How to Pass Class Field to Class Method Decorator
Passing Class Field to Class Method Decorator
When attempting to pass a class field to a decorator on a class method, you may encounter an error stating that the field does not exist. This arises because you are attempting to pass the field at the time of class definition, but it may not be available at that stage.
Solution 1: Runtime Check
To resolve this, consider checking the field at runtime instead. This can be achieved by modifying the decorator to intercept the method arguments, where the first argument will be the instance. The instance attribute can then be accessed using .:
<code class="python">def check_authorization(f): def wrapper(*args): print(args[0].url) return f(*args) return wrapper class Client(object): def __init__(self, url): self.url = url @check_authorization def get(self): print('get') Client('http://www.google.com').get()</code>
Solution 2: Attribute Name as String
If you wish to avoid hardcoding the attribute name in the decorator, you can pass it as a string:
<code class="python">def check_authorization(attribute): def _check_authorization(f): def wrapper(self, *args): print(getattr(self, attribute)) return f(self, *args) return wrapper return _check_authorization</code>
The above is the detailed content of How to Pass Class Field to Class Method Decorator. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


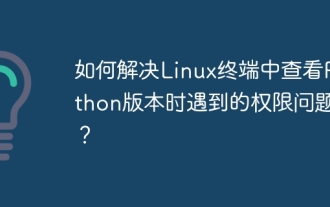
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
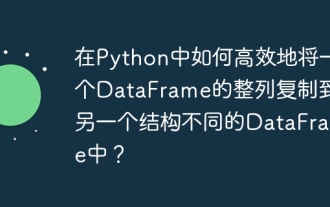
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
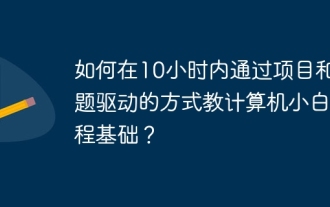
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
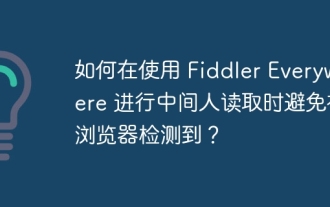
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
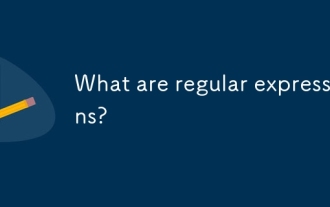
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
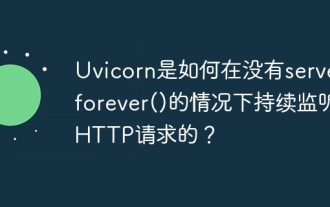
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
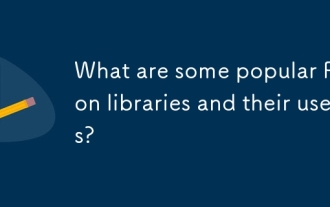
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
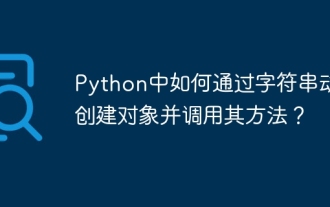
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
