PHP Forms: Handling User Input Like a Pro
Key steps for handling form input in PHP include: Validating input to prevent malicious code. Check for errors to ensure users fill out all fields correctly. Store data securely to prevent unauthorized access. By using filters and prepared statements, you can create secure PHP forms that handle user input.
PHP Forms: Handling User Input Professionally
Introduction
PHP Forms are An important tool for interacting with users and collecting information. When handling user input, it's crucial to ensure it's valid and safe. This article will guide you step-by-step through processing form input in PHP, including practical examples.
Step 1: Validate input
Use PHP built-in functions such as filter_input
to filter and sanitize user input.
$name = filter_input(INPUT_POST, 'name', FILTER_SANITIZE_STRING); $email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL);
Step 2: Check for Errors
Use filter_has_var
and filter_input_array
to check for any typing errors.
if (filter_has_var(INPUT_POST, 'name') && filter_input_array(INPUT_POST, 'name', FILTER_VALIDATE_STRING)) { // 表单已正确提交 } else { // 显示错误消息 }
Step 3: Store data securely
Store user input in a database or other secure location. Use prepared statements to prevent SQL injection attacks.
$stmt = $db->prepare("INSERT INTO users (name, email) VALUES (?, ?)"); $stmt->bind_param("ss", $name, $email); $stmt->execute();
Practical Case: Contact Form
Create a PHP script to handle contact form submission:
<?php // 验证输入 $name = filter_input(INPUT_POST, 'name', FILTER_SANITIZE_STRING); $email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL); $message = filter_input(INPUT_POST, 'message', FILTER_SANITIZE_STRING); // 检查错误 if (filter_has_var(INPUT_POST, 'name') && filter_input_array(INPUT_POST, 'message', FILTER_VALIDATE_STRING)) { // 存储数据 $stmt = $db->prepare("INSERT INTO messages (name, email, message) VALUES (?, ?, ?)"); $stmt->bind_param("sss", $name, $email, $message); $stmt->execute(); // 显示成功消息 echo "消息已发送。谢谢您的联系。"; } else { // 显示错误消息 echo "请填写所有必需的字段。"; } ?>
The above is the detailed content of PHP Forms: Handling User Input Like a Pro. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










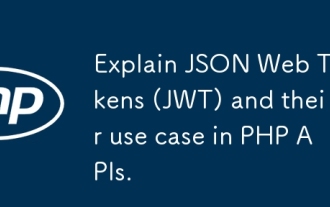
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
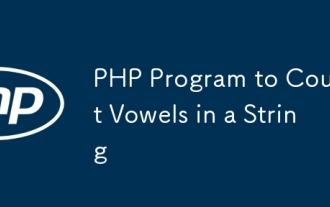
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
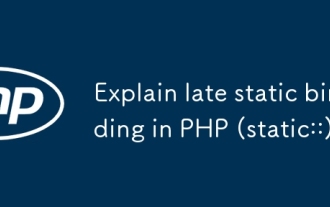
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
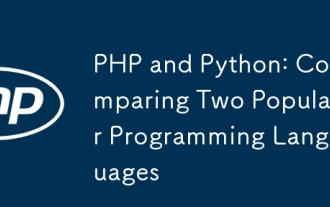
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
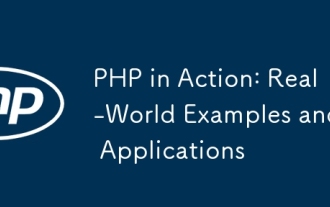
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
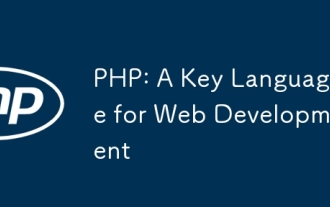
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
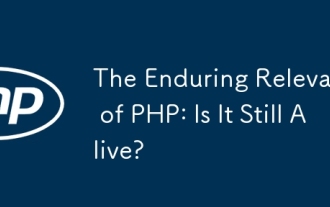
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
