


How to Effectively Read Files Recursively in Python Folders
Python: Tackling Recursive Folder Reading
As a beginner venturing into Python, one may encounter the challenge of recursively reading text files within a folder hierarchy. A code snippet circulating online addresses this issue, but its limitation lies in its inability to delve beyond a single folder. This guide delves deeper into Python's os.walk function and introduces os.path.join to effectively traverse and output the contents of a folder structure recursively.
Understanding os.walk
The core mechanism employed in the aforementioned code is Python's os.walk function. It returns three distinct values: root, subdirs, and files. root represents the current directory being inspected, subdirs lists subdirectories within root, and files enumerates non-directory files present in root.
Addressing the Loop Issue
The original code's loop iterations falter when attempting to traverse multiple levels of folders. To remedy this, it should be modified to loop through subdirectories and their respective files within the current root directory.
Applying os.path.join
This issue arises from an improper concatenation of file paths. os.path.join ensures accurate path manipulation by joining the current root with the file name.
Enhanced Code
The refined Python script incorporates these enhancements:
<code class="python">import os import sys walk_dir = sys.argv[1] for root, subdirs, files in os.walk(walk_dir): list_file_path = os.path.join(root, 'my-directory-list.txt') with open(list_file_path, 'wb') as list_file: for subdir in subdirs: list_file.write(('Subdirectory: {}\n'.format(subdir)).encode('utf-8')) for filename in files: file_path = os.path.join(root, filename) list_file.write(('File: {} (full path: {})\n'.format(filename, file_path)).encode('utf-8'))</code>
Utilizing the with Statement
The with statement offers a convenient and efficient way of handling file operations and ensures proper resource management. It streamlines the process, ensuring that files are automatically closed upon completion.
Conclusion
This extended guide elaborates on the initial problem, providing a comprehensive understanding of os.walk and os.path.join. The enhanced code embraces these concepts for efficient and accurate recursive folder reading, empowering Python users to effectively traverse complex directory structures.
The above is the detailed content of How to Effectively Read Files Recursively in Python Folders. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


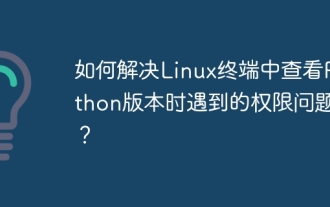
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
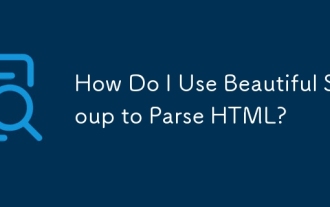
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
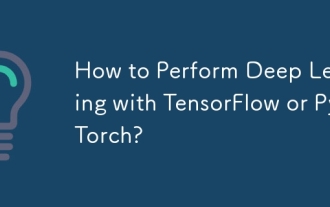
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
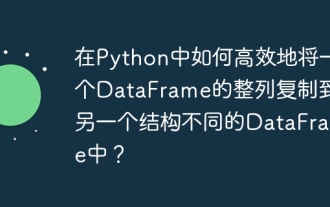
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
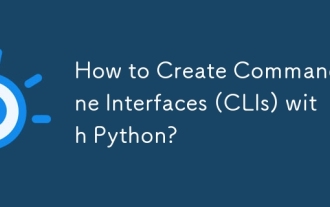
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
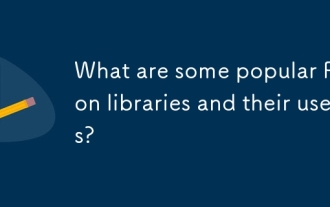
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
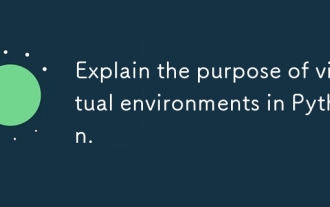
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.
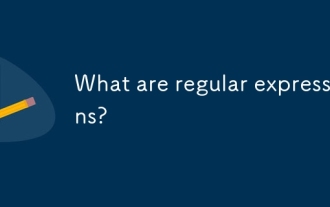
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
