Build a Dynamic Content Management System with PHP
You can use PHP to build a dynamic content management system (CMS) that allows users to manage website content. The steps include: 1. Create a database 2. Connect to MySQL 3. Create a controller for creating articles 4. Create a controller for reading articles 5. Create a controller for updating articles 6. Create a controller for deleting articles Controller 7. Configure routing 8. Create views 9. Once completed, the CMS is ready to use.
Building a dynamic content management system using PHP
Introduction
A dynamic content management system (CMS) allows users to manage and maintain content on a website Content, no technical knowledge required. This article will guide you through building a simple CMS using PHP.
Practical Case
We will create a simple blog CMS that allows users to create, edit and delete blog posts.
Steps
1. Create database
CREATE TABLE articles ( id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255) NOT NULL, content TEXT NOT NULL, created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP );
2. Connect to MySQL
$servername = "localhost"; $username = "root"; $password = ""; $dbname = "my_cms"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); }
3. Create CreateController
class CreateController { public function store() { $title = $_POST['title']; $content = $_POST['content']; $sql = "INSERT INTO articles (title, content) VALUES (?, ?)"; $stmt = $this->conn->prepare($sql); $stmt->bind_param("ss", $title, $content); $stmt->execute(); header("Location: /"); exit; } }
4. Create ReadController
class ReadController { public function index() { $sql = "SELECT * FROM articles ORDER BY created_at DESC"; $stmt = $this->conn->prepare($sql); $stmt->execute(); $articles = $stmt->get_result()->fetch_all(MYSQLI_ASSOC); return view('articles/index', ['articles' => $articles]); } }
5. Create UpdateController
class UpdateController { public function edit($id) { $sql = "SELECT * FROM articles WHERE id = ?"; $stmt = $this->conn->prepare($sql); $stmt->bind_param("i", $id); $stmt->execute(); $article = $stmt->get_result()->fetch_assoc(); return view('articles/edit', ['article' => $article]); } public function update($id) { $title = $_POST['title']; $content = $_POST['content']; $sql = "UPDATE articles SET title = ?, content = ? WHERE id = ?"; $stmt = $this->conn->prepare($sql); $stmt->bind_param("ssi", $title, $content, $id); $stmt->execute(); header("Location: /"); exit; } }
6. Create DeleteController
class DeleteController { public function destroy($id) { $sql = "DELETE FROM articles WHERE id = ?"; $stmt = $this->conn->prepare($sql); $stmt->bind_param("i", $id); $stmt->execute(); header("Location: /"); exit; } }
7. Create route
Use your favorite routing system configuration routing.
8. Create Views
Create the view file using your favorite template engine.
9. Complete
Once you complete these steps, your CMS is ready!
The above is the detailed content of Build a Dynamic Content Management System with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










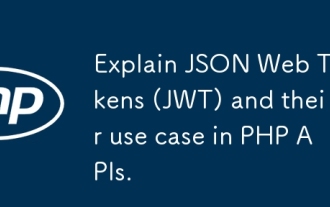
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
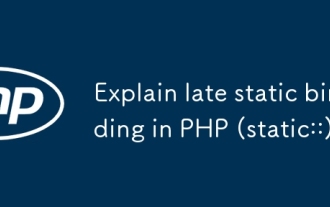
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
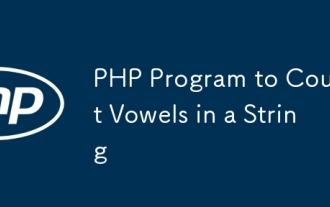
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
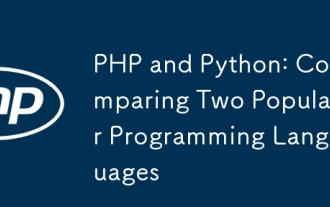
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
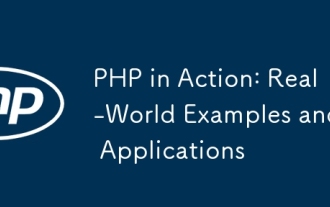
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
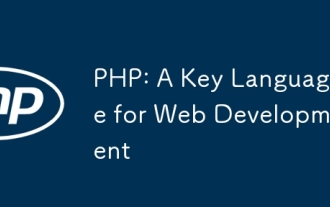
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
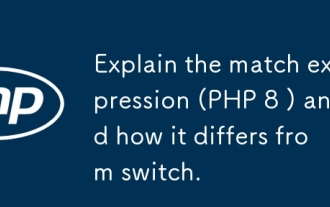
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
