Can You Use Async Await Directly Within a React Render Function?
Oct 18, 2024 pm 03:32 PMAsync Await in React Render Function
Problem:
You want to use the async await feature in the render function of a React component to perform asynchronous operations, such as fetching data from a backend API. However, you encounter issues with the await keyword not being recognized within the render function.
Solution:
While you can use the async keyword in a class component's render method, it's not recommended due to performance considerations. Instead, it's better to separate data fetching and display into two distinct components:
Parent Component (Data Fetching):
<code class="javascript">class ParentComponent extends React.Component { constructor() { super(); this.state = { data: null }; } componentDidMount() { fetch('/api/data') .then(res => res.json()) .then(data => this.setState({ data })); } render() { return this.state.data ? <ChildComponent data={this.state.data} /> : null; } }</code>
Child Component (Data Display):
<code class="javascript">const ChildComponent = ({ data }) => { return ( <table> <tbody> {data.map((item, index) => ( <tr key={index}> <td>{item.name}</td> </tr> ))} </tbody> </table> ); };</code>
Explanation:
- The parent component ParentComponent handles fetching the data from the API using the fetch function.
- Once the data is received, it sets the data state, which triggers a re-render.
- In the render method, the parent component checks if the data property in the state exists. If it does, it renders the child component ChildComponent, passing it the fetched data as props.
- ChildComponent is a pure functional component that displays the data in a table.
Note:
For React hooks-based components, you can use the useEffect hook to fetch data and update the component state, separating the data fetching logic from the render function.
The above is the detailed content of Can You Use Async Await Directly Within a React Render Function?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
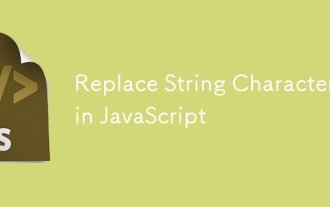
Replace String Characters in JavaScript
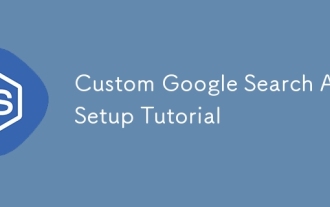
Custom Google Search API Setup Tutorial
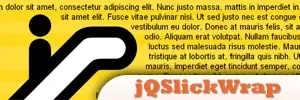
8 Stunning jQuery Page Layout Plugins
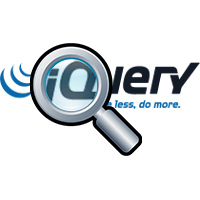
Improve Your jQuery Knowledge with the Source Viewer
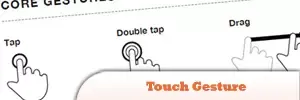
10 Mobile Cheat Sheets for Mobile Development
