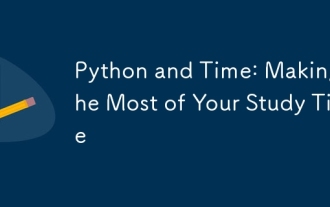
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
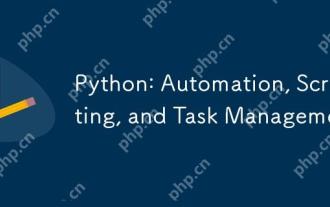
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
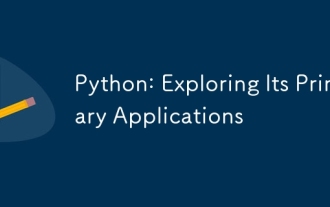
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
