Write React Components Like a Pro: A Beginner's Blueprint
Write a React Component Like a Pro: A Beginner's Guide from Zero to Hero
Introduction
React is one of the most popular JavaScript libraries for building user interfaces. Whether you are a beginner or a seasoned developer, mastering the art of creating and enhancing components is key to building scalable applications. In this guide, we will cover everything you need to know about writing React components, enhancing them with Higher-Order Components (HOCs), using hooks for fetching data, and controlling side effects. By the end, you'll be equipped to build and structure React applications like a pro.
What is a React Component?
A React component is essentially a JavaScript function or class that outputs UI elements using JSX (a syntax extension of JavaScript). Components allow you to split the UI into independent, reusable pieces.
Here’s an example of a simple functional component:
import React from 'react'; function WelcomeMessage() { return <h1>Welcome to React</h1>; } export default WelcomeMessage;
Why Components Matter: Components are the building blocks of any React application. They help in breaking down complex UIs into smaller, manageable parts.
Enhancing Components with Higher-Order Components (HOCs)
What are HOCs?
A Higher-Order Component is a function that takes a component and returns a new enhanced component. HOCs are used to reuse logic across multiple components.
Here’s an example of an HOC that adds logging functionality:
function withLogging(WrappedComponent) { return function EnhancedComponent(props) { console.log('Component is rendering with props:', props); return <WrappedComponent {...props} />; }; } // Usage const WelcomeWithLogging = withLogging(WelcomeMessage);
In this example, the withLogging HOC wraps the WelcomeMessage component and logs the props each time the component is rendered. This allows you to enhance existing components with additional functionality, like logging, authentication checks, etc., without modifying the original component.
Fetching Data with Hooks
React's built-in hooks allow you to add state and lifecycle features to functional components. One of the most important hooks for fetching data is useEffect.
Fetching Data Example:
import React, { useState, useEffect } from 'react'; function DataFetcher() { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/posts') .then((response) => response.json()) .then((data) => { setData(data); setLoading(false); }); }, []); if (loading) { return <div>Loading...</div>; } return ( <ul> {data.map((item) => ( <li key={item.id}>{item.title}</li> ))} </ul> ); } export default DataFetcher;
Here’s what happens:
- useState manages the loading state and fetched data.
- useEffect is used to fetch data when the component is first rendered.
- The component conditionally renders a loading state or the fetched data.
Why Hooks Matter: Hooks like useState and useEffect make managing state and side effects (like fetching data) much easier, especially in functional components.
Controlling Side Effects with useEffect
React's useEffect hook is crucial for handling side effects like API calls, timers, or manual DOM manipulations.
Effects Without Cleanup:
Not all effects require cleanup. For example, fetching data when a component mounts doesn't require cleanup. You can use useEffect for these types of operations.
import React from 'react'; function WelcomeMessage() { return <h1>Welcome to React</h1>; } export default WelcomeMessage;
The empty array ([]) ensures this effect runs only once, mimicking the behavior of componentDidMount.
Effects With Cleanup:
Certain side effects like setting up a subscription or a timer require cleanup when the component is unmounted to avoid memory leaks.
Example:
function withLogging(WrappedComponent) { return function EnhancedComponent(props) { console.log('Component is rendering with props:', props); return <WrappedComponent {...props} />; }; } // Usage const WelcomeWithLogging = withLogging(WelcomeMessage);
In this example, we set up a timer inside useEffect and return a cleanup function to clear the interval when the component is unmounted.
Why Cleanup is Important: Without cleanup, effects like subscriptions or intervals could continue running even after the component is no longer present, leading to performance issues.
Assembling the App
Now that we have seen the building blocks, let’s assemble a small app that fetches data, logs component renderings, and displays the data.
import React, { useState, useEffect } from 'react'; function DataFetcher() { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/posts') .then((response) => response.json()) .then((data) => { setData(data); setLoading(false); }); }, []); if (loading) { return <div>Loading...</div>; } return ( <ul> {data.map((item) => ( <li key={item.id}>{item.title}</li> ))} </ul> ); } export default DataFetcher;
In this example:
- DataFetcher fetches data from an API and displays it.
- The withLogging HOC wraps DataFetcher to log its render cycle.
- The App component assembles everything, displaying a header and the data fetched by DataFetcher.
FAQs
What is JSX?
JSX is a syntax extension for JavaScript that looks similar to HTML. It allows you to write UI elements inside JavaScript.
Why use useEffect?
useEffect allows you to handle side effects like fetching data or manipulating the DOM in a functional component.
What are Higher-Order Components (HOCs)?
HOCs are functions that take a component and return an enhanced component, allowing you to reuse component logic.
Conclusion
In this guide, we have covered everything you need to go from zero to hero in writing React components. From understanding basic components to enhancing them with Higher-Order Components and managing side effects with useEffect, you now have a strong foundation to build scalable and reusable components. With practice, you'll master these concepts and be able to handle more advanced React applications. Keep coding!
The above is the detailed content of Write React Components Like a Pro: A Beginner's Blueprint. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










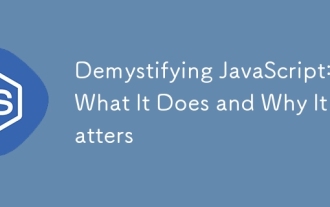
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
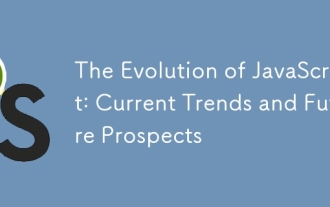
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
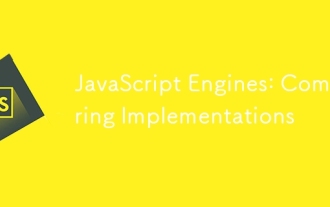
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
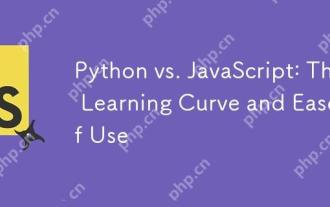
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
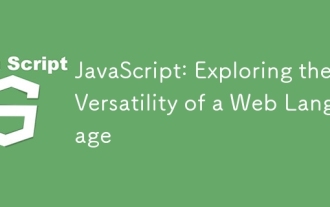
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
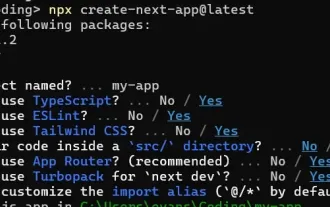
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
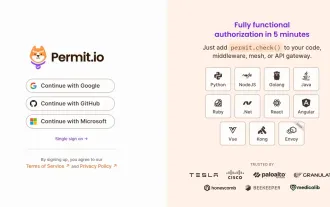
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
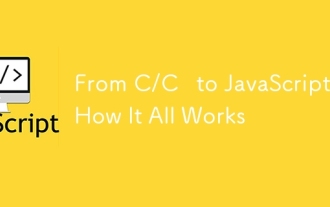
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
